Question
In Section 5.7.1, we presented a semaphore-based solution to the producerconsumer problem using a bounded buffer. In this project, you will design a programmingsolution tothe
In Section 5.7.1, we presented a semaphore-based solution to the producerconsumer problem using a bounded buffer. In this project, you will design a programmingsolution tothe bounded-buffer problemusing the producer and consumer processes shown in Figures 5.9 and 5.10. The solution presented in Section 5.7.1 uses three semaphores: empty and full, which count the number of empty and full slots in the buffer, and mutex, which is a binary (or mutualexclusion) semaphore that protects the actual insertion or removal of items in the buffer. For this project, you will use standard counting semaphores for emptyandfullandamutexlock,ratherthanabinarysemaphore,torepresent mutex.Theproducerandconsumerrunningasseparatethreadswillmove itemstoandfromabufferthatissynchronizedwiththeempty,full,andmutex structures. You can solve this problem using either Pthreads or the Windows API. #include "buffer.h" /* the buffer */ buffer item buffer[BUFFER int insert item(buffer SIZE]; item item) { /* insert item into buffer return 0 if successful, otherwise return -1 indicating an error condition */ } int remove item(buffer item *item) { /* remove an object from buffer placing it in item return 0 if successful, otherwise return -1 indicating an error condition */ } The Buffer Figure 5.24 Outline of buffer operations. Internally, the buffer will consist of a fixed-size array of type buffer (which will be defined using a typedef). The array of buffer item objects will be manipulated as a circular queue. The definition of buffer item,along with the size of the buffer, can be stored in a header file such as the following: /* buffer.h */ typedef int buffer #define BUFFER item; SIZE 5 The buffer will be manipulated with two functions, insert remove item item() and item(), which are called by the producer and consumer threads, respectively. A skeleton outlining these functions appears in Figure 5.24. The insert item() and remove item() functions will synchronize the producer and consumer using the algorithms outlined in Figures 5.9 and 5.10. The buffer will also require an initialization function that initializes the mutual-exclusion object mutex along with the empty and full semaphores. The main() function will initialize the buffer and create the separate producer and consumer threads. Once it has created the producer and consumer threads, the main() function will sleep for a period of time and, upon awakening, will terminate the application. The main() function will be passed three parameters on the command line: 1. How long to sleep before terminating 2. The number of producer threads 3. The number of consumer threads #include "buffer.h" Programming Projects 255 int main(int argc, char *argv[]) { /* 1. Get command line arguments argv[1],argv[2],argv[3] */ /* 2. Initialize buffer */ /* 3. Create producer thread(s) */ /* 4. Create consumer thread(s) */ /* 5. Sleep */ /* 6. Exit */ } Figure 5.25 Outline of skeleton program. Askeleton for this function appears in Figure 5.25. The Producer and Consumer Threads The producer thread will alternate between sleeping for a random period of time and inserting a random integer into the buffer. Random numbers will be produced using the rand() function, which produces random integers between 0 and RAND MAX. The consumer will also sleep for a random period of time and, upon awakening, will attempt to remove an item from the buffer. Anoutline of the producer and consumer threads appears in Figure 5.26. As noted earlier, you can solve this problem using either Pthreads or the Windows API. In the following sections, we supply more information on each of these choices. Pthreads Thread Creation and Synchronization Creating threads using the Pthreads API is discussed in Section 4.4.1. Coverage of mutex locks and semaphores using Pthreads is provided in Section 5.9.4. Refer to those sections for specific instructions on Pthreads thread creation and synchronization. Windows Section 4.4.2 discusses thread creation using the Windows API. Refer to that section for specific instructions on creating threads. Windows MutexLocks Mutex locks are a type of dispatcher object, as described in Section 5.9.1. The following illustrates how to create a mutex lock using the CreateMutex() function: #include
Step by Step Solution
There are 3 Steps involved in it
Step: 1
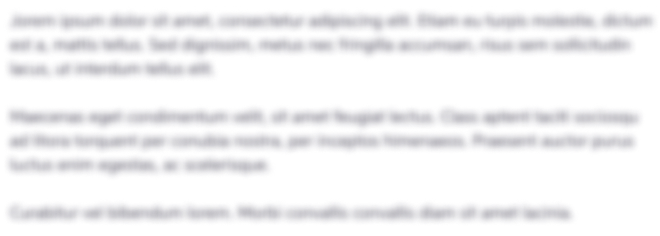
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started