Question
In java please. Part I: Coding Read Part I (coding) and Part II (testing) before starting your assignment. 1. [5 marks] Read the input one
In java please.
Part I: Coding
Read Part I (coding) and Part II (testing) before starting your assignment.
1. [5 marks] Read the input one line at a time and then output the lines in reverse order so that the last line is output first, followed by the second-last line, and so on until the first input line is output last.
2. [5 marks] Imagine each input line is numbered, starting from 0. Read the whole file and then output the even-numbered lines (in order) followed by the odd-numbered lines (also in order).
3. [5 marks] Read the input one line at a time and, if the line numbered i is empty and i42, then output the line numbered i-42. In this way, the number of lines you output is no more than the number of blank lines in the input.
4. [5 marks] Read the input one line at a time and store them in a buffer (a data structure of your choosing). If the current line has odd length, then output the oldest line in the buffer (and remove it from the buffer). In this way, the total number of output lines will be equal to the number of odd-length input lines.
5. [5 marks] Read all of the input lines and then output them in sorted order, using the usual sorted order on Strings. Duplicate lines should be printed only once. Take special care so that a file with a lot of duplicate lines does not use more memory than what is required for the number of unique lines.
6. [5 marks] Read all of the input lines and output them sorted by length, with ties being broken by the usual sorted order. Duplicate lines should be printed only once. Take special care so that a file with a lot of duplicate lines does not use more memory than what is required for the number of unique lines.
7. [5 marks] Read the input one line at a time and, instead of outputting the line, output the number of times you have already seen that line. Again, take special care so that a file with a lot of duplicate lines does not use more memory than what is required for the number of unique lines.
8. [5 marks] Read the input one line at a time, keeping track of the number of times you have seen each line. Once all lines are read, output them in decreasing order of frequency, so that the first line is the one that occurs most frequently. In the case of ties, resolve them using the usual sorted order.
9. [5 marks] Read the whole input one line at a time and output each line if it is not a prefix of some previous line. (One string s is a prefix of another string t if t can be written as t=sx for some string x. For example, s='help' is a prefix of t='helpful' because 'helpful' = 'help' + 'ful'). Take care not to waste memory, so that your code stores the fewest number of lines possible. Hint: Consider what happens when you compare 'help' and 'helpful' using the usual ordering on Strings.
10. [5 marks] Read the entire input one line at a time and then output a subsequence of the lines that appear in the same order they do in the file and that are also in non-decreasing or non-increasing sorted order. If the file contains n lines, then the length of this subsequence should be at least sqrt(n). For example, if the input contains 9 lines with the numbers 2, 1, 3, 7, 4, 6, 9, 5, 8 then your output should have at least 3 lines, which could be 1,3,4 (increasing) or 2,3,7 (increasing) or 1,4,6,9 (increasing) or 2,6,8 (increasing) or 7,6,5 (decreasing) or ...). Warning/Hint: This is probably the hardest question. You're being asked to implement the ErdsSzekeres theorem.
And please answer the question by the following style:
public class Part1 { /** * Your code goes here - see Part0 for an example * @param r the reader to read from * @param w the writer to write to * @throws IOException */ public static void doIt(BufferedReader r, PrintWriter w) throws IOException { // Your code goes here - see Part0 for an example }
/** * The driver. Open a BufferedReader and a PrintWriter, either from System.in * and System.out or from filenames specified on the command line, then call doIt. * @param args */ public static void main(String[] args) { try { BufferedReader r; PrintWriter w; if (args.length == 0) { r = new BufferedReader(new InputStreamReader(System.in)); w = new PrintWriter(System.out); } else if (args.length == 1) { r = new BufferedReader(new FileReader(args[0])); w = new PrintWriter(System.out); } else { r = new BufferedReader(new FileReader(args[0])); w = new PrintWriter(new FileWriter(args[1])); } long start = System.nanoTime(); doIt(r, w); w.flush(); long stop = System.nanoTime(); System.out.println("Execution time: " + 10e-9 * (stop-start)); } catch (IOException e) { System.err.println(e); System.exit(-1); } } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
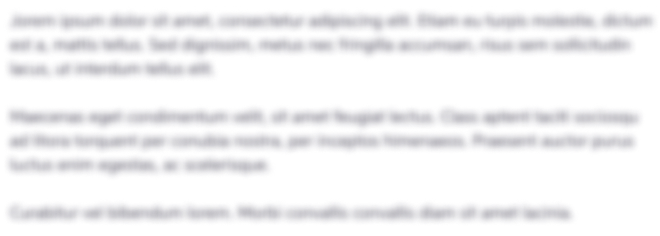
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started