Question
IN JAVA PLEASE 1. Write the VisitProcedure and Appointment classes. 2. Write two classes to handle the user interface. Part 1 - Class Specifications Class
IN JAVA PLEASE
1. Write the VisitProcedure and Appointment classes.
2. Write two classes to handle the user interface.
Part 1 - Class Specifications
Class VisitProcedure
Member Variables (all private)
Variable | Data Type | Description |
Procedure | Procedure | Contains the procedure being done. |
Quantity | Boolean | Contains the quantity of the procedure being done. |
IsCoveredByInsurance | Boolean | Indicates whether or not this procedure is covered by insurance. |
PctCovered | Double | The percentage of the procedure that is covered by insurance. The percentage should be given in the range 0.0 -1.0. For example, 50% would be .5 . |
Member Method Signatures and Descirptions (all public)
Signature | Description |
Default Constructor | Default constructor. Sets the values of each member variable to default values. Hint: Make sure that new is called for reference types. |
Get/set methods for all member variables | The get/set values for reference types should just get and set instance references. |
double CalculateProcedureAmount() | Returns the procedure amount (this does NOT take insurance into account). This is the price of the procedure times the quantity. Note: This method should NOT print anything on the screen. It just returns the data from the method. |
double CalculateProcedureAmountCovered() | Returns the procedure amount that is covered by insurance. If the procedure is not covered (check IsCovered member variable) then return 0. If the procedure is covered then calculate the amount covered from the procedure amount using percent covered. Note: This method should NOT print anything on the screen. It just returns the data from the method. |
double CalculateProcedureAmountDue() | Returns the procedure amount that the customer actually has to pay (not covered by insurance). This is the price of the procedure times the quantity with the amount covered by insurance subtracted from it. You should only take insurance into account if the IsCovered member is true. For example, assume the following: price = 100, quantity=2, IsCoveredByInsurance=true, PctCovered=.75. The procedure amount is 200. The amount covered by insurance is 150. This means the procedure amount due is 50. So the value 50 would be returned from the function for this example. Note: This method should NOT print anything on the screen. It just returns the data from the method. |
void Write(PrintStream ps) | Write the contents of all member variables to the given instance of PrintStream. Assume the PrintStream is already open and ready to use. Data should be written on to separate lines. DO NOT ADD ANY DESCRIPTIVE TEXT IN THE OUTPUT. JUST PRINT THE VALUES. IMPORTANT - Whatever data is written out should be readable by the Read method of this class. If descriptive text is added then Read will not work. |
void Read(Scanner s) | Read the contents of all member variables from the given instance of Scanner. Assume the following inside the method: Scanner is already open. Member variable values are on separate lines. |
String GetJSON() | This method should return a string using JSON formatting (). Here is the format: { variable name : value, } Each variable name should be surrounded by quotes. If the variable data type is String then the value should be surrounded by double quotes. There should be a comma between each pair (no comma after the last pair). Check at the end of document for an example of a properly formatted JSON string for this class. |
@Override String toString() | This method should return a String instance (not print on the screen) that contains the values of member variables with descriptive text. Here is an example of a string that gets returned from the method. For example: Name: Physical Therapy Price: $100.00 Quantity: 2.0 Is Covered By Insurance: true Pct Covered: 0.5 |
Class Appointment
Store in project/package: .bcs345.hwk.vet.business
Member Variables (all private)
VARIABLE | Data Type | DESCRIPTION |
Month | INT | Contains the month of the appointment. |
Day | INT | Contains the day of the appointment. |
Year | INT | Contains the year of the appointment. |
Pet | Pet | Contains the pet being seen at the appointment. |
Member Method Signatures and Descirptions (all public)
SIGNATURE | DESCRIPTION |
Default Constructor | Default constructor. Sets the values of each member variable to default values. Hint: Make sure that new is called for reference types. |
Get/set methods for all member variables | The get/set values for reference types should just get and set instance references. |
