Question
IN JAVA Part A: Payment Write three classes to represent payments. A payment is represented by the amount of money and the date of the
IN JAVA
Part A: Payment
Write three classes to represent payments.
A payment is represented by the amount of money and the date of the payment.
A cash payment provides no additional information.
A credit card payment is represented by the amount and the date, but also by the payer's name and credit card number.
In each class, include (or use inherited versions of):
instance data variables
one or more constructors
getters and setters
a toString method
In the credit card payment class, write an equals method.
Two credit card payments are logically equivalent if they have the same amount, date, name, and credit card number.
Note: you are not required to submit a driver program, but I strongly recommend you write one to test your classes!
For full credit, follow good principles of object-oriented design and inheritance. Think carefully about:
the best data types for instance data variables
the parent-child relationship should be
what classes/methods should be abstract (if any)
avoiding duplicated code
Part B: Animal Kingdom
Write classes and interfaces to represent the following:
Adoptable
Amphibian
Animal
Bat
Cat
Fish
Frog
Flyable
Goldfish
HumpbackWhale
Mammal
WaterLiveable
Whale
You need to decide on the structure of the classes/interfaces. Consider:
Which should be an abstract class?
Which should be an interface?
How should the classes be related through inheritance?
In what classes should methods be placed?
What methods should be overridden?
Some additional details/requirements:
All animals have a name.
All classes have a toString method that returns the animal's name.
The method can be in the class directly or inherited.
All animals have a method "isWarmBlooded" that returns a boolean.
The method can be in the class directly or inherited.
Animals that can be adopted as pets have a method "getHomeCareInstructions" that return a description of how to care for the animal.
Animals that can live underwater have a method "canLiveOnLand" that returns a boolean of whether the animal can also live on land.
Animals that can fly have a method "getFlightSpeed" that returns the average miles per hour that the animal can fly.
This part of the assignment isn't necessarily difficult from a programming perspective. What you should spend time on is carefully considering the design of you classes and how they should be related through inheritance or interfaces. To get full credit:
Your class hierarchy should make sense.
You can assume common knowledge or "googleable" facts about animals- you won't be graded on your biology knowledge!
Place common code as high up in the hierarchy as possible.
Declare classes that should not be instantiated as abstract.
In abstract classes, methods that must be implemented by all subclasses should be declared abstract.
Remember that classes can only have one parent but can implement multiple interfaces.
Below is a test file you can use to test your code.
Note that this file might not contain all possibilities you want to test!
You can edit this file to add more tests- consider doing this!
Animal Kingdom Tester File
public class AnimalKingdomTester {
public static void main(String[] args) {
Animal[] animals = new Animal[5];
animals[0] = new Cat("Mr. Meowerson");
animals[1] = new HumpbackWhale("Blubber");
animals[2] = new Bat("Dracky", 15);
animals[3] = new Goldfish("Nemo");
animals[4] = new Frog("Prince");
for(Animal a : animals) {
System.out.println(a + " is a " +
a.getClass().getSimpleName() + " which " +
(a.isWarmBlooded() ? "is" :
"is not" ) + " warm blooded.");
if(a instanceof Adoptable)
System.out.println("\t" + ( (Adoptable)
a).getHomeCareInstructions());
if(a instanceof Flyable)
System.out.println("\tFlies up to " + (
(Flyable) a).getFlightSpeed() + " mph!");
if(a instanceof WaterLiveable)
System.out.println("\t"+a.getName()+"
can live in the water " +
((
(WaterLiveable) a).canLiveOnLand() ? " and can also" : "but cannot" ) +
" live on
land.");
}
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
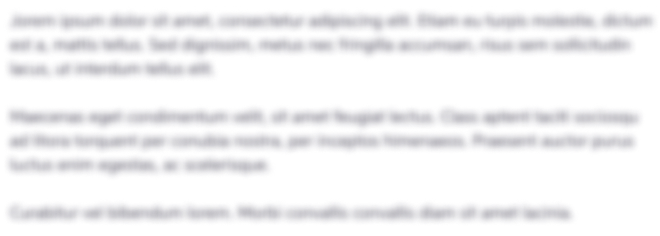
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started