Question
1 Overview of the Application In this assignment, you will develop your social media platform (what we will call it is mysocialbook) with very simplified
1 Overview of the Application
In this assignment, you will develop your social media platform (what we will call it is mysocialbook) with very simplified use case scenarios. To complete every functionality
of mysocialbook, please read each of the following lines with a high care.
2 Application's Specification
This and the following sections are dedicated to clearly review the requirements (or specification) of the system you are going to develop.The core components of the system will include:
• users (people having an account of mysocialbook),
• posts (textual or multimedia post shared by users).
You will be given two files separately: the one contains information necessary for creating the initial users for the system, while the other contains dierent commands to test various use case scenarios of your application. These components are further explained in detail in the following sections.
2.1 Users
Users refers to the people who only use mysocialbook platform (i.e., they do not have any privilege such as admin, system manager, and the like). The properties and behaviors that user can have are listed below:
• Every User in the system has a unique userID, a name, a unique username, a pass- word, a date of birth, information about school from which the user graduated,
a last log-in date, a collection of friends, a collection of blocked users, and a collection of posts.
• userIDs should be assigned as integers in order, starting from 1 for the first user that is added to the system. These IDs cannot be changed later. A newly added user
should never get an ID that has been assigned before to some other user, even in cases when other users get removed from the system.
• Users' dates of birth and last log-in dates should be stored as Date variables.
• Users must perform
( Sign in, Sign out, Update their user profile info (name, date of birth, and school graduated), Change their passwords, Add friends to their friend lists, Remove friends from their friend lists, Add posts, Remove posts, Block users, Unblock users, List their friends, List all users, List blocked friends, List blocked users.)
• It is a must for any user to be logged-in in order for her/him to perform any of these actions other than sign in.
• You are required to hide sensitive personal user info and implement setter and getter methods as necessary.
• mysocialbook platform should begin execution by reading a tab-separated file named input.txt. The user's data will be given as following format:
nameusernamepassworddateofBirthgraduatedSchool
An exemplar for the content of this file is shown below:
Ahmet ahmet ahmet 123 04/25/2001 Meram Anadolu Lisesi
Demet demet demet00 01/16/1999 Ankara Fen Lisesi
Zeki zeki zeki01 08/16/1987 Kadıkoy Lisesi
Gizem gizem gizem1 12/09/1997 Hacettepe Universitesi
Utku utku utku99 10/06/1999 Bilkent ¨Universitesi
Hakan hakan hakan81 03/01/1981 Mugla S.K. ¨Universitesi
2.2 Posts: They can either be a textual or multimedia content shared by users. You are expected to design your post classes in a hierarchical structure. The necessary information about posts is as follows:
• Posts are categorized as either text posts, image posts, or video posts. But be careful: these posts have a textual part in common, and can optionally contain image or
video (for image and video post categories, respectively!).
• mysocialbook should be designed such that it is open for an integration of any new post type (e.g., hypertext posts) that may or may not have a textual part.
• Every post category must contain a location, a collection of tagged friends, and a date when the post originated.
• Location should be implemented as a separate class with the following attributes: latitude and longitude (stored as double values).
• The format to display the post itself can change with respect to the post type of interest. So, in the case of inclusion a new post type to mysocialbook, designer must
specify its printing format. You should enforce user who proposes a new post type to implement display format of that post.
• Text posts have a text.
• Image posts have the image filename, and the image resolution (width and height in pixels).
• Video posts have the video filename, video duration, and a constant attribute that specifies the maximum video length in minutes (the maximum allowed video duration is 10 minutes).
• Note that you are required to implement setter and getter methods as necessary.
3 Testing Scenarios
i. Add New User: The system should also be open for adding new users. The program should add a if the syntax of the command is as provided below:
ADDUSERnameuserNamepassworddateofBirthschoolGraduated
Illustration of execution:
ADDUSER Adnan adnan adnan1 01/01/1991 Selcuk Universitesi
Adnan has been successfully added.
ii. Remove Existing User: The system should also remove an existing user It takes one parameter which indicates userID. REMOVEUSERuserID
Illustration of execution:
REMOVEUSER 1
User has been successfully removed.
