Question
In Java: Based on the Account Class, provided below, which represents a bank account do the following: Inspect the functionality of the Account Class. We
In Java:
- Based on the Account Class, provided below, which represents a bank account do the following:
Inspect the functionality of the Account Class. We want to create two subclasses that represent different types of accounts. These classes would inherit and use functionalities from the Account class, and override and add their own specialized functionalities.
- Create two new classes called SavingsAccount and CheckingAccount, which should be subclasses of the Account class.
- Create a constructor in the SavingsAccount class that uses the superclass constructor. Assume when customers open a SavingsAccount, they receive a bonus of 10 dollars deposited to their account.
- Add a private instance variable to the CheckingAccount class that indicates how many signatories are on the account. Add a getter and setter for it.
- Create a constructor in the CheckingAccount class that also uses the superclass constructor, but also accepts and initializes the number of signatories.
- Override the withdraw() method in the CheckingAccount class to ensure a minimum balance is maintained in the account. Store the minimum balance required as a static variable of type BigDecimal. If the withdrawal is too large, inform the user by printing a statement, otherwise use the withdraw() method from the Account class.
- Override the toString() method from the Object class to print information about Account instances in the Account class. Override the Account class toString() method to specialize it for the subclasses in SavingsAccount and CheckingAccount.
- Why is the setScale method, and BigDecimal.ROUND_HALF_UP, striked out in the Account class? And what programming construct is used to make this effect?
Write the answer as a comment on top of the method call.
- Replace the setScale call with another valid call that would achieve a similar functionality.
- Use the AccountManager Class provided below to test your code, as a driver or client class.
Sample valid output:
Account Created: Save001 Deposit: Savings Account Save001: Balance = 20.00 Withdrawal: Savings Account Save001: Balance = 15.00 Account Created: Check002 withdrawal of amount: 5.00 would put the balance below the minimum of: 100 Deposit: Checking Account Check002: Balance = 510.00 Withdrawal: Checking Account Check002: Balance = 505.00
|
public class Account { private String name; private BigDecimal amount;
public Account(String acctName, String startAmount) { this.setName(acctName); this.setAmount(startAmount); this.amount.setScale(2, BigDecimal.ROUND_HALF_UP); System.out.println("Account Created: " + this.getName()); }
public String getName() { return this.name; }
public BigDecimal getAmount() { return this.amount; }
public void setName(String newName) { String pattern = "^[a-zA-Z0-9]*$"; if (newName.matches(pattern)) { this.name = newName; } }
private void setAmount(String newAmount){ this.amount = new BigDecimal(newAmount); }
public void withdraw(String withdrawal) { BigDecimal desiredAmount = new BigDecimal(withdrawal);
//if desired amount is negative, inform the user if (desiredAmount.compareTo(BigDecimal.ZERO) < 0){ System.out.println("The desired amount is negative."); }
//if the amount is less than the desired amount, inform the user if (this.getAmount().compareTo(desiredAmount) < 0){ System.out.println("The amount is less than the desired amount."); }
this.setAmount(this.getAmount().subtract(desiredAmount).toString()); System.out.println("Withdrawal: " + this); }
public void deposit(String deposit) { BigDecimal desiredAmount = new BigDecimal(deposit);
//if desired amount is negative, inform the user if (desiredAmount.compareTo(BigDecimal.ZERO) < 0){ System.out.println("The desired amount is negative."); }
this.setAmount(this.getAmount().add(desiredAmount).toString()); System.out.println("Deposit: " + this); }
@Override public String toString(){ return this.getName() + ": Balance = " + this.getAmount(); } } |
public class AccountManager {
public static void main(String[] args) { Account mySavings = new SavingsAccount("Save001", "10.00");
mySavings.withdraw("5.00");
Account myChecking = new CheckingAccount("Check002", "10.00", 1);
myChecking.withdraw("5.00");
myChecking.deposit("500.00");
myChecking.withdraw("5.00"); } }
|
Step by Step Solution
There are 3 Steps involved in it
Step: 1
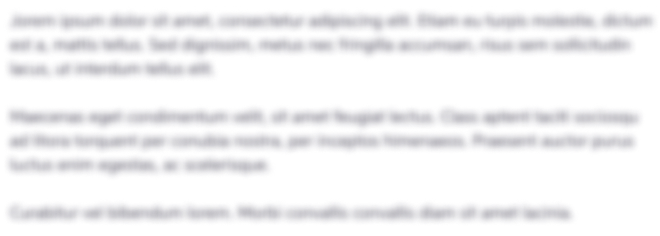
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started