Question
IN JAVA You have been asked to create a program for ABC Home Improvement Co. to create statements for their credit card processing system. The
IN JAVA
You have been asked to create a program for ABC Home Improvement Co. to create statements for their credit card processing system. The program is to process a set of transactions (i.e., charges, payments, credits and finance charges) against a set of accounts and produce a statement for each account. Input to the program will be two files one containing a list of accounts and the other a list of transactions. Both are CSV files. Output will be a report to standard out. The format of each file is as follows:
Your program must pass the file names on the command line. In the event the files are not passed on the command line, then print to System.err a Usage statement and exit. Using those files, the program will process the transactions then print the statement report. A sample output of the statement report is as follows:
Additional Specifications:
- Do not print a statement for account that have zero balance and no activity (no transactinons).
- An interest transaction is to be generated as the last transaction. An interest transaction is only generated if the total payments is less than the beginning balance. The interest will be computed as the average of the beginning balance and ending balance times a monthly interest rate.
- In a main class define a static variable for annual percentage rage (APR). The monthly interest rate used in calculating the interest is one twelfth of the APR.
- Use a java.time.LocalDate for all date data.
- In the main class define a static variable of type java.time.format.DateTimeFormatter and initialize it using the ofPattern method using the specified format. Use this DateTimeFormatter when parsing input date and when printing a date.
- In the main class define a static method getStatementDate which returns a date of 1/31/2022. Call this method when printing the statement date and on generating an Interest transaction.
- In addition to the fields for a transaction, include a balance field. This field is to hold the balance after a transaction has been applied to the account.
- When applying a transaction to the account, increment the account balance for a charge and interest transactions, decrement the account balance for payment and return transactions.
- Do not do any calculations in the methods to print a statement, all calculations are to be performed prior the printing.
- Use ABC HI Credit as the source for the generated interest transaction.
Accounts.txt
1234123400,John Smith,0 3828382810,Mary Jones,387.45 8833992012,Tom Green,540.45 8430200101,Sue Black,0 8293100112,David Williams,3422.12 8877283919,Jill Thompson,34.12
Transactions.txt
1234123400,C,#3 Indiana,1/01/2022,2.12 3828382810,P,Cash,1/01/2022,387.45 8833992012,P,Visa **4453,1/02/2022,100.00 8293100112,C,#3 Indiana,1/02/2022,53.61 1234123400,C,#3 Indiana,1/03/2022,6.34 8877283919,C,#5 Greensburg,1/03/2022,112.50 8293100112,C,#3 Indiana,1/04/2022,153.23 8293100112,R,#3 Indiana,1/04/2022,53.61 8293100112,P,Check 4301,1/04/2022,1000.00 1234123400,C,#3 Indiana,1/10/2022,43.89 8293100112,C,#3 Indiana,1/14/2022,31.34 1234123400,C,#3 Indiana,1/16/2022,9.53 1234123400,C,#3 Indiana,1/17/2022,32.12 8293100112,R,#3 Indiana,1/18/2022,5.12 1234123400,C,#3 Indiana,1/21/2022,98.75
Accounts.txt a text file containing a set of records in a csv (no header) format. Each record represents an account. The format of a record is: Fields Account number Name Balance Description 10 Digits String of characters Digits with decimal point and two fractional digits Transactions.txt a text file containing a set of records in a csv (no header) format. Each record represents a transaction. The format of a record is: Fields Account number Type Description 10 Digits 1 character: C for a Charge P for a Payment R for a Return I for Interest (note not in the file) A description as to the source of the transaction A date in M/dd/yyyy format Digits with decimal point and two fractional digits Source Date Amount A B C Home Improvement Co. Statement for 1/31/2022 Account Name: David Williams Account: 8293100112 Beginning Balance: $ 3, 422.12 Ending Balance: $ 2,639.22 Purchases: Payments: Returns : Finance Charge: u ) ) ) 238.18 $ 1,000.00 $ 58.73 37.65 Date 1/02/2022 1/04/2022 1/04/2022 1/04/2022 1/14/2022 1/18/2022 1/31/2022 Type Source Charge #3 Indiana Charge #3 Indiana Return #3 Indiana Payment Check 4301 Charge #3 Indiana Return #3 Indiana Interest ABC HI Credit Amount S 53.61 $ 153.23 $ 53.61 $ 1,000.00 $ 31.34 $ 5.12 $ 37.65 Balance $ 3,475.73 $ 3,628.96 $ 3, 575.35 $ 2,575.35 $ 2,606.69 $ 2,601.57 $ 2,639.22 A B C Home Improvement Co. Statement for 1/31/2022 $ Account Name: Jill Thompson Account: 8877283919 Beginning Balance: $ 34.12 Ending Balance: $ 147.75 Purchases: Payments: Returns: Finance Charge: 4) ) ) ) 112.50 0.00 0.00 1.13 S $ Date 1/03/2022 1/31/2022 Type Charge Interest Source #5 Greensburg ABC HI Credit S $ Amount 112.50 1.13 016 Balance 146.62 147.75 Accounts.txt a text file containing a set of records in a csv (no header) format. Each record represents an account. The format of a record is: Fields Account number Name Balance Description 10 Digits String of characters Digits with decimal point and two fractional digits Transactions.txt a text file containing a set of records in a csv (no header) format. Each record represents a transaction. The format of a record is: Fields Account number Type Description 10 Digits 1 character: C for a Charge P for a Payment R for a Return I for Interest (note not in the file) A description as to the source of the transaction A date in M/dd/yyyy format Digits with decimal point and two fractional digits Source Date Amount A B C Home Improvement Co. Statement for 1/31/2022 Account Name: David Williams Account: 8293100112 Beginning Balance: $ 3, 422.12 Ending Balance: $ 2,639.22 Purchases: Payments: Returns : Finance Charge: u ) ) ) 238.18 $ 1,000.00 $ 58.73 37.65 Date 1/02/2022 1/04/2022 1/04/2022 1/04/2022 1/14/2022 1/18/2022 1/31/2022 Type Source Charge #3 Indiana Charge #3 Indiana Return #3 Indiana Payment Check 4301 Charge #3 Indiana Return #3 Indiana Interest ABC HI Credit Amount S 53.61 $ 153.23 $ 53.61 $ 1,000.00 $ 31.34 $ 5.12 $ 37.65 Balance $ 3,475.73 $ 3,628.96 $ 3, 575.35 $ 2,575.35 $ 2,606.69 $ 2,601.57 $ 2,639.22 A B C Home Improvement Co. Statement for 1/31/2022 $ Account Name: Jill Thompson Account: 8877283919 Beginning Balance: $ 34.12 Ending Balance: $ 147.75 Purchases: Payments: Returns: Finance Charge: 4) ) ) ) 112.50 0.00 0.00 1.13 S $ Date 1/03/2022 1/31/2022 Type Charge Interest Source #5 Greensburg ABC HI Credit S $ Amount 112.50 1.13 016 Balance 146.62 147.75
Step by Step Solution
There are 3 Steps involved in it
Step: 1
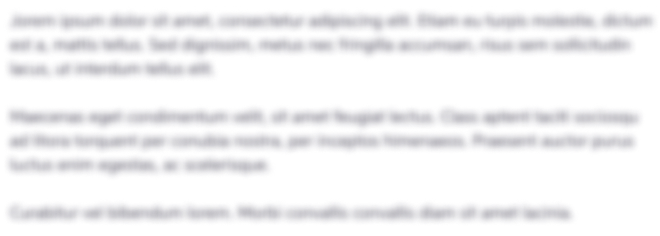
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started