Question
In Java, write a bunch of classes that will draw a bunch of lines! Dont worry you dont have to write any graphics, as that
In Java, write a bunch of classes that will draw a bunch of lines! Dont worry you dont have to write any graphics, as that part is provided in the driver. Each line is drawn based on a math function that takes in a given x coordinate and will return its y coordinate.
First download the driver and put it in your project
DO NOT ALTER THE DRIVER!
Write an interface called Line
Create the following method definition
getYPoint: This takes in a decimal value and returns a decimal value depending on the type of line.
Write a class called SlopedLine
This should implement Line Instance variable
slope: a decimal value corresponding to the lines slope
Create the following Constructors
Default Parameterized Constructor
Accessors and Mutators for each variable Create the following Methods
getYPoint this method takes in a decimal value corresponding to a x-coordinate and returns the y-coordinate based on the slope equation (y = slope*x)
Write a class called ExponentialLine
This should implement Line Instance variable
exponent: a decimal value corresponding to the lines exponent
Create the following Constructors
Default Parameterized Constructor
Accessors and Mutators for each variable Create the following Methods
getYPoint this method takes in a decimal value corresponding to a x-coordinate and returns the y-coordinate based on the slope equation (y = x^exponent)
Write a class called SineLine
This should implement Line Instance variable
amplitude: a decimal value corresponding to the lines amplitude frequency: a decimal value corresponding to the lines frequency
Create the following Constructors
Default Parameterized Constructor
Accessors and Mutators for each variable Create the following Methods
getYPoint this method takes in a decimal value corresponding to a x-coordinate and returns the y-coordinate based on a sine wave equation (y = amplitude*sin(x*frequency))
Write a class called SawLine
This should implement Line Instance variable
modValue: a decimal value corresponding to the modulo peak of the wave
Create the following Constructors
Default Parameterized Constructor
Accessors and Mutators for each variable Create the following Methods
getYPoint this method takes in a decimal value corresponding to a x-coordinate and returns the y-coordinate based on the equation (y = x mod modValue)
Write a class called StaircaseLine
This should implement Line Instance variable
width: a decimal value corresponding to the stairs width height: a decimal value corresponding to the stairs height
Create the following Constructors
Default Parameterized Constructor
Accessors and Mutators for each variable Create the following Methods
getYPoint this method takes in a decimal value corresponding to a x-coordinate and returns the y-coordinate based the width and height. HINT(using integer division and multiplying that by the height will achieve the effect).
HINT: If the lines are looking weird it may be a good idea to print out each of the coordinates and observing what is going on in the method getYPoint
//driver
import java.applet.*; //Needed to create the window import java.awt.*; //Needed for drawing import java.util.*; public class DrawingLines extends Applet{//When it extends applet it creates a window and calls certain methods private Image display; //Used as the flat image private Graphics drawingArea; //Used to draw item in the image /* *This is called by the parent Applet, as its an overridden method, and it initializes all of the instance variables. * Think of this as a variation of a constructor, but called by another, hidden piece of code */ public void init() { //get the height and width from the Applet int height = getSize().height; int width = getSize().width; //Creates an image using the height and width in the applet display = createImage(width,height); //Sets up the drawing area for the image above to be drawn on drawingArea = display.getGraphics(); //This draws the lines drawLines(drawingArea); } /* * This is also called by * the applet as it is an overridden method. */ public void paint(Graphics g) { g.drawImage(display,0,0,null); } /* * This Draws line! */ public void drawLines(Graphics g) { Color[] colors = {Color.black,Color.blue,Color.red,Color.green,Color.darkGray}; Line[] lines = new Line[5]; lines[0] = new SlopedLine(1); lines[1] = new SineLine(100,0.25); lines[2] = new ExponentialLine(1.25); lines[3] = new StaircaseLine(20,20); lines[4] = new SawLine(50); final int X_POINTS = 1000; for(int i=0;i
Step by Step Solution
There are 3 Steps involved in it
Step: 1
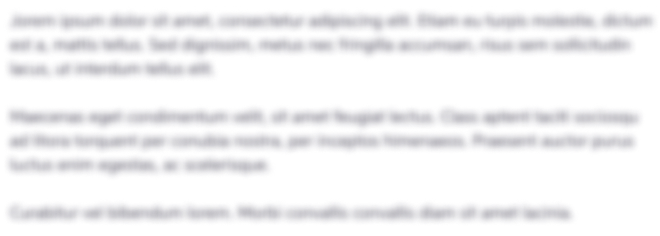
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started