Question
In java This lab implements the i. Auto classes. Note: a. Car i. Sedan ii. Coupe b. Truck i. Pickup ii. Semi Do NOT create
In java
This lab implements the i. Auto
classes. Note:
a. Car i. Sedan
ii. Coupe b. Truck
i. Pickup ii. Semi
Do NOT create fields or methods other than those prescribed in the lab. You may use additional local variables.
Use the exact method names and field names as specified
Manipulate the toString methods so they give the correct output
Use super in your sub-class constructors watch the parameters, they must be exactly as specified
Create the following classes:
1. Auto: a. Import DecimalFormat
b. Fields:
Final: doors (# of doors), wheels (# of wheels), mpg, tank (tank capacity), SN (serial number)
Private: mileage, fuel (gallons of fuel in tank)
Private Static: numAutos (set to zero)
c. Methods:
Auto(int doors, int wheels, double pmpg, double ptank)
Initialize all final fields
Zero mileage
Full tank
Increment numAutos
Set SN to numAutos
Getter and setter for mileage need setter for Truck.drive
Getter and Setter for fuel need setter for Truck.drive
double drive(double miles)
double pump(double gallons)
toString
public String toString() {
DecimalFormat df = new DecimalFormat("#.#");
String s = "SN: "+SN+", "; s += doors+" doors, "; s += wheels+" wheels, "; s += df.format(mpg)+" mpg, "; s += df.format(fuel)+"/"+df.format(tank)+" fuel, "; s += df.format(mileage)+" mileage";
return s; }
vii. equals
public boolean equals(Object o) { if (o instanceof Auto) {
Auto a = (Auto)o;
return this.doors == a.doors && this.wheels == a.wheels && this.mpg == a.mpg && this.tank == a.tank;
} else { return false;
} }
2. Car always 4 wheels: a. Fields:
Final: passCap
Private: passengers
b. Methods:
Car(int passCap, int doors, double pmpg, double ptank)
Call super constructor
Initialize final fields
Zero passengers
Getter for passenger
int embark(int persons)
int disembark(int persons)
toString see output below
equals
public boolean equals(Object o) {
if (o instanceof Car) { Car c= (Car)o;
return this.passCap == c.passCap && super.equals(c); } else {
return false; }
}
3. Sedan always 4 doors and always 5 passCap a. Constructor only
4. Coupe always 2 doors and always 4 passCap a. Constructor only
5. Truck a. Import DecimalFormat
b. Fields:
Final: mpgPerTon
Private: cargo
c. Methods:
Truck(double mpgPerTon, int doors, int wheels, double pmpg, double ptank)
Call super constructor
Initialize final fields
Zero cargo
Getter for cargo
double load(double payload)
double unload(double payload)
Override double drive(double miles)
double mpgActual = mpg + mpgPerTon * cargo;
if mpgActual <= 0; truck is too heavy; set fuel to 0 and return 0;
else change mileage and fuel as in Auto.drive, except use mpgActual instead of mpg in
calculations
NOTE: You must use getters and setters
toString
public String toString() { DecimalFormat df = new DecimalFormat("#.#");
String s = "Truck, "; s += super.toString()+", "; s += df.format(mpgPerTon)+" mpg/ton, ";
s += df.format(cargo)+" cargo";
return s; }
vii. equals
public boolean equals(Object o) { if (o instanceof Truck) {
Truck t= (Truck)o;
return this.mpgPerTon == t.mpgPerTon && super.equals(t); } else {
return false; }
}
6. Pickup:
Constructor
toString see output below
