Question
In Java, implement a hierarchy of classes: Book, Novel, TextBook, and UsedNovel. A book is specified by its title and base price. It contains methods
In Java, implement a hierarchy of classes: Book, Novel, TextBook, and UsedNovel. A book is specified by its title and base price. It contains methods that allow users to get the values of these variables as well as altering its base price. A Novel is a type of book that contains two additional information units: its type which could be romance, mystery, or adventure; and the percent markup on its base price. The actual cost of a Novel is its base price plus the percentage markup. For example, if the base price of a Novel was $20.00 and its markup percentage is 10%, then the actual cost of that Novel is $22.00. A Novel has methods that allow users to get the values of its title, type, base price, and markup percentage; calculate and return its actual cost; and change the markup percentage as desired. A TextBook is a type of book that identifies the field it belongs to, like CompSc, Physics, Economics, and so on. Additionally, invariably, TextBooks have percentage discount associated with them. The actual cost of a TextBook is its base price minus the discount. For example, if the base price of a TextBook was $50.00 and percent discount was 20%, then its actual cost would be $40.00. A TextBook provides methods that allow users to get the values of its title, field it belongs to, base price, and discount percentage; calculate and return its actual cost; and change the discount percentage as desired. A UsedNovel is a type of Novel that also indicates how old is that copy. If the copy is less than 5 years old, discount given to the customer is 5% of its actual cost, if it is more than or equal to 5 years but less than 10 years old, then the discount is 10%, and if it is greater or equal to 10 years old, the discount is 15%. The discount is applied after the price of the corresponding Novel is calculated. This class must provide methods to allow users to get the values of all instance variables declared or inherited, and return its cost price calculated as above. By the way, to create the individual objects of each type, the user must specify the values of all required parameters; no default values are assumed. It is mandatory that all these classes contain methods "toString" and "equals" that override these methods of Object class. Every class must be able to print out the values of all declared or inherited variables for its objects using the "toString" method. Two books are equal if they have the same title; two Novels are equal if they are the same book and are of the same type; two TextBooks are equal if they are the same book and belong to the same field; and two UsedNovels are equal if they are the same Novel and their actual costs are within a dollar of each other. To test your implementation of this hierarchy of classes, write a test program as follows: Prompt the user for the names of the input and output files. The first data in the input file is the number of Books to be created. Create Books in an ArrayList of Books by reading the information from the input file. For each Book, the first input item identifies what type of Book it is, and then the relevant information for that type of Book. Output all relevant information for each in the output file along with the actual cost. Make your output completely self-explanatory. You must use the "toString" method of each object's class (implicitly or explicitly) to output the values of all declared and inherited variables. Having created all Books, perform the specified transactions in the input file. After performing the transaction, output what was done and the result of that transaction. Each transaction is identified by a single Character as specified below: E Equality of two Books [E 1 2 means are Books at indices 1 and 2 of the ArrayList Equal?] M Change the markup of the Book to new value [M 1 20 means Change the markup of the Book at index 1 of the ArrayList to 20%.] D Change the discount of the Book to a new value [D 3 8 means Change the discount of the Book at index 3 of the ArrayList to 8%.] C Output the cost of the Book [C 5 means Output the Cost of the Book at index 5 of the ArrayList.] O Output the Book [O 6 means Output the details of Book at index 6 of the ArrayList.
Use the following code to scan user input
import java.io.FileReader; import java.io.PrintWriter; import java.util.Scanner; import javax.swing.JOptionPane; public class fileIO { public static void main (String[] args) throws Exception { String inf = JOptionPane.showInputDialog("Input file?"); // Input file is src/ FileReader inFile = new FileReader(inf); Scanner in = new Scanner(inFile); String outf = JOptionPane.showInputDialog("Output file?"); PrintWriter outFile = new PrintWriter(outf); while (in.hasNextLine()) { String line = in.nextLine(); outFile.println(line+" "); } outFile.close(); } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
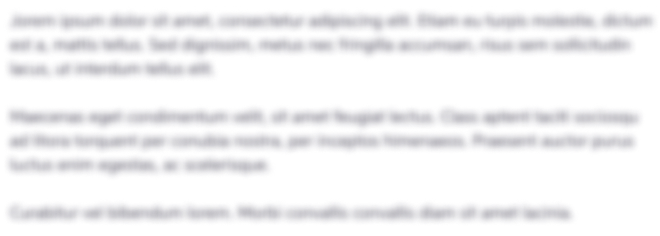
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started