Question
//in scala //the problem statement Write a function reduce that takes in two parameters: a function f that takes in two Ints and returns an
//in scala
//the problem statement
Write a function reduce that takes in two parameters: a function f that takes in two Ints and returns an Int, and a list xs of Ints. You should use the function f to combine all of the elements of the list. For instance, if I have a list and a function as follows:
def ls = 7::2::5::1::Nil
def add(x:Int, y:Int) = x+y
then reduce(add, ls) would return 7+2+5+1= 15. Ensure that your function would also do the right thing if called with a function that multiplied two inputs.
//this is the code I have so far but it's not working, please fix it
object MyClass { //function reduce that takes in two parameters: a function f that takes in two Ints and returns an Int, and a list xs of Ints. def reduce(f: (Int, Int) => Int, xs: List[Int]): Int = { if (x == 0) f(0) else if (x == 1) f(1) else f(x, reduce(f, reduce)) } def add(x: Int, y: Int): Int = x + y def multiply(x: Int, y: Int): Int = x * y
def main(args: Array[String]) { val xs = List(7, 2, 5, 1) val sum = reduce(add, xs) // sum = 15 val product = reduce(multiply, xs) // product = 70 println(reduce(add, ls)) println(reduce(multiply,ls)) } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
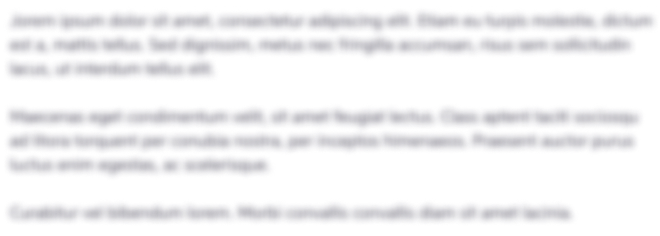
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started