In Java please: public class Imaging{ /*Inverts each value in a 2D array of integers between 0 and 255. A value of 255 becomes 0,
In Java please:
public class Imaging{
/*Inverts each value in a 2D array of integers between 0 and 255. A value of 255 becomes 0, 254 becomes 1,
all the way down to 0 becomes 255.
Parameters:
image - The array of integers with values between 0 and 255 inclusive.
Returns:
A new 2D array with integers inverted.*/
public static int[][] invert(int[][] image){
}
/*Reverses all the values in each row in a 2D array of integers, simulating a horizontal flip..
Parameters:
image - The array of integers.
Returns:
A new 2D array of integers with all the rows reversed.*/
public static int[][] horizontalFlip(int[][] image){
}
/*Reverses all the values in each column in a 2D array of integers, simulating a vertical flip.
Parameters:
image - The array of integers.
Returns:
A new 2D array of integers with all columns reversed.*/
public static int[][] verticalFlip(int[][] image){
}
/*Creates an ASCII image text file with each character represents a range of integer values.
The mapping key is specified in the assignment document.
Parameters:
image - The array of integers with values between 0 and 255 inclusive.
outName - The name of the new text file that will contain the representative characters.*/
public static void makeAscii(int[][] image, String outName){
}
/*Scales a 2D array of integers by a given factor.
Parameters:
image - The 2D array of integers to scale.
scalefactor - The percentage to scale the image by. This value is expected to be greater than 0.
Returns:
A new 2D array resized by the scale.*/
public static int[][] scale(int[][] image, double scalefactor){
}
/*Rotates a 2D array of integers 90 degrees clockwise. The value in the top left corner moves to the
top right corner and all over values move with respect to their orginal position.
Parameters:
image - The array of integers.
Returns:
A new 2D array of integers with values rotated.*/
public static int[][] rotate(int[][] image){
}
/*Reads an image file and converts it to a 2D array of integers. Each value in the array is a
representation of the corresponding pixel's grayscale value.
Parameters:
filename - The name of the image file
Returns:
A 2D array of integers.
Throws:
java.lang.RuntimeException - if the input file cannot be found or read.*/
public static int[][] readGrayscaleImage(String filename){
}
/*Parameters:
args - The input necessary to execute the appropriate conversion. The array is formated in one of the following ways:
scalefactor: A number: 1 maintains original size, < 1 reduces size, > 1 enlarges size.
public static void main(String[] args){
}
}
Image conversion java file needed and used to call certain methods:
import java.awt.Color; import java.awt.image.BufferedImage; import java.io.*; import javax.imageio.ImageIO; /** * Class ImageConversions is a collection of methods that convert jpg images * into variations. */ public class ImageConversions { /** * Handles all input instructions and relays the information to the * appropriate method. * If the input is not as specified, the program stops, with an informative message. * @param args The input necessary to execute the appropriate conversion. * The array is formated in one of the following ways: *
- *
- [infile outfile] invert *
- *
- infile: image file outfile: image file *
* - [infile outfile] verticalFlip *
- [infile outfile] horizontalFlip *
- [infile outfile] makeAscii *
- [infile outfile] scale [scalefactor] *
- *
- scalefactor: A number: 1 maintains original size, < 1 reduces size, > 1 enlarges size. *
* - [infile outfile] rotate *
Step by Step Solution
There are 3 Steps involved in it
Step: 1
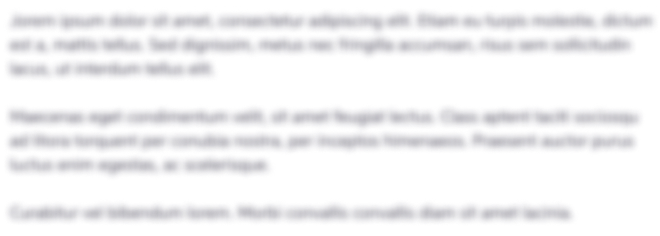
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started