Question
IN ORIGINAL JAVA CODE: 1. Create the following interface interface Fixable { // turns Power on, fix window and zeros out error codes void fix
IN ORIGINAL JAVA CODE:
1. Create the following interface interface Fixable { // turns Power on, fix window and zeros out error codes void fix (); // logs to a text file in the current directory called fix.log // prints to the console, and identify time and nature of // the fix void log(); } 2. Create inner classes PowerOn and FixWindow that implement Fixable. 3. Add a method to GreenhouseControls int getError(); which returns the error code saved. 4. Add a method within GreenhouseControls Fixable getFixable(int errorcode); which returns the appropriate Fixable object to correct the error and reset the error code to zero. 5. Create a class Restore to restore the system after a shutdown. When this command line is called: java GreenhouseControls -d dump.out a Restore object should be created and the file name dump.out is passed to the Restore object. It then retrieves the GreenhouseControls object saved by any emergency shutdown. It has the state of the saved system printed to the console. It sets any variable that was changed by the Event that initiated the shutdown back to its normal state (e.g., poweron=true) using the appropriate Fixable. It then starts the system running at the event following the event that caused the shutdown. A proper error message should be displayed on the console if any exception or error is caught by the Restore object.
************************************CODE*******************************************
package tme3; import java.text.DateFormat; import java.text.SimpleDateFormat; import java.util.Date; import java.util.Scanner; public class GreenhouseControls extends Controller { private boolean light = false; private boolean water = false; private String thermostat = "Day"; private String eventsFile = "example1.txt"; // 3. Add an instance variable in GreenhouseControls called error code. private int errorCode; public class LightOn extends Event { public LightOn(long delayTime) { super(delayTime); } public void action() { // Put hardware control code here to // physically turn on the light. light = true; } public String toString() { return "Light is on"; } } public class LightOff extends Event { public LightOff(long delayTime) { super(delayTime); } public void action() { // Put hardware control code here to // physically turn off the light. light = false; } public String toString() { return "Light is off"; } } public class WaterOn extends Event { public WaterOn(long delayTime) { super(delayTime); } public void action() { // Put hardware control code here. water = true; } public String toString() { return "Greenhouse water is on"; } } public class WaterOff extends Event { public WaterOff(long delayTime) { super(delayTime); } public void action() { // Put hardware control code here. water = false; } public String toString() { return "Greenhouse water is off"; } } public class ThermostatNight extends Event { public ThermostatNight(long delayTime) { super(delayTime); } public void action() { // Put hardware control code here. thermostat = "Night"; } public String toString() { return "Thermostat on night setting"; } } public class ThermostatDay extends Event { public ThermostatDay(long delayTime) { super(delayTime); } public void action() { // Put hardware control code here. thermostat = "Day"; } public String toString() { return "Thermostat on day setting"; } } // An example of an action() that inserts a // new one of itself into the event list: public class Bell extends Event { public Bell(long delayTime) { super(delayTime); } public void action() { addEvent(new Bell(delayTime)); } public String toString() { return "Bing!"; } } private boolean fans; public class FansOn extends Event { public FansOn(long delayTime) { super(delayTime); } public void action() { fans = true; } public String toString() { return "Fans are on"; } } public class FansOff extends Event { public FansOff(long delayTime) { super(delayTime); } public void action() { fans = false; } public String toString() { return "Fans are off"; } } private boolean windowork; public class WinidowMalfunction extends Event { public WinidowMalfunction(long delayTime) { super(delayTime); } // 1. After setting the variables, WindowMalfunction or PowerOut should throw an // exception specifying the faulty condition public void action() throws ControllerException { windowork = false; // 3. error code in an int variable error code -> 1 for WindowMalfunction