Question
In Java, implement a class to recursively calculate the n-th line of Pascal's Triangle: 1: 1 2: 1 1 3: 1 2 1 4: 1
In Java, implement a class to recursively calculate the n-th line of Pascal's Triangle:
1: 1
2: 1 1
3: 1 2 1
4: 1 3 3 1
5: 1 4 6 4 1
6: 1 5 10 10 5 1
7: 1 6 15 20 15 6 1
8: 1 7 21 35 35 21 7 1
9: 1 8 28 56 70 56 28 8 1
10: 1 9 36 84 126 126 84 36 9 1
Important questions to ask yourself:
What is the base case?
What is the recursive case?
What should the method return?
Your class should be called Pascal and have one static method called triangle that takes an argument 'n' and returns the n-th line of Pascal's Triangle. If you can please explain each part of the java code in detail, I would appreciate it. The code for the main method should not be changed. The java code needs to be able to produce the correct output for this project.
Here is the code for the main method that will call the method you write.
public class Main {
public static void main(String[] args) {
int n = args.length == 1 ? Integer.parseInt(args[0]) : 1;
for (int i = 1; i <= n; ++i) {
int[] arr = Pascal.triangle(i);
System.out.print((i < 10 ? " " : "") + i + ": ");
for (int j : arr) {
System.out.print(j + " ");
}
System.out.println();
}
}
}
class Pascal {
public static int[] triangle(int n) {
return new int[]{0};
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
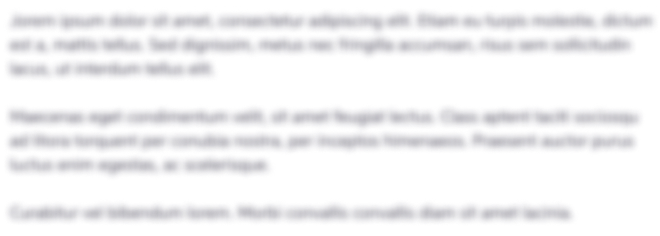
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started