Answered step by step
Verified Expert Solution
Question
1 Approved Answer
IN JAVA, This is code that needs to be in the correct format! I need the program output to match the examples. Please and thank
IN JAVA,
This is code that needs to be in the correct format!
I need the program output to match the examples. Please and thank you!
Starter code:
Print out of starting code to use:
import java.util.ArrayList; import java.util.Scanner; public class TicTacToe { // Static variables for the TicTacToe class, effectively configuration options // Use these instead of hard-coding ' ', 'X', and 'O' as symbols for the game // In other words, changing one of these variables should change the program // to use that new symbol instead without breaking anything // Do NOT add additional static variables to the class! static char emptySpaceSymbol = ' '; static char playerOneSymbol = 'X'; static char playerTwoSymbol = 'O'; public static void main(String[] args) { // TODO // This is where the main menu system of the program will be written. // Hint: Since many of the game runner methods take in an array of player names, // you'll probably need to collect names here. } // Given a state, return a String which is the textual representation of the tic-tac-toe board at that state. private static String displayGameFromState(char[][] state) { // TODO // Hint: Make use of the newline character to get everything into one String // It would be best practice to do this with a loop, but since we hardcode the game to only use 3x3 boards // it's fine to do this without one. return null; } // Returns the state of a game that has just started. // This method is implemented for you. You can use it as an example of how to utilize the static class variables. // As you can see, you can use it just like any other variable, since it is instantiated and given a value already. private static char[][] getInitialGameState() { return new char[][]{{emptySpaceSymbol, emptySpaceSymbol, emptySpaceSymbol}, {emptySpaceSymbol, emptySpaceSymbol, emptySpaceSymbol}, {emptySpaceSymbol, emptySpaceSymbol, emptySpaceSymbol}}; } // Given the player names, run the two-player game. // Return an ArrayList of game states of each turn -- in other words, the gameHistory private static ArrayListrunTwoPlayerGame(String[] playerNames) { // TODO return null; } // Given the player names (where player two is "Computer"), // Run the one-player game. // Return an ArrayList of game states of each turn -- in other words, the gameHistory private static ArrayList runOnePlayerGame(String[] playerNames) { // TODO return null; } // Repeatedly prompts player for move in current state, returning new state after their valid move is made private static char[][] runPlayerMove(String playerName, char playerSymbol, char[][] currentState) { // TODO return null; } // Repeatedly prompts player for move. Returns [row, column] of their desired move such that row & column are on // the 3x3 board, but does not check for availability of that space. private static int[] getInBoundsPlayerMove(String playerName) { Scanner sc = new Scanner(System.in); // TODO return null; } // Given a [row, col] move, return true if a space is unclaimed. // Doesn't need to check whether move is within bounds of the board. private static boolean checkValidMove(int[] move, char[][] state) { // TODO return false; } // Given a [row, col] move, the symbol to add, and a game state, // Return a NEW array (do NOT modify the argument currentState) with the new game state private static char[][] makeMove(int[] move, char symbol, char[][] currentState) { // TODO: // Hint: Make use of Arrays.copyOf() somehow to copy a 1D array easily // You may need to use it multiple times for a 1D array return null; } // Given a state, return true if some player has won in that state private static boolean checkWin(char[][] state) { // TODO // Hint: no need to check if player one has won and if player two has won in separate steps, // you can just check if the same symbol occurs three times in any row, col, or diagonal (except empty space symbol) // But either implementation is valid: do whatever makes most sense to you. // Horizontals // Verticals // Diagonals return false; } // Given a state, simply checks whether all spaces are occupied. Does not care or check if a player has won. private static boolean checkDraw(char[][] state) { // TODO return false; } // Given a game state, return a new game state with move from the AI // It follows the algorithm in the PDF to ensure a tie (or win if possible) private static char[][] getCPUMove(char[][] gameState) { // TODO // Hint: you can call makeMove() and not end up returning the result, in order to "test" a move // and see what would happen. This is one reason why makeMove() does not modify the state argument // Determine all available spaces // If there is a winning move available, make that move // If not, check if opponent has a winning move, and if so, make a move there // If not, move on center space if possible // If not, move on corner spaces if possible // Otherwise, move in any available spot return null; } // Given a game state, return an ArrayList of [row, column] positions that are unclaimed on the board private static ArrayList getValidMoves(char[][] gameState) { // TODO return null; } // Given player names and the game history, display the past game as in the PDF sample code output private static void runGameHistory(String[] playerNames, ArrayList gameHistory) { // TODO // We have the names of the players in the format [playerOneName, playerTwoName] // Player one always gets 'X' while player two always gets 'O' // However, we do not know yet which player went first, but we'll need to know... // Hint for the above: which symbol appears after one turn is taken? // Hint: iterate over gameHistory using a loop } }
I need the code in the right format!
