Question
**In Java with detailed comments** public class LinkedList3 { private class Node { private T data; private Node link; public Node( ) { data =
**In Java with detailed comments**
public class LinkedList3
private class Node
{
private T data;
private Node
public Node( )
{
data = null;
link = null;
}
public Node(T newData, Node
{
}//End of node
private Node
public LinkedList3( )
{
head = null;
}
/**
Adds a node at the start of the list with the specified data. The added node will be the first node in the list.
*/
public void addTostart (T itemData)
{
head = new Node
}
/**
Removes the head node and returns true if the list contains at least one node. Returns false if the list is empty.protected Object clone() throws CloneNotSupportedException {};
*/
public boolean deleteHeadNode ( )
{
if (head != null)
{
head = head.link;
return true;
}
else
return false;
}
/**
* Returns the number of nodes in the list.
*/
public int size( )
{
int count = 0;
Node
while (position !=null)
{
count++;
position = position.link;
}
return count;
}
public boolean contains(T item)
{
return (find(item) !=null);
}
/**
* Finds the first node containing the target item, and returns a reference to that node. If target is not in the list, null is returned.
*/
private Node
{
Node
T itemAtPosition;
while (position != null)
{
itemAtPosition = position.data;
if (itemAtPosition.equals(target))
return position;
position = position.link;
}
}
public boolean isEmpty( )
{
return (head == null);
}
public void clear ( )
{
head = null;
}
public void clear ( )
{
head = null;
}
/*
* For two lists to be equal they must contain the same data items in the same order. The equals method of T is used to compare data items.
* */
public boolean equals(Object otherObject)
{
if (otherObject == null)
return false;
else if (getClass ( ) != otherObject.getClass( ))
return false;
else
{
LinkedList3
if (size( ) != otherList.size( )}
return false;
Node
Node
while (position != null)
{
if (!(position.data.equals(otherPostiion.data))}
return false;
position = position.link;
otherPosition = otherPosition.link;
}
return true; /o mismatch was found
}
}
}
**In Java with detailed comments**
Assignment 10: Take the code (it is from the Savitch student data files) listed here and 1) make it doubly linked. 2) Add a copy constructor 3) Add a clone method 4) Replace outputList with toString 5) Code an iterator method 6) Keep track of the tail and add a method outputBackwards to prove the list is doubly linked. Or use the Savitch code in the book for a doubly linked list and add the functionality mentioned above - I think you will learn less that way but it is less work. To make the node doubly linked change to private NodeStep by Step Solution
There are 3 Steps involved in it
Step: 1
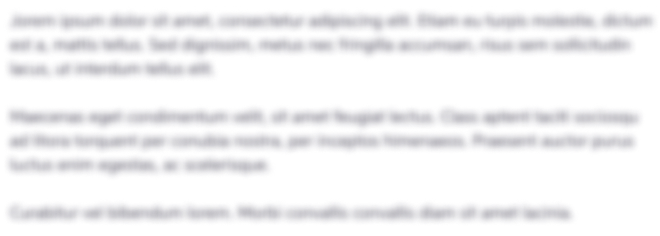
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started