Question
In Part B, you will add a structured record (struct) named CellId to identify a position on the game board by its row and column.
In Part B, you will add a structured record (struct) named CellId to identify a position on the game board by its row and column. You will also add and document some functions that work with CellIds. Put the record declaration and function prototypes in CellId.h and the function implementations in CellId.cpp.
Note: The CellId type is not encapsulated. Client code is expected to use the row and column fields directly.
By the end of Part B, you will have functions with the following prototypes:
CellId toCellId (int row1, int column1);
bool isOnBoard (const CellId& cell_id);
bool isOnBoard (const std::string& str);
CellId toCellId (const std::string& str);
void printCellId (const CellId& cell_id);
You will notice that in C++, two functions can have the same name as long as they have different types of parameters. Note: They are called overloaded funtions.
Perform the following steps:
- In CellId.h, define the CellId structured record to represent a cell position. It should have member fields named row and column, both of which should be ints.
- Copy in the function prototypes.
- In CellId.cpp, include the
, , and libraries as well as "BoardSize.h" and "CellId.h". Remember to put using namespace std;. The function prototypes use the string type, so you will need to include the library in CellId.h as well. - In CellId.cpp, add an implementation for the toCellId function that takes row and column parameters. First, it should create a local CellId variable. Then it should set the CellId's row and column. Use the name of the variable you just created and then the dot operator to choose the row component of the variable. Finally, it should return the CellId.
- Add an implementation for the isOnBoard function that takes a constant reference to a CellId as a parameter. It should return a value indicating whether or not that CellId is on the board. To be on the board, the cell row and column must both be greater than or equal to 0 and strictly less than BOARD_SIZE.
- Add an implementation for the printCellId function. It should print the cell name to standard output (cout).
- Reminder: The cell name is always an uppercase letter, representing a row, followed by a digit, representing a column.
- Hint: You have functions that give the name for a row and the name for a column.
- Add an implementation for the isOnBoard function that takes a constant reference to a string (const string&) as a parameter. It should return a value indicating whether or not that string names a cell on the board. To name a cell, the string must be composed of exactly 2 characters. If the length of the string is not 2, you should immediately return false. A cell name must consist of: an uppercase letter representing a row, followed by a digit representing a column. If the string names a cell and that cell is on the board, the function should return true. Otherwise, it should return false.
- Hint: If a cell is on the board, the row name will be at least 'A' and strictly less than 'A' + BOARD_SIZE. Similarly, the column name will be at least '0' and strictly less than '0' + BOARD_SIZE.
- Hint: To get the letter specifying the row, you will have to access position 0 of your string parameter and to get the digit specifying the column, you will have to access position 1.
- Add an implementation for the toCellId function that takes a constant reference to a string as a parameter. It should create, initialize, and return a CellId corresponding to the cell named in the parameter string.
- Hint: To create the CellId, you need to declare a variable of that type.
- Hint: To initialize the CellId, you need to use the dot operator on the variable you just declared. You need to assign a value to each field of the variable using =. From step B1, you should have fields for the row and column.
- Hint: To determine the column number, you will need to extract one character from position 1 of the string. When you extract one character, you will have a character value such as '5', which is not equal to the integer 5. Suppose you store this character in a char variable named ch. For a character stored in char variable ch, you need to compute ch '0' to get the corresponding integer, which you will use as the actual row number.
- Hint: To determine the row number, proceed similarly. Extract one character from position 0 and subtract 'A'.
- Add a precondition to the toCellId (const std::string& str) function that requires isOnBoard to return true when applied to the string parameter. Enforce the precondition using an assert.
- Test your CellId module using the TestCellId2.cpp program provided. You will need TestHelper.h and TestHelper.cpp. To compile, you should compile BoardSize.cpp, CellId.cpp, TestCellId2.cpp, and TestHelper.cpp together.
Note: Whenever you use #include to include a .h file in your folder, always compile the corresponding .cpp file when creating your executable file.
- Write interface specifications (comments in a certain format) for the functions in CellId.h in the format described in class and in Section 4a of the online notes. For example, the comments in Player.h give interface specifications for the functions there.
- Hint: When documenting a precondition, the easiest and most helpful way is just to put the code from inside the assert. For example: // Pecondition(s): // <1> n > 0
- Note: Interface specifications are written for other programmers (or you in the future) who want to use your module and need to know how to do so. Assume that the client who wants to use your module can (and will) look at your interface (.h) file but cannot look at your implementation (.cpp) file.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
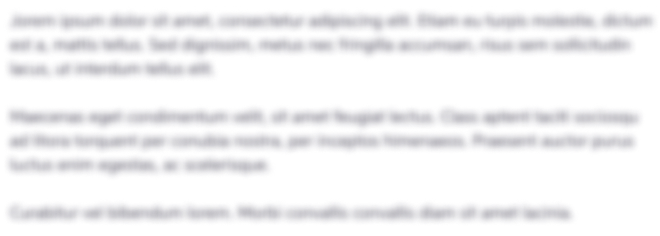
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started