Question
In java please. Show code and required output. Shopper, grocery item and grocerybag class are given. When the window first opens, it should appear (1)
In java please. Show code and required output. Shopper, grocery item and grocerybag class are given.
When the window first opens, it should appear (1) with all buttons disabled, (2) the Products list populated with items, (3) the Shopping Cart and Contents lists should be empty and (4) the Total Price should appear as $0.00, aligned to the right side of the text field and properly formatted. You MUST create an update() method that properly adjusts the contents/view of each list, button and the text field whenever it is called. You should call this at the end of the start() method to populate all the window components. The array below should be used as the contents of the Products list. GroceryItem[] products = { new FreezerItem("Smart-Ones Frozen Entrees", 1.99f, 0.311f), new GroceryItem("SnackPack Pudding", 0.99f, 0.396f), new FreezerItem("Breyers Chocolate Icecream",2.99f,2.27f), new GroceryItem("Nabob Coffee", 3.99f, 0.326f), new GroceryItem("Gold Seal Salmon", 1.99f, 0.213f), new GroceryItem("Ocean Spray Cranberry Cocktail",2.99f,2.26f), new GroceryItem("Heinz Beans Original", 0.79f, 0.477f), new RefrigeratorItem("Lean Ground Beef", 4.94f, 0.75f), new FreezerItem("5-Alive Frozen Juice",0.75f,0.426f), new GroceryItem("Coca-Cola 12-pack", 3.49f, 5.112f), new GroceryItem("Toilet Paper - 48 pack", 40.96f, 10.89f), new RefrigeratorItem("2L Sealtest Milk",2.99f,2.06f), new RefrigeratorItem("Extra-Large Eggs",1.79f,0.77f), new RefrigeratorItem("Yoplait Yogurt 6-pack",4.74f,1.02f), new FreezerItem("Mega-Sized Chocolate Icecream",67.93f,15.03f)};
You will want to make sure that the model for your user interface is your Shopper object from assignment #4, which contains a shopping cart of GroceryItem objects and GroceryBag objects.
Shopper class
public class Shopper { public static final int MAX_CART_ITEMS = 100; // max # items allowed private Carryable[] cart; // items to be purchased private int numItems; // #items to be purchased public Shopper() { cart = new Carryable[MAX_CART_ITEMS]; numItems = 0; } public Carryable[] getCart() { return cart; } public int getNumItems() { return numItems; } public String toString() { return "Shopper with shopping cart containing " + numItems + " items"; } // Return the total cost of the items in the cart public float totalCost() { float total = 0; for (int i=0; i GroceryBag public class GroceryItem implements Carryable{ private String name; private float price; private float weight; public GroceryItem() { name = "?"; price = 0; weight = 0; } public GroceryItem(String n, float p, float w) { name = n; price = p; weight = w; } public String getName() { return name; } public float getPrice() { return price; } public float getWeight() { return weight; } public String toString () { return name + " weighing " + weight + "kg with price $" + price; } public String getDescription() { return name; } public String getContents(){ return ""; } }
public class GroceryBag implements Carryable { public static final float MAX_WEIGHT = 5; // max weight allowed (kg) public static final int MAX_ITEMS = 25; // max # items allowed private GroceryItem[] items; // actual GroceryItems in bag private int numItems; // # of GroceryItems in bag private float weight; // current weight of bag public GroceryBag() { items = new GroceryItem[MAX_ITEMS]; numItems = 0; weight = 0; } public GroceryItem[] getItems() { return items; } public int getNumItems() { return numItems; } public float getWeight() { return weight; } public String toString() { if (weight == 0) return "An empty grocery bag"; return ("A " + weight + "kg grocery bag with " + numItems + " items"); } public boolean canHold(GroceryItem g) { return (((weight + g.getWeight()) <= MAX_WEIGHT) && (numItems <= MAX_ITEMS)); } public void addItem(GroceryItem g) { if (canHold(g)) { items[numItems++] = g; weight += g.getWeight(); } } public void removeItem(GroceryItem item) { for (int i = 0; i < numItems; i++) { if (items[i] == item) { weight -= items[i].getWeight(); items[i] = items[numItems - 1]; numItems -= 1; return; } } } // Finds and returns the heaviest item in the shopping cart public GroceryItem heaviestItem() { if (numItems == 0) return null; GroceryItem heaviest = items[0]; for (int i=0; i
Step by Step Solution
There are 3 Steps involved in it
Step: 1
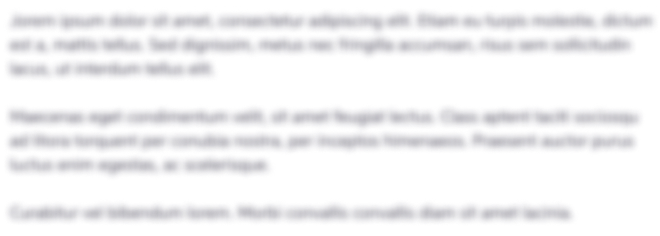
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started