Answered step by step
Verified Expert Solution
Question
1 Approved Answer
IN python please Question 1 90 points 85 mins This question is about OBJECT-ORIENTED PROGRAMMING. Write a Python class named Histogram that keeps track of
IN python please
Question 1 90 points 85 mins This question is about OBJECT-ORIENTED PROGRAMMING. Write a Python class named Histogram that keeps track of how many times each unique element or category in a given sequence occurs. You must implement this class from scratch. That is, your implementation cannot use the dict, collections.defaultdict, or collections. Counter data types anywhere for any purpose whatsoever! If you do, unfortunately, you will automatically receive a zero for this exam! . In addition, your implementation cannot also use any import statements anywhere as well! If you do, unfortunately, you will automatically receive a zero for this exam! WARNING! If your code violates any of these two restrictions, your grade for this entire exam will automatically be zero! The Histogram class will have the following public interface: Histogram( sequence will initialize a histogram of all individual elements or categories occurring in the provided sequence. get( category, default=None ) will return the frequency, that is, how many times a given element or category occurs in the given histogram. For example, >>> h = Histogram( 'aaabbbbc' ) >>> h.get( 'b') 4 If the key or category name provided to get() does not occur in the histogram, then get() should return the value provided as default in the default argument. If no default argument is provided, get() should return None for a non-occurring key. For example, >>> h.get( 's', 5) 5 since character s does not occur in the input string. However, >>> h.get('s') None most_freq( n=5 ) should return the most frequently occurring elements in the given sequence. If n=1 , then the most frequent category value should be returned in a 2-tuple along with its frequency value. For example, >>> h.most_freq(n=1) ('b', 4) If n is larger than then the function should return an ordered list of (key, value) tuples of the top n most frequent values, starting with the highest occurring category entry. For example, >>> h.most_freq(n=2) [('b', 4), ('a', 3)] If n is larger than the number of elements, then this method should return all elements and their corresponding values. For example, >>> h.most_frequent( 100 ) [('b', 4), ('a', 3), ('c', 1)] Note that the default value of n is 5. immaterial. However, the frequencies() should return the frequency values in the histogram in a list. The order of the returned values order of the frequenc values should match that returned from categories(). See below. categories() should return a list of categories in the histogram, akin to the keys in a dictionary. data() should return a list of all the data in the histogram as (category, frequency) two-tuples, in which the first item is the category and second item is the frequency of that category. Note that the order of the returned (category, frequency) pairs is not important. update sequence ) should update the existing histogram data with the category data in the provided sequence. This method does not have an explicit return value. For example, >>> h = Histogram( "aaabbc" ) >>> h.data() [('a': 3), ('b', 2), ('c': 1)] >>> h. update( "fcfcfcfdfeefeee" ) >>> h.data() [('a', 3), ('b', 2), ('c', 4), ('f', 6), ('d', 1), ('e', 5)] >>> h.most_freq() [('f', 6), ('e', 5), ('c', 4), ('a', 3), ('b', 2)] deletel category ) should remove the indicated category from the histogram and return the frequency value for that category. If the specified category is not present in the histogram, the delete() method should raise a KeyError . See the test cases below. For example, >>> h = Histogram( "aaabbc" ) >>> h.delete( 'b') >>> h.data() [('a', 3), ('c',1)] >>> h.delete 'k') KeyError: "No such category: 'k'" VERY IMPORTANT You must solve this question without using any external modules, that is, without any import statements! If you do, you will automatically get a zero for this question. Make sure you do not remove the file magic command, file histogram.py, at the top of the code cell below. If you remove or modify this command, the tests below will fail, and your grade will be affected. Make sure that running the code cell below creates a file called histogram.py in the same directory as this notebook. The rest will be handled by the testing code. PROVIDE YOUR ANSWER IN THE FOLLOWING CODE CELL In []: file histogram.py # YOUR CODE HERE pass class Histogram: SEE ABOVE SPECIFICATIONS # YOUR CODE HERE pass TEST CASES: In [ ]: # TEST #1 # # from histogram import Histogram h = Histogram( "aababbacbcadacedef" ) student answer = sorted( h.data() ) correct answer = sorted ( [('a', 6), ('b', 4), ('c', 3), ('d', 2), ('e', 2), ('f', 1)] ) assert student_answer == correct_answer In [ ]: # # TEST #2 # # from histogram import Histogram h = Histogram( "aababbacbcadacedef" ) student_answer = h.get( 'a') correct answer = 6 assert student_answer == correct_answer In (): # # TEST #3 # # from histogram import Histogram h = Histogram( "aababbacbcadacedef" ) student_answer = h.get( 'X', 5) correct answer = 5 assert student_answer == correct answer In [ ]: # # TEST #4 # # from histogram import Histogram h = Histogram( "aababbacbcadacedef" ) student_answer = h. most_freq( 3 ) correct answer = [('a', 6), ('b', 4), ('c', 3)] assert student_answer == correct_answer In (): # # TEST #5 # # from histogram import Histogram h = Histogram( "aababbacbcadacedef" ) student_answer = h. most_freq( 1 ) correct answer = ('a', 6) assert student_answer == correct_answer In [ ]: # # TEST #6 # # from histogram import Histogram h = Histogram( "aababbacbcadacedef" ) student answer = h. most_freg() correct answer = [('a', 6), ('b', 4), ('c', 3), ('d', 2), ('e', 2)] assert student_answer == correct_answer In [ ]: # TEST #7 # # from histogram import Histogram h = Histogram( "aababbacbcadacedef" ) student_answer = sorted( h.categories()) correct answer = sorted ( ['a', 'b', 'c','d', 'e': 'f']) assert student_answer == correct answer In [ ]: # # TEST #8 # # from histogram import Histogram h = Histogram( "aababbacbcadacedef" ) student_answer = sorted( h. frequencies()). correct answer = sorted [6, 4, 3, 2, 2, 1] ) assert student_answer == correct_answer In [ ]: # # TEST #9 # # from histogram import Histogram h = Histogram( "aababbacbcadacedef" ) h.deletel 'a') student_answer = sorted( h.data() ) correct answer = sorted ( [('b', 4), ('c', 3), ('d', 2), ('e', 2), ('f', 1)) ) assert student_answer == correct answer In [ ]: # # TEST #10 # # from histogram import Histogram h = Histogram( "aababbacbcadacedef" ) try: h.delete( 'X' ) except KeyError as ke: #print( ke ) pass else: print("*** FIX YOUR IMPLEMENTATION! ***" ) assert False
Step by Step Solution
There are 3 Steps involved in it
Step: 1
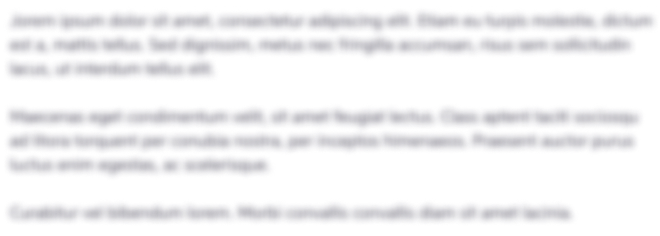
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started