Question
in main cpp Create a separate list for each data type C++ language Floating Point Link List Example // This program demonstrates the deleteNode member
in main cpp Create a separate list for each data type
C++ language
Floating Point Link List Example
// This program demonstrates the deleteNode member function
#include
#include
#include "FloatList.h"
using namespace std;
char menu()
{ char ch;
cout<<"\t\tWhat Operation would like to perform ? ";
cout<<"\t\t\tC. Create a List ";
cout<<"\t\t\tD. Delete a List ";
cout<<"\t\t\tI. Insert a List ";
cout<<"\t\t\tS. Show a List ";
cout<<"\t\t\tQ. Quit the Program ";
cout<<"\t\tEnter your choice ==> ";
cin>>ch;
return ch;
}
int main()
{ FloatList list;
float val;
char choice;
choice = menu();
while (choice !='Q' && choice != 'q')
{ system("cls");
switch(choice) {
case 'C': case 'c':
// Build the list
list.appendNode(2.5);
list.appendNode(7.9);
list.appendNode(12.6);
cout << "Here are the initial values: ";
list.displayList();
cout << endl;
break;
case 'D': case 'd':
cout<<" Here is the contents of the current list ";
list.displayList();
cout<<" Enter your value to be destroyed ..";
cin>>val;
list.deleteNode(val);
cout << " Here are the nodes left. ";
list.displayList();
break;
case 'I': case 'i':
cout<<" Enter your value to be inserted ..";
cin>>val;
list.insertNode(val);
cout << " Here are the new nodes. ";
list.displayList();
break;
case 'S': case 's':
cout<<" Here are the list of nodes .... ";
list.displayList();
}
choice = menu();
}
cout<<" Here are the left over nodes before quitting ... ";
list.displayList();
cout< return 0; } Linklist header File: // Specification file for the FloatList class #ifndef FLOATLIST_H #define FLOATLIST_H class FloatList { private: // Declare a structure for the list struct ListNode { float value; struct ListNode *next; }; ListNode *head; // List head pointer public: FloatList() // Constructor { head = NULL; } ~FloatList(); // Destructor void appendNode(float); void insertNode(float); void deleteNode(float); void displayList(); }; #endif LinkList CPP File: // Implementation file for the FloatList class #include #include "FloatList.h" using namespace std; //************************************************** // appendNode appends a node containing the * // value pased into num, to the end of the list. * //************************************************** void FloatList::appendNode(float num) { ListNode *newNode, *nodePtr; // Allocate a new node & store num newNode = new ListNode; newNode->value = num; newNode->next = NULL; // If there are no nodes in the list // make newNode the first node if (!head) head = newNode; else // Otherwise, insert newNode at end { // Initialize nodePtr to head of list nodePtr = head; // Find the last node in the list while (nodePtr->next) nodePtr = nodePtr->next; // Insert newNode as the last node nodePtr->next = newNode; } } //************************************************** // displayList shows the value * // stored in each node of the linked list * // pointed to by head. * //************************************************** void FloatList::displayList() { ListNode *nodePtr; nodePtr = head; while (nodePtr!= NULL) { cout << nodePtr->value << endl; nodePtr = nodePtr->next; } } //************************************************** // The insertNode function inserts a node with * // num copied to its value member. * //************************************************** void FloatList::insertNode(float num) { ListNode *newNode, *nodePtr, *previousNode = NULL; // Allocate a new node & store num newNode = new ListNode; newNode->value = num; // If there are no nodes in the list // make newNode the first node if (!head) { head = newNode; newNode->next = NULL; } else // Otherwise, insert newNode { // Initialize nodePtr to head of list and previousNode to NULL. nodePtr = head; previousNode = NULL; // Skip all nodes whose value member is less // than num. while (nodePtr != NULL && nodePtr->value < num) { previousNode = nodePtr; nodePtr = nodePtr->next; } // If the new node is to be the 1st in the list, // insert it before all other nodes. if (previousNode == NULL) { head = newNode; newNode->next = nodePtr; } else // Otherwise, insert it after the prev. node. { previousNode->next = newNode; newNode->next = nodePtr; } } } //************************************************** // The deleteNode function searches for a node * // with num as its value. The node, if found, is * // deleted from the list and from memory. * //************************************************** void FloatList::deleteNode(float num) { ListNode *nodePtr, *previousNode; // If the list is empty, do nothing. if (!head) return; // Determine if the first node is the one. if (head->value == num) { nodePtr = head->next; delete head; head = nodePtr; } else { // Initialize nodePtr to head of list nodePtr = head; // Skip all nodes whose value member is // not equal to num. while (nodePtr != NULL && nodePtr->value != num) { previousNode = nodePtr; nodePtr = nodePtr->next; } // If nodePtr is not at the end of the list, // link the previous node to the node after // nodePtr, then delete nodePtr. if (nodePtr) { previousNode->next = nodePtr->next; delete nodePtr; } } } //************************************************** // Destructor * // This function deletes every node in the list. * //************************************************** FloatList::~FloatList() { ListNode *nodePtr, *nextNode; nodePtr = head; while (nodePtr != NULL) { nextNode = nodePtr->next; delete nodePtr; nodePtr = nextNode; } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
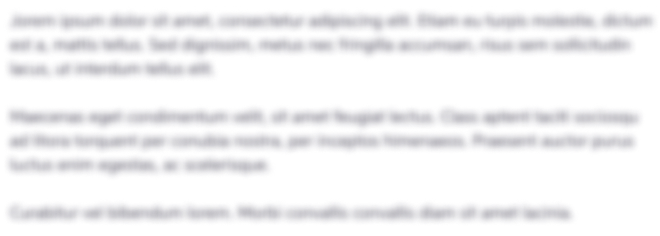
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started