Question
IN JAVA LANGUAGE PLEASE! Problem: Write a program to play the pig game against the computer. At each turn, the current player will roll a
IN JAVA LANGUAGE PLEASE!
Problem:
Write a program to play the pig game against the computer. At each turn, the current player will roll a pair of dice and accumulates points. The goal is to reach to 100 or more points before your opponent does. (For the testing purposes use 30 instead of 100 points) If, on any turn, the player rolls a 1, all the points accumulated for that round are forfeited and the control of the dice moves to the other player. If the player rolls two 1s in one turn, the player loses all the points accumulated thus far are forfeited and the control moves to the other player. The player may voluntarily turn over the control of the dice after each roll. Therefore player must decide to roll again (be a pig) and risk losing points, or relinquish control of the dice, possibly allowing the other player to win. Computer is going to flip a coin to choose the first player
Here is the list of the tasks that needs to be done
Describe the game by writing a method.
Data validation: A method that accepts a Scanner object as its parameter, prompt the user to
enter yes or no. as long as the user is not entering a valid input , prompt the user again
Flip the coin: This method accepts a Random object and returns head or tail based on the
random number that was generated
Roll two dices: this method accepts a Random object. Generates two random number
representing one of the numbers on a dice. Returns the sum of the dices.
Choose a name for the computer: Come up with 10 different name for the computer. Then
select a random name from the list that you created. Return the selected name.
Play: this method calls the other methods to play the game
Declare all the needed variables to keep track of the scores for each player, and Boolean variables to indicate who is playing at the moment.
Ask the users name
Decide who start the game first by calling one of the methods you created to flip the
coin.
Write conditional statements to switch the game between the computer and the player
based on the dice rolled and overall points. Read the output and the program description to figure out the conditions. You need to use couple while loops: one loop for the human player, one loop for the computer player. The previous two loops will be nested in another while loop to witch the game between the two players. //declaring your variables While (there is no winner) {
//some codes While (human is playing)
{
//some codes, conditional statements }
//you may need some codes While (computer is playing)
{ //some codes, conditional statements
} //you may need some codes }
e. Keep playing the game until the player or the computer has 100 or more points (40 or more for the simplicity). You are not allowed to hard code any numbers.
7. Main method: Calls the method play, keep playing the game as long as there are more players.
SAMPLE OUTPUT:
*********************************************************************************** * You are about to play the pig game against the computer. * * On each turn, the current player will roll a pair of dice * * and acumulates points. The goal is to reach to40 points * * before your opponent does. If, on any turn, the player roll * * all the points accumulated for that round are forfeited and * * the control of the dice moves to the other player. If the player * * rolls two 1s in one turn , the player loses all the points * * accumulated thus far and forfeit and the control moves to the * * other player. The player may voluntarily turn over the dice after * * each roll. Therefore player must decide to roll again(be a pig) * * and risk losing points , or relinquish control of the dice, possibly * * allowing the other player to win. * * Computer is going to flip a coin to choose the first player * *********************************************************************************** lets start the fun Hi my name is Ginger What is your name? Mary Hi Mary, I am fliping the coin to determine who goes first press any key to start the game. Ginger is going to start the game Ginger's turn: Points : 0 dice1 : 5 dice2 : 6 points : 11 Press any key to continue Ginger's turn: Points : 11 dice1 : 2 dice2 : 1 Sorry Ginger you lost the points for this turn points : 11 Press any key to continue Mary's turn: Points : 0 dice1 : 4 dice2 : 1 Sorry Mary you lost the points for this turn points : 0 press any key to continue Ginger's turn: Points : 11 dice1 : 3 dice2 : 1 Sorry Ginger you lost the points for this turn points : 11 Press any key to continue Mary's turn: Points : 0 dice1 : 4 dice2 : 2 points : 6 press any key to continue Mary's turn: Points : 6 dice1 : 6 dice2 : 3 points : 15 press any key to continue Mary's turn: Points : 15 dice1 : 3 dice2 : 6 points : 24 Do you want to forfeit your turn since you have 20 or more points? no points : 24 press any key to continue Mary's turn: Points : 24 