Question
In Java, you will be simulating a building that contains a single elevator. However, this elevator is somewhat simplified: it only moves a person to
In Java, you will be simulating a building that contains a single elevator. However, this elevator is somewhat simplified: it only moves a person to a floor and then the person will remain on that floor forever. That is, the elevator cannot pick people up from any floor besides the lobby. People will be entered into a building going to a specific floor. Two things need to happen. As a person arrives at the building, the elevator may or may not be in the lobby. To pick them up, the elevator (1) must be sent to the lobby to pick them up and then (2) bring them to their floor.
What to code:
Person.java
public Person(String firstName, String lastName) This will construct a Person with a given first and last name.
public boolean enterBuilding(Building building, int floor) This method will enter this Person into a Buildings Elevator with a provided destination floor number. This should return true if the Person can be brought by the elevator to floor and false if not. You may assume a Person will only ever try to enter one Building.
public String getLocation() This should return this Persons location as a String. To do this, you should maintain a field that tracks this Persons location in some way. When this method is called it should return the following exact Strings: o
1. Waiting to be serviced from the time this Person is validated by a Building (to be processed) to the time they arrive on their desired Floor.
2. In Lobby if this Person is in a Buildings lobby and will never be serviced by the Buildings Elevator.
3. In Floor x if this Person is on Floor number x.
Building class:
package main;
public class Building {
private Floor[] floors;
private Elevator elevator;
public Building(int numFloors) { //This constructs a Building that has some number of floors. When you construct the Building, its Elevator should be at the lobby.
floors = new Floor[10];
startFloor();
elevator = new Elevator(this);
}
public void startFloor() {
for (int i = 0; i < floors.length; i++) {
floors[i] = new Floor();
}
}
public boolean enterElevatorRequest(Person person, int floor) { //public boolean enterElevatorRequest(Person person, int floor) This method will handle the request made by a Person to enter this Building and be taken to some floor. This will involve ensuring that the Persons desired floor can be reached by this Buildings Elevator. If the Elevator cannot reach the Persons desired floor, return false. If the elevator can reach the persons desired floor, return true. This method should ensure that the Elevator will never process this Persons request if it is not valid.
elevator.createJob(person, floor);
elevator.goToLobbyJob();
person.setFloor(-1);
System.out.println(person.getLocation());
return true;
}
public void startElevator() { public void startElevator() This will call a method in this Buildings Elevator instance that should process all of its current Jobs.
elevator.processAllJobs();
}
public boolean enterFloor(Person person, int floor) { public void enterFloor(Person person, int floor) This method should save a reference of a Person in the Floor with the provided floor number.
floors[floor].enterFloor(person);
person.setFloor(floor);
return true;
}
public Floor[] getFloor() {
return floors;
}
public Elevator getElevator() {
return elevator;
}
public String toString() {
String people = "";
int i = 0;
while (i < floors.length) {
if (!floors[i].toString().equals("")) {
people = people + floors[i].toString() + " " + i + ".";
}
i++;
}
return people;
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
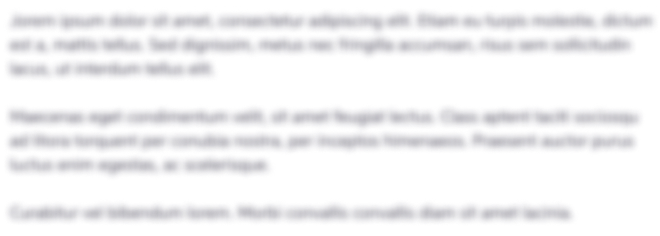
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started