No such user!
iii. Show Posts: The system should also be able to display users' posts. The parameter for this action is a username. SHOWPOSTSuserName
Illustration of execution:
SHOWPOSTS userName
adnan does not have any posts yet.
No such user!
**************
adnan's Posts
**************
1st text post
Date: 14.04.2017
Location: 39.8833431, 32.7381663
--------------------------------
1st text post
Date: 14.04.2017
Location: 39.8833431, 32.7381663
Friends tagged in this post: ahmet, demet, adnan
-----------------------------------
1st image post
Date: 14.04.2017
Location: 42.8833431, 32.6381533
Friends tagged in this post: demet, gizem
Image: image.png
Image resolution: 135x250
-------------------------------------
1st video post
Date: 14.04.2017
Location: 39.8833431, 32.6381533
Friends tagged in this post: utku
Video: myvideo.avi
Video duration: 8 minutes
--------------------------------------
iv. Sign-In: This is the base action for users to perform before they are allowed to interact with the system and execute other commands. As in a real application, this evaluator
takes two parameters that are a username and a password. SIGNINuserNamepassword
Illustration of execution:
SIGNIN adnan adnan1
You have successfully signed in.
Invalid username or password! Please try again.
v. Sign-Out: It prevents the user from executing any scenarios other than sign-in. No argument needed to perform this command since it is assumed only one user can log in
to the system at a particular time. SIGNOUT
Illustration of execution:
SIGNOUT
You have successfully signed out.
vi. Update Profile: User who has already logged in may change his/her personal information (name, date of birth, and school from which (s)he graduated). It takes three
arguments and these are assumed to be the updated values. UPDATEPROFILEnamedateofBirthschoolGraduated
Illustration of execution:
UPDATEPROFILE Adnan 07/01/1991 Mugla S.K. Universitesi
Your user profile has been successfully updated
Error: Please sign in and try again
vii. Change Password: A user may want to change his/her password. The system should first check if the provided password matches the current password.
CHPASSoldPasswordnewPassword
Illustration of execution:
CHPASS adnan1 adnan123
Password mismatch!
Error: Please sign in and try again.
viii. Add New Friend: Friendship is the most important point in mysocialbook and without which probably it would not gain much attention. The system you will develop should
allow a user who has signed in to add an existing user as a friend. It takes only one argument, which indicates user name of the user who will be added. ADDFRIENDuserName
Illustration of execution:
ADDFRIEND ahmet
ahmet has been successfully added to your friend list.
This user is already in your friend list!
No such user!
Error: Please sign in and try again.
ix. Remove Friend: A user can also remove a user from his/her friend list. This operator takes one argument that is a username. REMOVEFRIENDuserName
Illustration of execution:
REMOVEFRIEND ahmet
ahmet has been successfully removed from your friend list.
No such friend!
Error: Please sign in and try again.
x. Add Text Post: User can create a new text post by specifying the text content, location (longitude & latitude), and username of the friends who will be tagged to this post, each of the usernames is separated by column (:) mark. ADDPOST-TEXTtextContentlongitudelatitude user-Name1<:>userName2<:>..<:>userNameN
Illustration of execution:
ADDPOST-TEXT 1st text post 39.88 32.73 ahmet:demet:adnan
The post has been successfully added.
ahmet is not your friend, and will not be tagged!
The post has been successfully added.
ahmet is not your friend, and will not be tagged!
demet is not your friend, and will not be tagged!
The post has been successfully added.
Error: Please sign in and try again.
xi. Add Image Post: A user can also create an image or a video post. In this scenario,the image path and resolution information should be provided to the system as well.
