Question
IN PYTHON: I need to call a new file that will import format changes to apply to another file's output. Before formatting the output, you
IN PYTHON:
I need to call a new file that will import format changes to apply to another file's output. Before formatting the output, you will create a Python module file containing functions to assist with producing formatted output. You will use the import statement to include code from your Python module.
- Create cis122_assign06_shared.py file using IDLE Editor
- Note: An underscore is required rather than a dash in a module filename.
- Create the following three functions:
- pad_string(): Parameters include data to be formatted, the size of the format space, a direction (e.g. left padded or right padded), and the padding character; direction must default to left padded, and the padding character must default to a single space; if the the length of the data to be formatted is greater than the size of the format space, simply return the original data to be formatted
- pad_left(): Parameters include data to be formatted, the size of the format space, and the padding character; call pad_string(), passing the data, size and formatting character, and explicitly set the padding direction to left padded
- pad_right(): Parameters include data to be formatted, the size of the format space, and the padding character; call pad_string(), passing the data, size and formatting character, and explicitly set the padding direction to right padded
You should first create and test pad_string(), then add and test pad_left() and pad_right().
To test your code module, you could add test code to your Python code, but you can also simply run the module. No output will appear in the IDLE Shell as the file only contains function definitions; however, once executed, you can use the functions directly in the IDLE Shell.
Part V: Format Printed Output
Revisit your code that prints the results and apply both pad_left() and pad_right() functions to format your output to match the output below (your total and average values will be different).
- Use the import function to include the module. Only import the pad_left() and pad_right() functions.
from cis122_assign06_shared import pad_left, pad_right
- Use the functions as expressions to format the printed output
- Use 10 as the padding space for both pad_left() and pad_right(), but rather than code 10 directly into each function call, create two variables, label_spacing and num_spacing,that may be easily changed to adjust the formatting.
Part VI: Input Filename
Add a while loop to prompt for the integers filename. You will use guardian code to test if no filename was entered and exit the program. You will also add code to close the file.
- Add a while loop that prompts for the integers filename using the prompt below:
- Use the strip() method to remove leading and trailing white space
- Use break to exit the program if no filename is entered
- Use the close() method to close the file object once you've completed reading the file
- Once the file has been read, closed, and results printed, re-prompt for the filename.
Part VII: Test File Existence
Even though you will only read a single file, you should check to see if the file exists before attempting to open the file. We will use the os Python library to test if the file exists, specifically using os.path.exists() method. Note that the path object is an object found in the os library.
- Add an import statement for the os library to your Python file
import os
- Add a conditional statement that tests for the existence of the entered file. You may want to consider an elif and the not operator. Use the following expression to test for existence of a file where the name of the file is stored in a variable filename.
os.path.exists(filename)
- Output the following error message with the name of the invalid file if the file does not exist, and re-prompt for the input file.
Here is my code so far:;
# to count number counter = 0 # sum of numbers total_sum = 0
r = "random_numbers.txt"
# Counting comments def count_comments(file_name): fin = open(file_name) comments = 0 for line in fin: if line.startswith('#'): comments += 1 fin.close() return comments
# Reading the file till end def count_numbers(file_name): fin = open(file_name) numbers = [] for line in fin: line = line.strip() if not line.startswith('#'): numbers.append(int(line)) fin.close() return numbers
numbers = []
numbers= count_numbers(r)
total_sum = 0 # For total numbers for i in numbers: total_sum = total_sum + int(i) counter = counter + 1
# Printing total print("Count: ", len(numbers)) print("Comments: ", count_comments(r)) print("Total: ", total_sum) print("Average: ", (total_sum/len(numbers)))
>>> pad_left("abc", 10) abc' >>> pad_right ("abc", 10) abc 100 Count: Comments: Total: Average : 4796 47.96 Enter filename (blank to exit): Enter filename (blank to exit): random_numbers.txt Count: 100 Comments: Total: 4796 Average: 47.96 Enter filename (blank to exit): Enter filename (blank to exit): somefile.txt Invalid filename: somefile.txt Enter filename (blank to exit): (bilo potreba somety >>> pad_left("abc", 10) abc' >>> pad_right ("abc", 10) abc 100 Count: Comments: Total: Average : 4796 47.96 Enter filename (blank to exit): Enter filename (blank to exit): random_numbers.txt Count: 100 Comments: Total: 4796 Average: 47.96 Enter filename (blank to exit): Enter filename (blank to exit): somefile.txt Invalid filename: somefile.txt Enter filename (blank to exit): (bilo potreba somety
Step by Step Solution
There are 3 Steps involved in it
Step: 1
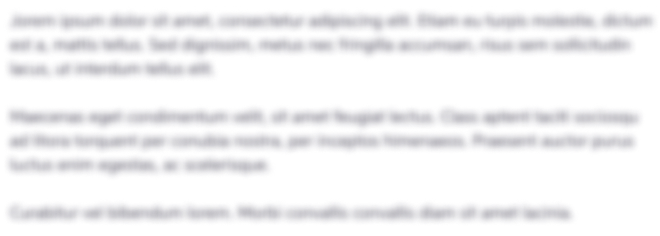
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started