equals this only calls super.equals
7. Semi:
Constructor
toString see output below
equals this only calls super.equals
8. TestAuto:
public static void main(String[] args)
Declare and new ArrayList of Auto called autos
Add the following to autos:
Coupe(20, 10) Coupe(20, 10) Coupe(25, 11) Sedan(20,12) Sedan(25,12) Sedan(20,12) Pickup(-5, 2, 4, 16, 12) Pickup(-6, 2, 6, 20, 16) Semi(-0.5, 2, 18, 8, 40) Semi(-0.2, 4, 18, 6, 50)
Print Initial autos
Print all autos see output
Print Embark, Load, Drive
Enhanced for loop through all autos
If it is a car, embark 10 passengers
If it is a pickup, load 3.2 tons
If it is a semi, load 15 tons
NOTE: you must cast to the right type
Drive all autos 100 miles
Print all autos
Print Disembark, unload, pump
Enhanced for loop through all autos
If it is a car, disembark 5 passengers
If it is a truck, unload 10 tons
NOTE: you must cast to the right type
Pump 30 gallons into all autos
Print all autos
Sample output
Initial autos
[Car, SN: 1, 2 doors, 4 wheels, 20 mpg, 10/10 fuel, 0 mileage, 0/4 passengers] [Car, SN: 2, 2 doors, 4 wheels, 20 mpg, 10/10 fuel, 0 mileage, 0/4 passengers] [Car, SN: 3, 2 doors, 4 wheels, 25 mpg, 11/11 fuel, 0 mileage, 0/4 passengers] [Car, SN: 4, 4 doors, 4 wheels, 20 mpg, 12/12 fuel, 0 mileage, 0/5 passengers] [Car, SN: 5, 4 doors, 4 wheels, 25 mpg, 12/12 fuel, 0 mileage, 0/5 passengers] [Car, SN: 6, 4 doors, 4 wheels, 20 mpg, 12/12 fuel, 0 mileage, 0/5 passengers] [Pickup Truck, SN: 7, 2 doors, 4 wheels, 16 mpg, 12/12 fuel, 0 mileage, -5 mpg/ton, 0 cargo]
[Pickup Truck, SN: 8, 2 doors, 6 wheels, 20 mpg, 16/16 fuel, 0 mileage, -6 mpg/ton, 0 cargo] [Semi Truck, SN: 9, 2 doors, 18 wheels, 8 mpg, 40/40 fuel, 0 mileage, -0.5 mpg/ton, 0 cargo]
[Semi Truck, SN: 10, 4 doors, 18 wheels, 6 mpg, 50/50 fuel, 0 mileage, -0.2 mpg/ton, 0 cargo]
Embark, Load, Drive [Car, SN: 1, 2 doors, 4 wheels, 20 mpg, 5/10 fuel, 100 mileage, 4/4 passengers] [Car, SN: 2, 2 doors, 4 wheels, 20 mpg, 5/10 fuel, 100 mileage, 4/4 passengers] [Car, SN: 3, 2 doors, 4 wheels, 25 mpg, 7/11 fuel, 100 mileage, 4/4 passengers] [Car, SN: 4, 4 doors, 4 wheels, 20 mpg, 7/12 fuel, 100 mileage, 5/5 passengers] [Car, SN: 5, 4 doors, 4 wheels, 25 mpg, 8/12 fuel, 100 mileage, 5/5 passengers] [Car, SN: 6, 4 doors, 4 wheels, 20 mpg, 7/12 fuel, 100 mileage, 5/5 passengers] [Pickup Truck, SN: 7, 2 doors, 4 wheels, 16 mpg, 0/12 fuel, 0 mileage, -5 mpg/ton, 3.2 cargo] [Pickup Truck, SN: 8, 2 doors, 6 wheels, 20 mpg, 0/16 fuel, 12.8 mileage, -6 mpg/ton, 3.2 cargo] [Semi Truck, SN: 9, 2 doors, 18 wheels, 8 mpg, 0/40 fuel, 20 mileage, -0.5 mpg/ton, 15 cargo] [Semi Truck, SN: 10, 4 doors, 18 wheels, 6 mpg, 16.7/50 fuel, 100 mileage, -0.2 mpg/ton, 15 cargo]
Disembark, unload, pump [Car, SN: 1, 2 doors, 4 [Car, SN: 2, 2 doors, 4 [Car, SN: 3, 2 doors, 4 [Car, SN: 4, 4 doors, 4 [Car, SN: 5, 4 doors, 4 [Car, SN: 6, 4 doors, 4 [Pickup Truck, SN: 7, 2 cargo]
[Pickup Truck, SN: 8, 2 cargo] [Semi Truck, SN: 9, 2 doors, 18 wheels, 8 mpg, 30/40 fuel, 20 mileage, -0.5 mpg/ton, 5 cargo] [Semi Truck, SN: 10, 4 doors, 18 wheels, 6 mpg, 46.7/50 fuel, 100 mileage, -0.2 mpg/ton, 5 cargo]
Step by Step Solution
There are 3 Steps involved in it
Step: 1
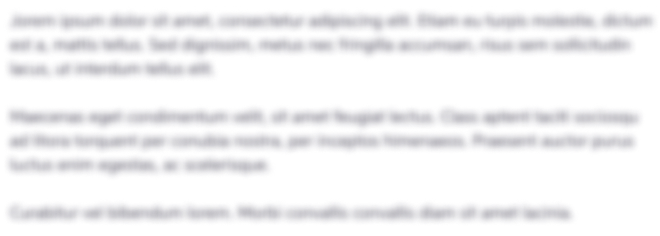
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started