void Write(PrintStream ps) | Write the contents of all member variables to the given instance of PrintStream. Assume the PrintStream is already open and ready to use. Data should be written on to separate lines. DO NOT ADD ANY DESCRIPTIVE TEXT IN THE OUTPUT. JUST PRINT THE VALUES. IMPORTANT - Whatever data is written out should be readable by the Read method of this class. If descriptive text is added then Read will not work. |
void Read(Scanner s) | Read the contents of all member variables from the given instance of Scanner. Assume the following inside the method: Scanner is already open. Member variable values are on separate lines. |
STRING GetJSON() | This method should return a string using JSON formatting (). Here is the format: { variable name : value, } Each variable name should be surrounded by quotes. If the variable data type is String then the value should be surrounded by double quotes. There should be a comma between each pair (no comma after the last pair). Check at the end of document for an example of a properly formatted JSON string for this class. |
@OVERRIDE STRING toSTRING() | This method should return a String instance (not print on the screen) that contains the values of member variables with descriptive text. Here is an example of a string that gets returned from the method. For example: Date: 9/3/2018 Name: Snickers Species: Dog Gender: Male |
Class VisitProcedureConsoleUI
Store in project/package: .bcs345.hwk.vet.presentation
Member Variables (all private)
VARIABLE | Data Type | DESCRIPTION |
No member variables |
Member Method Signatures and Descriptions (all public)
SIGNATURE | DESCRIPTION |
void ShowUI() | Shows the user interface and handles user choices. When this method is called it should do the following: Display the menu to the user. Process the user selections. There should be no display or processing code in main. See the Menu Description section below for details. |
Class Main (revise from previous assignment)
Store in project/package: .bcs345.hwk.vet.presentation
Member Variables (all private)
VARIABLE | Data Type | DESCRIPTION |
No member variables |
Member Method Signatures and Descriptions (all public)
SIGNATURE | DESCRIPTION |
public static void main(String args[]) | Just create an instance of VisitProcedureConsoleUI inside of main and call the ShowUI method on it. No other code should be in main. Comment out any code that was previously in main. |
VisitProcedure UI Menu Description
This program will present a menu to the user and then perform an action depending on what the user chooses to do. You should create an instance of VisitProcedure inside of ShowUI. When the program runs it should display the menu to the user and give them a chance to input a choice. An action should be taken depending on what choice the user makes. Here is the user menu:
Visit Procedure UI
------------------
1 Read visit procedure from file
2 Write visit procedure to file
3 Show visit procedure data with descriptive text on screen
4 Show visit procedure JSON on screen
5 - Exit
Enter Choice:
THE PROGRAM SHOULD KEEP SHOWING THE MENU AND PERFORMING AN ACTION UNTIL THE USER CHOOSES TO EXIT.
CHOICE | ACTION |
1 | Reads in one visit procedures data into a VisitProcedure instance from a user-specified file. This menu option expects data to come in according to the VisitProcedure file format specified at the end of the assignment. The user should be prompted to enter a filename to read from. Hint: You can use a function on VisitProcedure to help out with this. |
2 | Writes data from the VisitProcedure instance to a file. The user should be prompted to enter a filename to write the data to. Data should be written out according to the VisitProcedure file format specified at the end of this assignment. Hint: You can use a method on VisitProcedure to help out with |
3 | Show all data from the VisitProcedure instance data on the screen. This data should have descriptive text in it. Hint: You can use a method on VisitProcedure to help out with this. |
4 | Show VisitProcedure JSON output on the screen. Hint: You can use a method on VisitProcedure to help out with this. |
5 | Do not call System.exit to do this. |
VisitProcedure File Format
ProcedureName
ProcedureCost
Quantity
IsCoveredByInsurance
PctCovered
VisitProcedure Sample Input File (VisitProcedure.txt)
Physical Therapy
100.00
2.0
true
.5
VisitProcedure Sample JSON
{
"procedure" :
{
"name" : "Physical Therapy",
"price" : 100.0
},
"quantity" : 2.0,
"isCoveredByInsurance" : true,
"pctCovered" : 0.5
}
Appointment File Format
VisitMonth
VisitDay
VisitYear
PetName
PetSpecies
PetGender
Appointment Sample Input File (Appointment.txt)
9
3
2018
Snickers
Dog
Male
Appointment Sample JSON
{
"month" : 9,
"day" : 3,
"year" : 2018,
"pet" :
{
"name" : "Snickers",
"species" : "Dog",
"gender" : "Male"
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
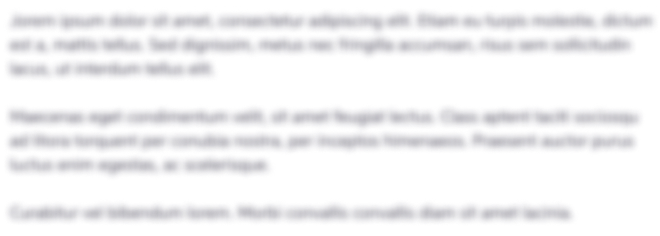
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started