Question
In Java. Modify the single server version of KnockKnock program so that the client and server take turns exchanging string messages (Simple Instant Messenger). SimpleIMProtocol
In Java. Modify the single server version of KnockKnock program so that the client and server take turns exchanging string messages (Simple Instant Messenger). SimpleIMProtocol replaces KnockKnockProtocol. Display a prompt to indicate whose turn it is to type a message.
KnockKnockProtocol:
import java.net.*; import java.io.*; public class KnockKnockProtocol { private static final int WAITING = 0; private static final int SENTKNOCKKNOCK = 1; private static final int SENTCLUE = 2; private static final int ANOTHER = 3; private static final int NUMJOKES = 5; private int state = WAITING; private int currentJoke = 0; private String[] clues = { "Turnip", "Young man", "CYT", "Little Old Lady", "Atch", "Who", "Who"}; private String[] answers = { "Turnip the heat, it's cold in here!", "Someone can help you!", "See you tomorrow!", "I didn't know you could yodel!", "Bless you!", "Is there an owl in here?", "Is there an echo in here?"}; /** * processInput: * @param theInput, a String, what the client last said * @return a String representing the server's intended reply */ public String processInput(String theInput) { String theOutput = null; if (state == WAITING) { theOutput = "Knock! Knock!"; state = SENTKNOCKKNOCK; } else if (state == SENTKNOCKKNOCK) { if (theInput.equalsIgnoreCase("Who's there?")) { theOutput = clues[currentJoke]; state = SENTCLUE; } else { theOutput = "You're supposed to say \"Who's there?\"! " + "Try again. Knock! Knock!"; } } else if (state == SENTCLUE) { if (theInput.equalsIgnoreCase(clues[currentJoke] + " who?")) { theOutput = answers[currentJoke] + " Want another? (y/n)"; state = ANOTHER; } else { theOutput = "You're supposed to say \"" + clues[currentJoke] + " who?\"" + "! Try again. Knock! Knock!"; state = SENTKNOCKKNOCK; } } else if (state == ANOTHER) { if (theInput.equalsIgnoreCase("y")) { theOutput = "Knock! Knock!"; if (currentJoke == (NUMJOKES - 1)) currentJoke = 0; else currentJoke++; state = SENTKNOCKKNOCK; } else { theOutput = "Bye."; state = WAITING; } } return theOutput; } }
Server:
import java.net.*; import java.io.*; public class KnockKnockServer { public static void main(String[] args) throws IOException { if (args.length != 1) { System.err.println("Usage: java SimpleIMProtocolServer
Client:
import java.io.*; import java.net.*; public class KnockKnockClient { public static void main(String[] args) throws IOException { if (args.length != 2) { System.err.println( "Usage: java EchoClient
Step by Step Solution
There are 3 Steps involved in it
Step: 1
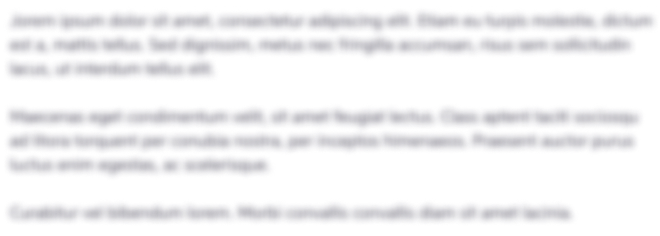
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started