ADDPOST-IMAGEtextContentlongitudelatitude user- Name1<:>userName2<:>..<:>userNameNfilePathresolution
Illustration of execution:
ADDPOST-IMAGE 1st image post 42.88 32.63 demet:gizem img.png 135x250
The post has been successfully added.
demet is not your friend, and will not be tagged!
The post has been successfully added.
demet is not your friend, and will not be tagged!
gizem is not your friend, and will not be tagged!
The post has been successfully added.
Error: Please sign in and try again.
xii. Add Video Post: User should only provide the file path and duration of the video. ADDPOST-VIDEOtextContentlongitudelatitude user-
Name1<:>userName2<:>..<:>userNameNfilePathvideoDuration
Illustration of execution:
ADDPOST-VIDEO 1st video post 39.88 32.63 utku:gizem myvideo.avi 8
The post has been successfully added.
gizem is not your friend, and will not be tagged!
The post has been successfully added.
utku is not your friend, and will not be tagged!
gizem is not your friend, and will not be tagged!
The post has been successfully added.
Error: Your video exceeds maximum allowed duration of 10 minutes.
Error: Please sign in and try again.
xiii. Remove Post: User is able to remove a post that has been created the latest. REMOVELASTPOST
Illustration of execution:
REMOVELASTPOST
Your last post has been successfully removed.
Error: You do not have any post.
Error: Please sign in and try again.
xiv. Block User: As in many of the real-world social platforms, a user may block another user due to several reasons (disturbing posts, etc.) in mysocialbook as well. Users can
block any other user through her/his username as argument. BLOCKuserName
Illustration of execution:
BLOCK ahmet
ahmet has been successfully blocked.
No such user!
Error: Please sign in and try again.
xv. Unblock User: The system should also allow a user to unblock another user who has been blocked by him/her. UNBLOCKuserName
Illustration of execution:
UNBLOCK ahmet
ahmet has been successfully unblocked.
No such user in your blocked-user list!
Error: Please sign in and try again.
xvi. List Friends: Users should be able to lists their friends no matter they are blocked or not. LISTFRIENDS
Illustration of execution:
LISTFRIENDS
You have not added any friend yet!
Error: Please sign in and try again.
Name: Adnan
Username: adnan
Date of Birth: 01/01/1991
School: Selcuk Universitesi
-----------------------------------
Name: Ahmet
Username: ahmet
Date of Birth: 04/25/2001
School: Meram Anadolu Lisesi
------------------------------------------
xvii. List Users: Users should be able to view all users in the system. LISTUSERS
Illustration of execution:
LISTUSERS
Error: Please sign in and try again.
Name: Adnan
Username: adnan
Date of Birth: 01/01/1991
School: Selcuk Universitesi
-----------------------------------------
Name: Ahmet
Username: ahmet
Date of Birth: 04/25/2001
School: Meram Anadolu Lisesi
xviii. Show Blocked Friends: Users should be able to lists their friends whom they have blocked. SHOWBLOCKEDFRIENDS
Illustration of execution:
SHOWBLOCKEDFRIENDS
You haven't blocked any friend yet!
Error: Please sign in and try again.
Name: Adnan
Username: adnan
Date of Birth: 01/01/1991
School: Selcuk Universitesi
xix. Show Blocked Users: Similar to the previous scenario, users should be able to lists all users whom they have blocked including no-friendship. SHOWBLOCKEDUSERS
Illustration of execution:
SHOWBLOCKEDUSERS
You haven't blocked any user yet!
Error: Please sign in and try again.