I need the code in the starting code's format!
If it isn't I will down vote it
Your little brother achieved some amazing results thanks to your program. So you decide to reward him by making a game for him. In particular, he loves to play tictac-toe with you so you decide to build that. Furthermore, you want him to be able to compete against the computer as well as you. Hence, your game is going to have two modes: (i) single player mode (against the computer) and (ii) two player mode. In two player mode, your program should first ask the players their names and toss a coin to decide who goes first. Then the program should ask the users to take turns playing the game. Ensure that each move is legitimate and print the game result when someone wins or when the game ends in a draw. In one player mode, your program should first ask for the player's name. You will be playing with the computer. Then it should toss a coin to decide who gets to go first. Player one and player two refer to the order in which the players enter their names. Player one gets the ' C ' character while player two gets the 'O' character. Player one does NOT refer to the player who takes the first turn, since it's based on a coin-flip. When playing one-player mode, the Al will always be player two. Lastly, the menu should repeat unless the user selects the quit option. Menu contains four options that user can select: I. 1. Single player > user will play with computer II. 2. Two player -> user will play with another human player III. D. Display last match -> displays the last played match IV. Q. Quit -> user wish to quit the game, when selected you should display proper message. Refer to sample output for expected functionality. Hints, edge-cases, and design considerations This is a challenging assignment with many moving parts! It would be a great idea to start early to leave ample time to visit office hours and get help when you're stuck. To get you started, this week we provide starter code in the form of an IntelliJ project folder, which has all the headers of the methods you'd must implement to create the program as well as more specific hints for doing so. You are required to use this starter code and you may modify it by adding additional methods, but do not change the existing methods provided. You can unzip the starter code file to get a project folder. Then, open this in IntelliJ and work with it as you would any other project. Make extensive use of methods to organize your code and break the problem down into manageable pieces. The starter code contains all the method headers you would need for this - the solution we wrote has all these methods and no more than these methods. Start with the two-player game since that'll be most similar to what you're familiar with and makes testing easier. After you have that, the only challenge with the one-player game will be implementing the Al algorithms, as the rest of the game logic will carry over and can be reused. You must use an ArrayList to store the game history, and you must use a 2D char array to store a game state. The starter code has three static variables in the TicTacToe class, which are used to centrally store what symbols in the game represent the empty space, the player one symbol, and the player two symbol. Throughout your code, you must refer to these static variables rather than hard coding the game to rely on ' ', ' X ', and 'O'. You may not add additional static variables to the class. These are effectively used as configuration options for the game. Certain methods in the starter code are marked as private. Since we're only using one class, there is no functional difference. Later in the course you'll see why some have their access from other classes restricted in this way. All methods are static. You'll also see why when we cover what it means for methods and variables to be static in detail. Your program should conform to these edge-case expectations regarding user input: - In every menu, check whether the user's choice of menu option is valid and inform them if their choice isn't valid. - When inputting positions on the board, you do not need to handle non-numerical or non-integer inputs. Assume they will only input integers. However, you should check that the integers they inputted are valid. This means their input should both be within the 33 grid and should be an unoccupied space. Inform the user if their input was invalid and which of these two rules it broke. Deliverables \& Reminders The usual deliverables and reminders apply. Compress and submit your project folder to Canvas before the deadline (or after with penalty, per the syllabus). The more serious warnings are listed below, but of course past assignments have more detailed instructions. Ensure your submission contains the necessary file(s) and is the correct version of your code that you want graded. This is your warning. You will not be allowed to resubmit this assignment after the due date if you submit the wrong file. Remember that this is graded on accuracy, and this is a pair project. Plagiarism or cheating will be dealt with seriously. Sample program output Two players, general case with replay display Welcome to game of Tic Tac Toe, choose one of the following options from below: 1. Single player 2. Two player D. Display last match Q. Quit What do you want to do: D No match found. Welcome to game of Tic Tac Toe, choose one of the following options from below: 1. Single player 2. Two player