Question
IN JAVA Write a program to take a float value from the user that represents a monetary amount, such as 17.89, and print back out
IN JAVA
Write a program to take a float value from the user that represents a monetary amount, such as 17.89, and print back out the least number of each type of US bill and coin needed to represent that amount, starting with the highest. As a simplification, assume that the ten-dollar bill is the maximum size bill that you have to work with.
For example, if the user enters 47.63, your program should print:
4 ten dollar bills 1 five dollar bill 2 one dallar bills 2 quarters 1 dime 0 nickels 3 pennies
You must use a read-until-sentinel loop pattern to keep asking the user if they wish to enter a new value (Y/N). Your program will always complete one calculation as above before asking whether they want to try again.
Note that there are no coins for fractions of a cent. You can give somebody $3.50, but not $3.501. For this assignment, you may assume that the user always enters a valid amount of money.
Note: Floating point numbers are approximations, and subject to surprising rounding errors. Because of this, you should never use floating point types to perform monetary calculations that need to be precise not in this assignment, not ever! Instead, for this assignment, represent money using an int that records the number of cents. For example, a dollar would be represented as 100, and $3.50 would be represented as 350.
Hints:
Pay close attention to the singulars and plurals in the output above. Study each line.
Be sure to round properly when you convert from floating point types to integer types. For example, 1.05 represented as a float comes out to 1.04999995. Does your code give the correct answer for numbers like this?
Javas Scanner class has some surprising behavior. If you encounter something puzzling or vexing, ask your preceptors and instructor for a hint!
Testing your program: Because this program takes its input straight from the user typing and prints its output straight back to the screen, it does not lend itself to unit testing. Instead, you will need to use manual interactive testing. Test out your program by trying various amounts to insure that it is correct. Be sure that you try enough cases to ensure that all parts of your code solution execute. This means that you choose examples so that all parts of conditional if statements get tried and the possible range of amounts for each bill and coin get used.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
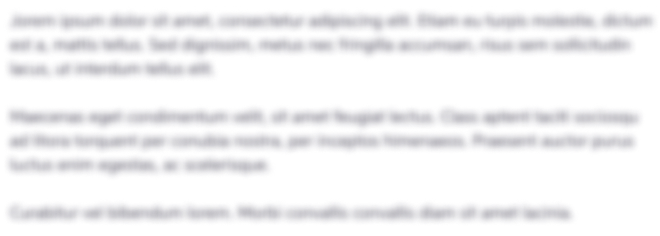
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started