Question
In JAVA Add the hashCode method to the FamousPerson class. @Override public int hashCode() // Returns a hash code value for this FamousPerson object. {
In JAVA
Add the hashCode method to the FamousPerson class.
@Override public int hashCode() // Returns a hash code value for this FamousPerson object. { return Math.abs((lastName.hashCode() * 3) + firstName.hashCode()); }
Write an application that reads the input/FamousCS.txt file, creates a FamousPerson object for each line of the file, and generates and saves its associated hashCode() value in an array.
Output the values from the array in sorted order.
Replace each array value A with the value A % 1,000, and again output the values in the array in sorted order.
Replace each array value A with the value A % 100, and again output the values in the array in sorted order.
Replace each array value A with the value A % 10, and again output the values in the array in sorted order.
Write a report about your observations on the output.
@Override public boolean equals(Object obj) // Returns true if 'obj' is a FamousPerson with same first and last // names as this FamousPerson, otherwise returns false. { if (obj == this) return true; else if (obj == null || obj.getClass() != this.getClass()) return false; else { FamousPerson fp = (FamousPerson) obj; return (this.firstName.equals(fp.firstName) && this.lastName.equals(fp.lastName)); } }
//--------------------------------------------------------------------------- // CSInfo.java by Dale/Joyce/Weems Chapter 5 // // Reads information about famous computer scientists from the file // FamousCS.txt. Allows user to enter names and provides them the information // from the file that matches the name. //--------------------------------------------------------------------------- package ch05.apps; import java.io.*; import java.util.*; import ch05.collections.*; import support.FamousPerson; public class CSInfo { public static void main(String[] args) throws IOException { // instantiate collection final int CAPACITY = 300; ArrayCollectionpeople = new ArrayCollection (CAPACITY); // set up file reading FileReader fin = new FileReader("input/FamousCS.txt"); Scanner info = new Scanner(fin); info.useDelimiter("[,\ ]"); // delimiters are commas, line feeds Scanner scan = new Scanner(System.in); FamousPerson person; String fname, lname, fact; int year; // read the info from the file and put in collection while (info.hasNext()) { fname = info.next(); lname = info.next(); year = info.nextInt(); fact = info.next(); person = new FamousPerson(fname, lname, year, fact); people.add(person); } // interact with user, getting names and displaying info final String STOP = "X"; System.out.println("Enter names of computer scientists."); System.out.println("Enter: firstname lastname (" + STOP + " to exit) "); fname = null; lname = null; while (!STOP.equals(fname)) { System.out.print("Name> "); fname = scan.next(); if (!STOP.equals(fname)) { lname = scan.next(); person = new FamousPerson(fname, lname, 0, ""); if (people.contains(person)) { person = people.get(person); System.out.println(person); } else System.out.println("No information available "); } } } }
public int compareTo(FamousPerson other) // Precondition: 'other' is not null // // Compares this FamousPerson with 'other' for order. Returns a // negative integer, zero, or a positive integer as this object // is less than, equal to, or greater than 'other'. { if (!this.lastName.equals(other.lastName)) return this.lastName.compareTo(other.lastName); else return this.firstName.compareTo(other.firstName); }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
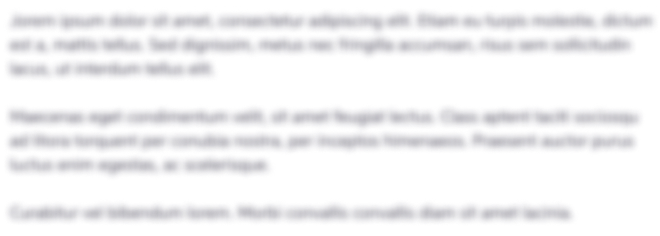
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started