someThingWentWrongHere(1, "Error at WinidowMalfunction"); } } private boolean poweron; public class PowerOut extends Event { public PowerOut(long delayTime) { super(delayTime); } // 1. After setting the variables, WindowMalfunction or PowerOut should throw an // exception specifying the faulty condition public void action() throws ControllerException { poweron = false; // 3. error code in an int variable error code -> 2 for powerOut someThingWentWrongHere(2, "Error at powerOut event"); } } void someThingWentWrongHere(int errorCode, String errorMessage) throws ControllerException { this.errorCode = errorCode; throw new ControllerException(errorMessage); } public class ControllerException extends Exception { public ControllerException(String except) { super(except); } public String getMessage() { return super.getMessage(); } public void shutdown() { } } public class Restart extends Event { public Restart(long delayTime, String filename) { super(delayTime); eventsFile = filename; } public void action() { Scanner scanner = new Scanner(eventsFile); while (scanner.hasNextLine()) { String line = scanner.nextLine(); } addEvent(new ThermostatNight(0)); addEvent(new LightOn(2000)); addEvent(new WaterOff(8000)); addEvent(new ThermostatDay(10000)); addEvent(new Bell(2000)); addEvent(new WaterOn(6000)); addEvent(new LightOff(4000)); addEvent(new Terminate(12000)); addEvent(new FansOn(5000)); addEvent(new FansOff(1000)); } public String toString() { return "Restarting system"; } } public class Terminate extends Event { public Terminate(long delayTime) { super(delayTime); } public void action() { System.exit(0); } public String toString() { return "Terminating"; } } public static void printUsage() { System.out.println("Correct format: "); System.out.println(" java GreenhouseControls -f, or"); System.out.println(" java GreenhouseControls -d dump.out"); } // --------------------------------------------------------- public static void main(String[] args) { GreenhouseControls gc = new GreenhouseControls(); try { /* * String option = args[0]; String filename = args[1]; * * if (!(option.equals("-f")) && !(option.equals("-d"))) { * System.out.println("Invalid option"); printUsage(); } * * if (option.equals("-f")) { gc.addEvent(gc.new Restart(0, filename)); } */ // UnCommenting above code will never test below code because above code with // throw first exception and it will never execute below code // To test PowerOut fail with exception // gc.addEvent(gc.new PowerOut(100)); // To test WinidowMalfunction fail with exception gc.addEvent(gc.new WinidowMalfunction(100)); gc.run(); } catch (ArrayIndexOutOfBoundsException e) { System.out.println("Invalid number of parameters"); printUsage(); } // 2. the Controller catches the exception catch (ControllerException e) { // 2. then initiates an emergency shutdown with an appropriate message gc.shutdown(e.getMessage()); } } // override this method in GreenhouseControls to accomplish the shutdown. @Override protected void shutdown(String message) { // super.shutdown(); DateFormat df = new SimpleDateFormat("dd/MM/yy HH:mm:ss"); Date dateobj = new Date(); // logs the time and the reason for the shutdown in a text file ,, Here you will // create file and log it System.out.println("Shutdown due to Error: " + message + " | Error Code:" + errorCode + " | DateTime:" + df.format(dateobj)); } /* * enum ErrorCode { WindowMalfunction, PowerOut } */ }
public class Controller { // A class from java.util to hold Event objects: private ListeventList = new ArrayList (); public void addEvent(Event c) { eventList.add(c); } public void run() throws ControllerException{ while (eventList.size() > 0) // Make a copy so you're not modifying the list // while you're selecting the elements in it: for (Event e : new ArrayList (eventList)) if (e.ready()) { System.out.println(e); e.action(); eventList.remove(e); } } //2. Add a method to Controller called shutdown, protected void shutdown(String message) { System.out.println("ShutDown from controller"); } }
public abstract class Event { private long eventTime; protected final long delayTime; public Event(long delayTime) { this.delayTime = delayTime; start(); } public void start() { // Allows restarting eventTime = System.currentTimeMillis() + delayTime; } public boolean ready() { return System.currentTimeMillis() >= eventTime; } public abstract void action() throws ControllerException; }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
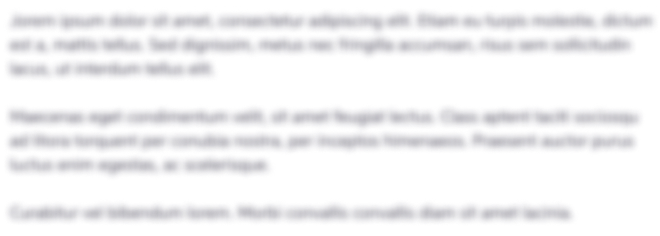
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started