Name: Adnan
Username: adnan
Date of Birth: 01/01/1991
School: Selcuk Universitesi
---------------------------------------------
Name: Ahmet
Username: ahmet
Date of Birth: 04/25/2001
School: Meram Anadolu Lisesi
Input and File for Commands
mysocialbook platform should read a tab-separated file named commands.txt. A detailed explanation, format and expected output for each command is given before.
users.txt
Ahmet ahmet ahmet123 04/25/2001 Meram Anadolu Lisesi
Demet demet demet00 01/16/1999 Ankara Fen Lisesi
Zeki zeki zeki01 08/16/1987 Kadikoy Lisesi
Gizem gizem gizem1 12/09/1997 Hacettepe Universitesi
Utku utku utku99 10/06/1999 Bilkent Universitesi
Hakan hakan hakan81 03/01/1981 Mugla S.K. Üniversitesi
commands.txt:
ADDUSERAdnanadnanadnan101/01/1991Selcuk Universitesi
REMOVEUSER1
SIGNINcemilcemil1
SIGNINadnanadnan1
LISTUSERS
UPDATEPROFILEAdnan07/01/1991Gazi University
CHPASSadnan123adnan1234
CHPASSadnan1adnan123
ADDFRIENDahmet
ADDFRIENDdemet
ADDFRIENDdemet
ADDFRIENDgizem
ADDFRIENDutku
ADDFRIENDziya
REMOVEFRIENDzeki
REMOVEFRIENDziya
REMOVEFRIENDutku
LISTFRIENDS
ADDPOST-TEXTThis is my 1st text post39.232.81ahmet
ADDPOST-IMAGEThis is my 1st image post37.8732.46demet:gizemimage.png135x250
ADDPOST-VIDEOThis is my 1st video post40.8729.24utku:gizemmyvideo.avi8
ADDPOST-TEXTThis is my 2nd text post38.3533.1demet:gizem:utku
REMOVELASTPOST
SHOWPOSTSadnan
BLOCKdemet
BLOCKgizem
BLOCKahmet
SHOWBLOCKEDFRIENDS
UNBLOCKziya
UNBLOCKgizem
UNBLOCKgizem
SHOWBLOCKEDFRIENDS
SHOWBLOCKEDUSERS
SIGNOUT
expected_output
-----------------------
Command: ADDUSERAdnanadnanadnan101/01/1991Selcuk Universitesi
Adnan has been successfully added.
-----------------------
Command: REMOVEUSER1
User has been successfully removed.
-----------------------
Command: SIGNINcemilcemil1
-----------------------
Command: SIGNINadnanadnan1
You have successfully signed in.
-----------------------
Command: LISTUSERS
Name: Demet
Username: demet
Date of Birth: 16/01/1999
Username: Ankara Fen Lisesi
---------------------------
Name: Zeki
Username: zeki
Date of Birth: 16/08/1987
Username: Kadikoy Lisesi
---------------------------
Name: Gizem
Username: gizem
Date of Birth: 09/12/1997
Username: Hacettepe Universitesi
---------------------------
Name: Utku
Username: utku
Date of Birth: 06/10/1999
Username: Bilkent Universitesi
---------------------------
Name: Hakan
Username: hakan
Date of Birth: 01/03/1981
Username: Mugla S.K. Üniversitesi
---------------------------
Name: Adnan
Username: adnan
Date of Birth: 01/01/1991
Username: Selcuk Universitesi
---------------------------
-----------------------
Command: UPDATEPROFILEAdnan07/01/1991Gazi University
-----------------------
Command: CHPASSadnan123adnan1234
Password mismatch!
-----------------------
Command: CHPASSadnan1adnan123
-----------------------
Command: ADDFRIENDahmet
No such user!
-----------------------
Command: ADDFRIENDdemet
demet has been successfully added to your friend list.
-----------------------
Command: ADDFRIENDdemet
This user is already in your friend list!
-----------------------
Command: ADDFRIENDgizem
gizem has been successfully added to your friend list.
-----------------------
Command: ADDFRIENDutku
utku has been successfully added to your friend list.
-----------------------
Command: ADDFRIENDziya
No such user!
-----------------------
Command: REMOVEFRIENDzeki
No such friend!
-----------------------
Command: REMOVEFRIENDziya
Error: Please sign in and try again.
-----------------------
Command: REMOVEFRIENDutku
utku has been successfully removed from your friend list.
