Question
In Python: Modify the supermarket checkout simulator so that it simulates astore with many checkout lines that have their own cashierscontaining the customers processed per
In Python:
Modify the supermarket checkout simulator so that it simulates astore with many checkout lines that have their own cashierscontaining the customers processed per cashier, average wait timein each checkout line and the amount of customers left in eachcheckout line. Add the number of cashiers as a new user input.
In the cashier.py file, complete the following:
Modify the __init__ method:
Maintains a cashier's number with the number as an instance of__init_.
Modify the __str__ method:
Handles totalCustomerWaitTime as a float type
Checks if there is check out lines where no customers have beenserved
If no customers were served set aveWaitTime to 0.00
Only store the results of the simulation and don't print them. Theresults should only be printed from the model.
In the marketmodel.py file, complete the following:
At instantiation, the model should create a list of thesecashiers.
Modify the runSimulation method:
When a customer is generated, it should be sent to a cashierrandomly chosen from the list of cashiers.
On each tick for the length of the simulation, each cashier shouldbe told to serve its next customer.
Modify the __str__ method:
At the end of the simulation, the results for each cashier shouldbe displayed.
To test your program run the main method in the marketapp.pyfile.
Your program's output should look like the following:
Enter the total running time: 30
Enter the average processing time per customer: 3
Enter the probability of a new arrival: 1
Enter the number of cashiers: 6
----------------------------------------
CASHIER CUSTOMERS AVERAGE LEFT IN
PROCESSED WAIT TIME LINE
1 4 0.25 0
2 5 0.00 0
3 7 0.86 0
4 6 1.50 0
5 4 0.25 0
6 4 0.50 0
Output will vary because a cashier is randomly chosen.
-------------------------------------------------------------------------------------------------------------------------
"""
File: cashier.py
Project 8.4
Models multiple cashiers.
"""
from linkedqueue import LinkedQueue
class Cashier(object):
"""Represents a cashier."""
def __init__(self):
"""Maintains a cashier number, a queue of customers,
number of customers served, total customer wait time,
and a current customer being processed."""
# Write your code here
self.totalCustomerWaitTime = 0
self.customersServed = 0
self.currentCustomer = None
self.queue = LinkedQueue()
def addCustomer(self, c):
"""Adds an arriving customer to my line."""
self.queue.add(c)
def serveCustomers(self, currentTime):
"""Serves my cuatomers during a given unit of time."""
if self.currentCustomer is None:
# No customers yet
if self.queue.isEmpty():
return
else:
# Pop first waiting customer and tally results
self.currentCustomer = self.queue.pop()
self.totalCustomerWaitTime +=
currentTime -
self.currentCustomer.getArrivalTime()
self.customersServed += 1
# Give a unit of service
self.currentCustomer.serve()
# If current customer is finished, send it away
if self.currentCustomer.getAmountOfServiceNeeded() == 0:
self.currentCustomer = None
def __str__(self):
"""Returns my results: my total customers served,
my average wait time per customer, and customers left on my queue."""
result = "TOTALS FOR THE CASHIER" +
"Number of customers served: " +
str(self.customersServed) + ""
if self.customersServed != 0:
aveWaitTime = self.totalCustomerWaitTime /
self.customersServed
result += "Number of customers left in queue: " +
str(len(self.queue)) + "" +
"Average time customers spend" +
"waiting to be served: " +
"%5.2f" % aveWaitTime
return result
-------------------------------------------------------------------------------------------------------------------------
"""
File: customer.py
Project 8.4
Customer's processing time varies around the average,
so give it a random time between 1 and average time * 2 + 1.
"""
import random
class Customer(object):
"""Represents a customer."""
@classmethod
def generateCustomer(cls, probabilityOfNewArrival,
arrivalTime,
averageTimePerCustomer):
"""Returns a Customer object if the probability
of arrival is greater than or equal to a random number.
Otherwise, returns None, indicating no new customer.
"""
if random.random() <= probabilityOfNewArrival:
return Customer(arrivalTime, averageTimePerCustomer)
else:
return None
def __init__(self, arrivalTime, serviceNeeded):
"""Maintains the arrival time and amount of service needed."""
self.arrivalTime = arrivalTime
self.amountOfServiceNeeded = serviceNeeded
def getArrivalTime(self):
"""Returns the arrival time."""
return self.arrivalTime
def getAmountOfServiceNeeded(self):
"""Returns the amount of service needed."""
return self.amountOfServiceNeeded
def serve(self):
"""Accepts a unit of service from the cashier."""
self.amountOfServiceNeeded -= 1
-------------------------------------------------------------------------------------------------------------------------
Step by Step Solution
3.42 Rating (152 Votes )
There are 3 Steps involved in it
Step: 1
Cashierpy from linkedqueue import LinkedQueue class Cashierobject Represents a cashier def initself ...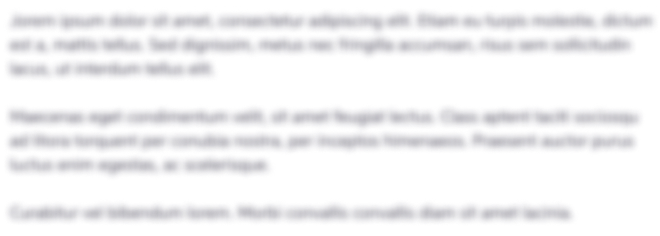
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started