Question
In Java The purpose of the BigInt class is to solve a very large integer problem using short methods that work together to solve the
In Java
The purpose of the BigInt class is to solve a very large integer problem using short methods that work together to solve the operations of add, subtract multiply, divide, and modulus. The constructor will process a String of characters and store them as individual Bytes in an ArrayList. If incoming String contains a leading sign, the constructor will remove it from the String prior to storing the value in the ArrayList. The object's public math methods do not do the actual math. They call helper methods to do the work.
This is a challenging project that requires some thought to be successful.
You must use the test driver programs that I provide to test your implementation of your BigInt class. The first test driver will a test your implementation of the BigInt constructor, clone(), equals(Bigint), compareTo(Bigint), and toString() methods. It will also test your implementation of the BigIntException class. You may not change the test driver programs. There is a second BigInt tester for add, subtract, multiply, divide, and modulus.
Initial requirements:
- BigInt may only have two variables. An ArrayList variable to hole the BigInt digits and a variable to hold the sign.
- Implement two BigInt constructors; one that receives a String argument, and one that receives an integer argument.
- The BigInt(String) constructor must throw a BigIntException if the input to the constructor is invalid.
- Create a BigIntException class that is thrown when the constructor does not create a properly formed integer.
- The clone() method will return a deep copy of the BigInt object.
- The toString() method will return a String representation of the BigInt object.
- The equals(Bigint):boolean method will return true if "this" is mathematically equal to the argument.
- The compareTo(BigInt):int will return -1 if "this" is mathematically less than the argument
- The compareTo(BigInt):int will return 0 if "this" is mathematically equal to the argument.
- The compareTo(BigInt):int will return 1 if "this" is mathematically greater than the argument
__________________________________________________________
Tester
package testerPackage;
import bigIntegerPackage.BigInt; import exceptionPackage.BigIntException;
import java.util.ArrayList; import java.util.Scanner; public class BigIntTesterTwo { private static Scanner keyboard = new Scanner(System.in); public static void main(String[] args) { int numberOfIntegers = 0; ArrayList bigInts = new ArrayList(); BigInt bigNumberOne = null; BigInt bigNumberTwo = null; String stringNumberOne = null; String yesNo = ""; int compareValue = 0; boolean invalidInput = true; do { bigInts.clear(); do { do { invalidInput = true; try { System.out.println("Please enter an integer."); System.out.println("The number can any positive or negative whole number"); stringNumberOne = keyboard.next(); keyboard.nextLine(); bigNumberOne = new BigInt(stringNumberOne); bigInts.add(bigNumberOne); System.out.println("Number one is " + bigNumberOne); invalidInput = false; } catch (BigIntException badValueError) { System.out.println(badValueError.getMessage() + " Please enter a valid whole.");
} } while (invalidInput); System.out.println("Would you like to enter another big integer?"); do { System.out.println("Please enter \"yes\" or \"no\"."); yesNo = keyboard.next(); keyboard.nextLine(); } while (!(yesNo.equalsIgnoreCase("yes") || yesNo.equalsIgnoreCase("no"))); } while (yesNo.equalsIgnoreCase("yes"));
numberOfIntegers = bigInts.size(); bigNumberOne = bigInts.get(0); for (int index = 0; index < numberOfIntegers; index++) { bigNumberTwo = bigInts.get(index); compareValue = bigNumberOne.compareTo(bigNumberTwo); if (bigNumberOne.equals(bigNumberTwo) && compareValue == 0) System.out.printf("%s is equal to %s ", bigNumberOne, bigNumberTwo); else if (compareValue > 0) System.out.printf("%s is larger than %s ", bigNumberOne, bigNumberTwo); else System.out.printf("%s is smaller than %s ", bigNumberOne, bigNumberTwo); }
System.out.println("Would you like to enter a new set of values?"); do { System.out.println("Please enter \"yes\" or \"no\"."); yesNo = keyboard.next(); keyboard.nextLine(); } while (!(yesNo.equalsIgnoreCase("yes") || yesNo.equalsIgnoreCase("no"))); } while (yesNo.equalsIgnoreCase("yes")); }
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
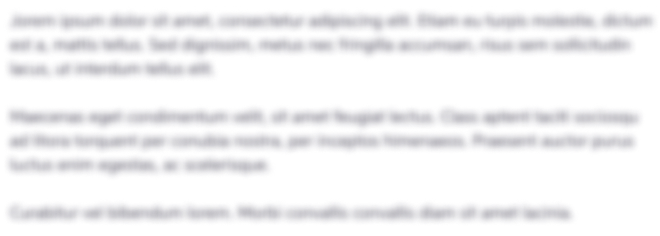
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started