Answered step by step
Verified Expert Solution
Question
1 Approved Answer
IN JAVA Instructions Your goal in this assignment is to implement LinkedList class invert method. As the name suggests, the invert method should reverse the
IN JAVA
Instructions
Your goal in this assignment is to implement LinkedList class invert method. As the name suggests, the invert method should reverse the order of elements in the original list. For example, if the original list is 1, 2, 3, after calling invert the list should read 3, 2, 1.
I WILL POST THE CODE FOR EACH CLASS BELOW
LinkedList.java
public class LinkedList { | |
private Node head; | |
public LinkedList() { | |
head = null; | |
} | |
// add in front | |
public void add(int data) { | |
Node newNode = new Node(data); | |
newNode.setNext(head); | |
head = newNode; | |
} | |
// add tail | |
public void append(int data) { | |
Node newNode = new Node(data); | |
if (isEmpty()) | |
head = newNode; | |
else { | |
Node current = head; | |
while (current.getNext() != null) | |
current = current.getNext(); | |
current.setNext(newNode); | |
} | |
} | |
@Override | |
public String toString() { | |
String out = ""; | |
Node current = head; | |
while (current != null) { | |
out += current.toString() + " "; | |
current = current.getNext(); | |
} | |
return out; | |
} | |
public boolean isEmpty() { | |
return head == null; | |
} | |
public int size() { | |
int count = 0; | |
Node current = head; | |
while (current != null) { | |
count++; | |
current = current.getNext(); | |
} | |
return count; | |
} | |
public int get(int index) { | |
if (index < 0 || index >= size()) | |
return 0; | |
int i = 0; | |
Node current = head; | |
while (i < index) { | |
i++; | |
current = current.getNext(); | |
} | |
return current.getData(); | |
} | |
public void set(int index, int data) { | |
if (index < 0 || index >= size()) | |
return; | |
int i = 0; | |
Node current = head; | |
while (i < index) { | |
i++; | |
current = current.getNext(); | |
} | |
current.setData(data); | |
} | |
void insert(int index, int data) { | |
if (index < 0 || index >= size()) | |
return; | |
if (index == 0) | |
add(data); | |
else { | |
Node newNode = new Node(data); | |
int i = 0; | |
Node current = head; | |
while (i < index - 1) { | |
i++; | |
current = current.getNext(); | |
} | |
newNode.setNext(current.getNext()); | |
current.setNext(newNode); | |
} | |
} | |
void remove(int index) { | |
if (index < 0 || index >= size()) | |
return; | |
if (index == 0) { | |
Node temp = head; | |
head = head.getNext(); | |
temp.setNext(null); | |
} | |
else { | |
int i = 0; | |
Node current = head; | |
while (i < index - 1) { | |
i++; | |
current = current.getNext(); | |
} | |
Node temp = current.getNext(); | |
current.setNext(current.getNext().getNext()); | |
temp.setNext(null); | |
} | |
} | |
} |
LinkedListDriver.java
import com.sun.security.jgss.GSSUtil; | |
public class LinkedListDriver { | |
public static void main(String[] args) { | |
LinkedList ll = new LinkedList(); | |
ll.append(3); | |
// ll.append(5); | |
// ll.append(8); | |
// ll.append(2); | |
System.out.println(ll); | |
ll.remove(0); | |
System.out.println(ll); | |
System.out.println(ll.isEmpty()); | |
} | |
} |
LinkedListTest.java
import org.junit.jupiter.api.Test; | |
import javax.sound.sampled.Line; | |
import static org.junit.jupiter.api.Assertions.*; | |
class LinkedListTest { | |
@Test | |
public void testListEmpty() { | |
LinkedList ll = new LinkedList(); | |
assertTrue(ll.isEmpty()); | |
assertEquals(0, ll.size()); | |
} | |
@Test | |
public void testListNotEmpty() { | |
LinkedList ll = new LinkedList(); | |
ll.add(5); | |
assertFalse(ll.isEmpty()); | |
assertNotEquals(0, ll.size()); | |
} | |
@Test | |
public void testListThreeFiveEight() { | |
// create the scenario 3, 5, 8 | |
LinkedList ll = new LinkedList(); | |
ll.append(3); | |
ll.append(5); | |
ll.append(8); | |
// do the assertions with isEmpty, size, get | |
assertFalse(ll.isEmpty()); | |
assertEquals(3, ll.size()); | |
assertEquals(3, ll.get(0)); | |
assertEquals(5, ll.get(1)); | |
assertEquals(8, ll.get(2)); | |
} | |
@Test | |
public void testListThreeFiveEightInsertTenBtwThreeFive() { | |
// create the scenario 3, 5, 8 | |
LinkedList ll = new LinkedList(); | |
ll.append(3); | |
ll.append(5); | |
ll.append(8); | |
ll.insert(1, 10); | |
// do the assertions with isEmpty, size, get | |
assertFalse(ll.isEmpty()); | |
assertEquals(4, ll.size()); | |
assertEquals(3, ll.get(0)); | |
assertEquals(10, ll.get(1)); | |
assertEquals(5, ll.get(2)); | |
assertEquals(8, ll.get(3)); | |
} | |
} |
NODE.java
public class Node { | |
private int data; | |
private Node next; | |
public Node() { | |
data = 0; | |
next = null; | |
} | |
public Node(int data) { | |
this.data = data; | |
next = null; | |
} | |
public int getData() { | |
return data; | |
} | |
public Node getNext() { | |
return next; | |
} | |
public void setData(int data) { | |
this.data = data; | |
} | |
public void setNext(Node next) { | |
this.next = next; | |
} | |
@Override | |
public String toString() { | |
return data + ""; | |
} | |
} |
Step by Step Solution
There are 3 Steps involved in it
Step: 1
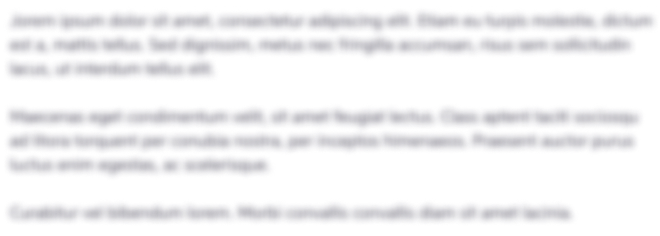
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started