dice1 : 1 dice2 : 1 Sorry Mary you lost all your points points : 0 press any key to continue Ginger's turn: Points : 11 dice1 : 2 dice2 : 5 points : 18 Press any key to continue Ginger's turn: Points : 18 dice1 : 4 dice2 : 1 Sorry Ginger you lost the points for this turn points : 18 Press any key to continue Mary's turn: Points : 0 dice1 : 4 dice2 : 4 points : 8 press any key to continue Mary's turn: Points : 8 dice1 : 6 dice2 : 6 points : 20 Do you want to forfeit your turn since you have 20 or more points? no points : 20 press any key to continue Mary's turn: Points : 20 dice1 : 1 dice2 : 6 Sorry Mary you lost the points for this turn points : 20 press any key to continue Ginger's turn: Points : 18 dice1 : 4 dice2 : 2 points : 24 I am forfeiting my turn since I have 24 which is more than twenty points. Press any key to continue Mary's turn: Points : 20 dice1 : 6 dice2 : 1 Sorry Mary you lost the points for this turn points : 20 press any key to continue Ginger's turn: Points : 24 dice1 : 4 dice2 : 6 points : 34 I am forfeiting my turn since I have 34 which is more than twenty points. Press any key to continue Mary's turn: Points : 20 dice1 : 1 dice2 : 1 Sorry Mary you lost all your points points : 0 press any key to continue Ginger's turn: Points : 34 dice1 : 4 dice2 : 1 Sorry Ginger you lost the points for this turn points : 34 I am forfeiting my turn since I have 34 which is more than twenty points. Press any key to continue Mary's turn: Points : 0 dice1 : 6 dice2 : 1 Sorry Mary you lost the points for this turn points : 0 press any key to continue Ginger's turn: Points : 34 dice1 : 3 dice2 : 1 Sorry Ginger you lost the points for this turn points : 34 I am forfeiting my turn since I have 34 which is more than twenty points. Press any key to continue Mary's turn: Points : 0 dice1 : 6 dice2 : 1 Sorry Mary you lost the points for this turn points : 0 press any key to continue Ginger's turn: Points : 34 dice1 : 1 dice2 : 3 Sorry Ginger you lost the points for this turn points : 34 I am forfeiting my turn since I have 34 which is more than twenty points. Press any key to continue Mary's turn: Points : 0 dice1 : 1 dice2 : 6 Sorry Mary you lost the points for this turn points : 0 press any key to continue Ginger's turn: Points : 34 dice1 : 3 dice2 : 6 points : 43 Hurray !!!!!! You reached or passed 40 points Press any key to continue Hurray!!! We have a winner Somebody got 40 or more Ginger points: 43 Mary points: 0 Ginger won the game Is there another player? yes *********************************************************************************** * You are about to play the pig game against the computer. * * On each turn, the current player will roll a pair of dice * * and acumulates points. The goal is to reach to40 points * * before your opponent does. If, on any turn, the player roll * * all the points accumulated for that round are forfeited and * * the control of the dice moves to the other player. If the player * * rolls two 1s in one turn , the player loses all the points * * accumulated thus far and forfeit and the control moves to the * * other player. The player may voluntarily turn over the dice after * * each roll. Therefore player must decide to roll again(be a pig) * * and risk losing points , or relinquish control of the dice, possibly * * allowing the other player to win. * * Computer is going to flip a coin to choose the first player * *********************************************************************************** lets start the fun Hi my name is Sleepy What is your name? Alex Hi Alex, I am fliping the coin to determine who goes first press any key to start the game. Sleepy is going to start the game Sleepy's turn: Points : 0 dice1 : 4 dice2 : 5 points : 9 Press any key to continue Sleepy's turn: Points : 9 dice1 : 4 dice2 : 5 points : 18 Press any key to continue Sleepy's turn: Points : 18 dice1 : 5 dice2 : 5 points : 28 I am forfeiting my turn since I have 28 which is more than twenty points. Press any key to continue Alex's turn: Points : 0 dice1 : 5 dice2 : 4 points : 9 press any key to continue Alex's turn: Points : 9 dice1 : 5 dice2 : 6 points : 20 Do you want to forfeit your turn since you have 20 or more points? no points : 20 press any key to continue Alex's turn: Points : 20 dice1 : 3 dice2 : 2 points : 25 Do you want to forfeit your turn since you have 20 or more points? no points : 25 press any key to continue Alex's turn: Points : 25 dice1 : 5 dice2 : 3 points : 33 Do you want to forfeit your turn since you have 20 or more points? no points : 33 press any key to continue Alex's turn: Points : 33 dice1 : 6 dice2 : 3 Hurray !!!!!! You reached or passed 40 points points : 42 press any key to continue Hurray!!! We have a winner Somebody got 40 or more Sleepy points: 28 Alex points: 42 Alex won the game Is there another player? no
Step by Step Solution
There are 3 Steps involved in it
Step: 1
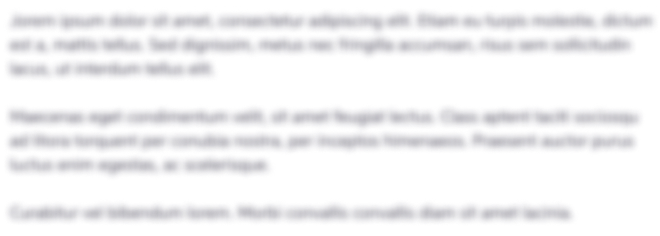
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started