-----------------------
Command: LISTFRIENDS
Name: Demet
Username: demet
Date of Birth: 16/01/1999
Username: Ankara Fen Lisesi
---------------------------
Name: Gizem
Username: gizem
Date of Birth: 09/12/1997
Username: Hacettepe Universitesi
---------------------------
-----------------------
Command: ADDPOST-TEXTThis is my 1st text post39.232.81ahmet
ahmet is not your friend, and will not be tagged!
The post has been successfully added.
-----------------------
Command: ADDPOST-IMAGEThis is my 1st image post37.8732.46demet:gizemimage.png135x250
The post has been successfully added.
-----------------------
Command: ADDPOST-VIDEOThis is my 1st video post40.8729.24utku:gizemmyvideo.avi8
utku is not your friend, and will not be tagged!
The post has been successfully added.
-----------------------
Command: ADDPOST-TEXTThis is my 2nd text post38.3533.1demet:gizem:utku
utku is not your friend, and will not be tagged!
The post has been successfully added.
-----------------------
Command: REMOVELASTPOST
Your last post has been successfully removed.
-----------------------
Command: SHOWPOSTSadnan
**************
Adnan's Posts
**************
This is my 1st text post
Date: Mon May 03 22:33:38 EET 2021
Location: 32.81, 39.2
----------------------
This is my 1st image post
Date: Mon May 03 22:33:38 EET 2021
Location: 32.46, 37.87
Friends tagged in this post: Demet, Gizem
Image: image.png
Image resolution: 135x250
----------------------
This is my 1st video post
Date: Mon May 03 22:33:38 EET 2021
Location: 29.24, 40.87
Friends tagged in this post: Gizem
Video: myvideo.avi
Video duration: 8.0 minutes
----------------------
-----------------------
Command: BLOCKdemet
demet has been successfully blocked.
-----------------------
Command: BLOCKgizem
gizem has been successfully blocked.
-----------------------
Command: BLOCKahmet
No such user!
-----------------------
Command: SHOWBLOCKEDFRIENDS
Name: Demet
Username: demet
Date of Birth: 16/01/1999
Username: Ankara Fen Lisesi
---------------------------
Name: Gizem
Username: gizem
Date of Birth: 09/12/1997
Username: Hacettepe Universitesi
---------------------------
-----------------------
Command: UNBLOCKziya
No such user!
-----------------------
Command: UNBLOCKgizem
gizem has been successfully unblocked.
-----------------------
Command: UNBLOCKgizem
No such user in your blocked-user list!
-----------------------
Command: SHOWBLOCKEDFRIENDS
Name: Demet
Username: demet
Date of Birth: 16/01/1999
Username: Ankara Fen Lisesi
---------------------------
-----------------------
Command: SHOWBLOCKEDUSERS
Name: Demet
Username: demet
Date of Birth: 16/01/1999
Username: Ankara Fen Lisesi
---------------------------
-----------------------
Command: SIGNOUT
You have successfully signed out.
Command for Running the Application
java MySocialBook users.txt commands.txt
Notes
• Your source code should be designed as easy-to-follow. Place a comment in it as much as possible. Create separate source code FIles for the tasks you handled.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
postjava package comsimplecodingsocialmodel import javaxpersistence import javautilDate Entity Tablename posts public class Post Id GeneratedValuestrategy GenerationTypeIDENTITY private Integer id Man...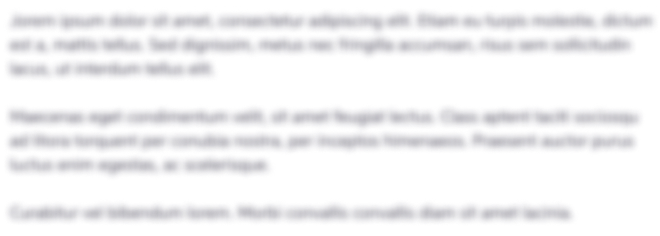
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started