Question
In Java, please help me improve/fix my Java code to run the following modular program with a GUI interface that verifies student grades. Create a
In Java, please help me improve/fix my Java code to run the following modular program with a GUI interface that verifies student grades.
Create a Student class with the following:
-Homework, which is 60% of the final grade -Participation, which is 10% of the final grade -Project, which is 30% of the final grade -Student name
The Student class should calculate the grade average based on the given weights. Test the class with a main method.
Develop a GUI that displays the following menu:
Read Student File Save Student File Save Student Report Search for Student Update Student Add Student Show Students Delete Student
Use text fields for corresponding input and a text area to display output. Use JOptionPane to display messages or get information from the user as necessary. The methods JOptionPane.showMessageDialog and JOptionPane.ShowInputDialog can be used as needed. Use the console, System.out.println(), to log information and debug if necessary. You can leave them in the code if you want.
Following is a possible GUI but feel free to develop a variation of your own. It consists of labels and text areas for input/output fields, a pull down for commands, a button to execute a selected command, and a text area to display results if necessary.
All data should be validated from the form, so you dont have to revalidate the data when objects are created and modified. You can use exception processing and range checking for the numeric fields. Make sure something is entered for the name field and do exception processing for the input/ output. Depending on the selection, the necessary text fields will be read from the form (or updated to the form). For instance, if a report is selected it should be output to a file given in the corresponding text field and to the output text area.
HERE IS MY CODE. PLEASE INCLUDE A SCREENSHOT OF YOUR PROGRAM AND SUGGEST HOW MANY CLASSES TO CREATE to run this program. I use Eclipse and very new to coding.
-------
import java.awt.*;
import java.awt.event.*;
import javax.swing.*;
class StudForm{
static JTextField name_txt ;
static JTextField part_txt;
static JTextField home_txt;
static JTextField proj_txt;
static JTextField avg_txt;
static JTextField inf_txt;
static JTextField ouf_txt;
static JFrame frame;
static JComboBox foop;
static JTextArea add_txtArea;
static JTextField phone_txt;
static JTextField email_txt;
static JCheckBox chkbox;
static JButton submit_btn;
static JTextArea output_txtArea;
public static void main(String args[])
{
frame=new JFrame("Registration Form Example");
frame.setBounds(200,100,700,600 );
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
Container c=frame.getContentPane();
c.setLayout(null);
Font f=new Font("Arial",Font.BOLD,20);
JLabel heading_lbl=new JLabel();
heading_lbl.setBounds(250,5,200,40);
heading_lbl.setText("Student Grade Form");
heading_lbl.setFont(f);
Font f1=new Font("Arial",Font.BOLD,14);
JLabel name_lbl=new JLabel("Name : ");
name_lbl.setBounds(50,80,100,30);
name_txt=new JTextField();
name_txt.setBounds(180,80,200,30);
JLabel part_lbl=new JLabel("Participation ");
part_lbl.setBounds(50,120,150,30);
part_txt=new JTextField();
part_txt.setBounds(180,120,200,30);
JLabel home_lbl=new JLabel("Homework ");
home_lbl.setBounds(50,160,150,30);
home_txt=new JTextField();
home_txt.setBounds(180,160,200,30);
JLabel proj_lbl=new JLabel("Project");
proj_lbl.setBounds(50,200,150,30);
proj_txt=new JTextField();
proj_txt.setBounds(180,200,200,30);
JLabel avg_lbl=new JLabel("Average");
avg_lbl.setBounds(50,240,150,30);
avg_txt=new JTextField();
avg_txt.setBounds(180,240,200,30);
JLabel inf_lbl=new JLabel("Input File ");
inf_lbl.setBounds(50,280,150,30);
inf_txt=new JTextField();
inf_txt.setBounds(180,280,200,30);
JLabel ouf_lbl=new JLabel("Output File");
ouf_lbl.setBounds(50,320,150,30);
ouf_txt=new JTextField();
ouf_txt.setBounds(180,320,200,30);
Cursor cur=new Cursor(Cursor.HAND_CURSOR);
String arr[]={"Read Student File","Save Student File","Save Student Report","Search for Student","Update Average","Add Student","Show Students","Delete Student" };
foop=new JComboBox(arr);
foop.setBounds(50,360,150,30);
submit_btn=new JButton("Process Selection");
submit_btn.setBounds(220,360,180,30);
submit_btn.setCursor(cur); // Applying hand cursor on the button
submit_btn.addActionListener(new ActionListener(){
public void actionPerformed(ActionEvent event){
submit_action(event);
}
});
output_txtArea=new JTextArea();
output_txtArea.setBounds(50,400,260,320);
// Step 13 : Applying Global Font on all the JLabels
name_lbl.setFont(f1);
part_lbl.setFont(f1);
home_lbl.setFont(f1);
proj_lbl.setFont(f1);
avg_lbl.setFont(f1);
inf_lbl.setFont(f1);
ouf_lbl.setFont(f1);
name_txt.setFont(f1);
part_txt.setFont(f1);
home_txt.setFont(f1);
proj_txt.setFont(f1);
avg_txt.setFont(f1);
inf_txt.setFont(f1);
ouf_txt.setFont(f1);
submit_btn.setFont(f1);
output_txtArea.setFont(f1);
frame.setVisible(true);
c.add(heading_lbl);
c.add(name_lbl);
c.add(part_lbl);
c.add(home_lbl);
c.add(proj_lbl);
c.add(avg_lbl);
c.add(inf_lbl);
c.add(ouf_lbl);
c.add(name_txt);
c.add(part_txt);
c.add(home_txt);
c.add(proj_txt);
c.add(avg_txt);
c.add(inf_txt);
c.add(ouf_txt);
c.add(foop);
c.add(output_txtArea);
c.add(submit_btn);
}
public static void submit_action(ActionEvent event)
{
String name=inf_txt.getText();
String part=inf_txt.getText();
String hw=inf_txt.getText();
String pr=inf_txt.getText();
String avg=inf_txt.getText();
String inf=inf_txt.getText();
String of=inf_txt.getText();
String foop_name=(String)foop.getSelectedItem();
if(!name.equals(null) || !part.equals(null)|| !hw.equals(null)|| !pr.equals(null)|| !avg.equals(null)|| !inf.equals(null)|| !of.equals(null))
{
if(foop_name.equals("Save Student File") )
JOptionPane.showMessageDialog(frame,"Input Field cannot be empty.","Alert",JOptionPane.WARNING_MESSAGE);
}
output_txtArea.setText("input file is missing");
if(foop_name.equals("Read Student File") && inf.equals(" "))
output_txtArea.setText("input file is missing");
//like this continue for other comboitems
}
}
Name Participation Homework Project Average Input File Output File Read Student FileProcess Selection Read Student File Save Studentyile Save Student Report Search for Student Update Average Add Student Show Students Delete StudentStep by Step Solution
There are 3 Steps involved in it
Step: 1
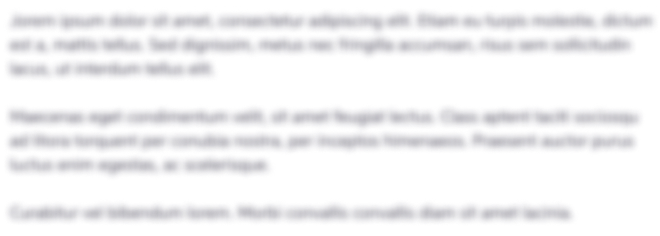
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started