D. Display last match Q. Quit What do you want to do: 2 Enter player 1 name: Nazim Enter player 2 name: Obed Tossing a coin to decide who goes first!!! Nazim gets to go first. I Nazim's turn Nazim enter Nazim enter X I I Obed's turn: Obed enter row: Obed enter col: That space is already taken. Try again. Obed enter row: Obed enter col: 3 That row or column is out of bounds. Try again. Obed enter row: 1 Obed enter col: 1 \begin{tabular}{c} x | 1 \\ \hline 10 I \\ \hline 1 \end{tabular} Nazim's turn: Nazim enter row: 1 Nazim enter col: // Given a [row, col] move, return true if a space is unclaimed. // Doesn't need to check whether move is within bounds of the board. no usages private static boolean checkValidMove(int[] move, char[][] state) \{ // TODO return false; \} // Given a [row, col] move, the symbol to add, and a game state, // Return a NEW array (do NOT modify the argument currentstate) with the new game state no usages private static char[][] makeMove(int[] move, char symbol, char[][] currentState) \{ // TODo: // Hint: Make use of Arrays.copyOf() somehow to copy a 10 array easily // You may need to use it multiple times for a 10 array return null; // Given a state, return true if some player has won in that state nousages private static boolean checkWin(char[][] state) // TODO // Hint: no need to check if player one has won and if player two has won in separate steps, // you can just check if the same symbol occurs three times in any row, col, or diagonal (except empty space sym // But either implementation is valid: do whatever makes most sense to you. // Horizontals // Verticals // Diagonals return false; \} // Given a state, simply checks whether all spaces are occupied. Does not care or check if a player has won. no usages private static boolean checkDraw (char[][] state) \{ // Todo return false; \} // Given a game state, return a new game state with move from the AI // It follows the algorithm in the PDF to ensure a tie possible) // Given a game state, return an Arraylist of [row, column] positions that are unclaimed on the board nousages private static Arraylist getValidMoves(char[][] gamestate) \{ // TODO return null; // Given player names and the game history, display the past game as in the PDF sample code output nousages private static void rungameHistory(String[] playerNames, Arraylist gameHistory) \{ // TOD0 // We have the names of the players in the format [playeroneName, playerTwoName] // Player one always gets 'X' while player two always gets '0' // However, we do not know yet which player went first, but we'll need to know... // Hint for the above: which symbol appears after one turn is taken? // Hint: iterate over gameHistory using a loop Your little brother achieved some amazing results thanks to your program. So you decide to reward him by making a game for him. In particular, he loves to play tictac-toe with you so you decide to build that. Furthermore, you want him to be able to compete against the computer as well as you. Hence, your game is going to have two modes: (i) single player mode (against the computer) and (ii) two player mode. In two player mode, your program should first ask the players their names and toss a coin to decide who goes first. Then the program should ask the users to take turns playing the game. Ensure that each move is legitimate and print the game result when someone wins or when the game ends in a draw. In one player mode, your program should first ask for the player's name. You will be playing with the computer. Then it should toss a coin to decide who gets to go first. Player one and player two refer to the order in which the players enter their names. Player one gets the ' C ' character while player two gets the 'O' character. Player one does NOT refer to the player who takes the first turn, since it's based on a coin-flip. When playing one-player mode, the Al will always be player two. Lastly, the menu should repeat unless the user selects the quit option. Menu contains four options that user can select: I. 1. Single player > user will play with computer II. 2. Two player -> user will play with another human player III. D. Display last match -> displays the last played match IV. Q. Quit -> user wish to quit the game, when selected you should display proper message. Refer to sample output for expected functionality. Hints, edge-cases, and design considerations This is a challenging assignment with many moving parts! It would be a great idea to start early to leave ample time to visit office hours and get help when you're stuck. To get you started, this week we provide starter code in the form of an IntelliJ project folder, which has all the headers of the methods you'd must implement to create the program as well as more specific hints for doing so. You are required to use this starter code and you may modify it by adding additional methods, but do not change the existing methods provided. You can unzip the starter code file to get a project folder. Then, open this in IntelliJ and work with it as you would any other project. Make extensive use of methods to organize your code and break the problem down into manageable pieces. The starter code contains all the method headers you would need for this - the solution we wrote has all these methods and no more than these methods. Start with the two-player game since that'll be most similar to what you're familiar with and makes testing easier. After you have that, the only challenge with the one-player game will be implementing the Al algorithms, as the rest of the game logic will carry over and can be reused. You must use an ArrayList to store the game history, and you must use a 2D char array to store a game state. The starter code has three static variables in the TicTacToe class, which are used to centrally store what symbols in the game represent the empty space, the player one symbol, and the player two symbol. Throughout your code, you must refer to these static variables rather than hard coding the game to rely on ' ', ' X ', and 'O'. You may not add additional static variables to the class. These are effectively used as configuration options for the game. Certain methods in the starter code are marked as private. Since we're only using one class, there is no functional difference. Later in the course you'll see why some have their access from other classes restricted in this way. All methods are static. You'll also see why when we cover what it means for methods and variables to be static in detail. Your program should conform to these edge-case expectations regarding user input: - In every menu, check whether the user's choice of menu option is valid and inform them if their choice isn't valid. - When inputting positions on the board, you do not need to handle non-numerical or non-integer inputs. Assume they will only input integers. However, you should check that the integers they inputted are valid. This means their input should both be within the 33 grid and should be an unoccupied space. Inform the user if their input was invalid and which of these two rules it broke. Deliverables \& Reminders The usual deliverables and reminders apply. Compress and submit your project folder to Canvas before the deadline (or after with penalty, per the syllabus). The more serious warnings are listed below, but of course past assignments have more detailed instructions. Ensure your submission contains the necessary file(s) and is the correct version of your code that you want graded. This is your warning. You will not be allowed to resubmit this assignment after the due date if you submit the wrong file. Remember that this is graded on accuracy, and this is a pair project. Plagiarism or cheating will be dealt with seriously. Sample program output Two players, general case with replay display Welcome to game of Tic Tac Toe, choose one of the following options from below: 1. Single player 2. Two player D. Display last match Q. Quit What do you want to do: D No match found. Welcome to game of Tic Tac Toe, choose one of the following options from below: 1. Single player 2. Two player D. Display last match Q. Quit What do you want to do: 2 Enter player 1 name: Nazim Enter player 2 name: Obed Tossing a coin to decide who goes first!!! Nazim gets to go first. I Nazim's turn Nazim enter Nazim enter X I I Obed's turn: Obed enter row: Obed enter col: That space is already taken. Try again. Obed enter row: Obed enter col: 3 That row or column is out of bounds. Try again. Obed enter row: 1 Obed enter col: 1 \begin{tabular}{c} x | 1 \\ \hline 10 I \\ \hline 1 \end{tabular} Nazim's turn: Nazim enter row: 1 Nazim enter col: // Given a [row, col] move, return true if a space is unclaimed. // Doesn't need to check whether move is within bounds of the board. no usages private static boolean checkValidMove(int[] move, char[][] state) \{ // TODO return false; \} // Given a [row, col] move, the symbol to add, and a game state, // Return a NEW array (do NOT modify the argument currentstate) with the new game state no usages private static char[][] makeMove(int[] move, char symbol, char[][] currentState) \{ // TODo: // Hint: Make use of Arrays.copyOf() somehow to copy a 10 array easily // You may need to use it multiple times for a 10 array return null; // Given a state, return true if some player has won in that state nousages private static boolean checkWin(char[][] state) // TODO // Hint: no need to check if player one has won and if player two has won in separate steps, // you can just check if the same symbol occurs three times in any row, col, or diagonal (except empty space sym // But either implementation is valid: do whatever makes most sense to you. // Horizontals // Verticals // Diagonals return false; \} // Given a state, simply checks whether all spaces are occupied. Does not care or check if a player has won. no usages private static boolean checkDraw (char[][] state) \{ // Todo return false; \} // Given a game state, return a new game state with move from the AI // It follows the algorithm in the PDF to ensure a tie possible) // Given a game state, return an Arraylist of [row, column] positions that are unclaimed on the board nousages private static Arraylist getValidMoves(char[][] gamestate) \{ // TODO return null; // Given player names and the game history, display the past game as in the PDF sample code output nousages private static void rungameHistory(String[] playerNames, Arraylist gameHistory) \{ // TOD0 // We have the names of the players in the format [playeroneName, playerTwoName] // Player one always gets 'X' while player two always gets '0' // However, we do not know yet which player went first, but we'll need to know... // Hint for the above: which symbol appears after one turn is taken? // Hint: iterate over gameHistory using a loopStep by Step Solution
There are 3 Steps involved in it
Step: 1
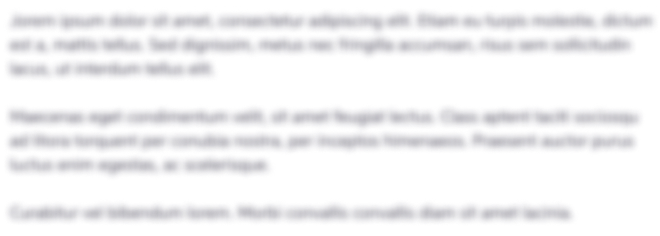
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started