Question
In the 1D Arrays Unit, i have created a command-line Contact Manager v1 which is given below. Now upgrade the software to include a graphic
In the 1D Arrays Unit, i have created a command-line Contact Manager v1 which is given below. Now upgrade the software to include a graphic user interface.
//written by - Adil Mahmood //Sunday April 10 2022 /** * The Contact Manager Assignment class implements an application that demonstrates * Contact Manager Assignment */ import java.util.Scanner;
class Contact { // fields private String name; private String number;
// constructor public Contact(String name, String number) { this.name = name; this.number = number; }
// getters and setters public String getName() { return this.name; }
public void setName(String name) { this.name = name; }
public String getNumber() { return this.number; }
public void setNumber(String number) { this.number = number; } } public class ContactManager { // fields private Contact[] contacts; private int numContacts;
// constructor public ContactManager() { this.contacts = new Contact[100]; this.numContacts = 0; }
// methods // view all contacts public void viewAll() { for (int i = 0; i < numContacts; i++) { System.out.println("Name: " + contacts[i].getName()); System.out.println("Phone number: " + contacts[i].getNumber()); } }
// add a contact public void add(String name, String number) { contacts[numContacts] = new Contact(name, number); numContacts++; }
// modify a contact public void modify(String name, String number) { for (int i = 0; i < numContacts; i++) { if (contacts[i].getName().equals(name)) { contacts[i].setNumber(number); } } }
// delete a contact public void delete(String name) { for (int i = 0; i < numContacts; i++) { if (contacts[i].getName().equals(name)) { for (int j = i; j < numContacts - 1; j++) { contacts[j] = contacts[j + 1]; } numContacts--; } } }
public void printMenu() { System.out.println("1. View all contacts"); System.out.println("2. Add a contact"); System.out.println("3. Modify a contact"); System.out.println("4. Delete a contact"); System.out.println("5. Save book"); System.out.println("6. Load book"); System.out.println("7. Quit"); }
public static void main(String[] args) { ContactManager manager = new ContactManager(); Scanner in = new Scanner(System.in); String input; int choice;
do { manager.printMenu(); input = in.nextLine(); choice = Integer.parseInt(input);
if (choice == 1) { manager.viewAll(); } else if (choice == 2) { System.out.println("Enter name:"); input = in.nextLine(); String name = input; System.out.println("Enter number:"); input = in.nextLine(); String number = input; manager.add(name, number); } else if (choice == 3) { System.out.println("Enter name:"); input = in.nextLine(); String name = input; System.out.println("Enter number:"); input = in.nextLine(); String number = input; manager.modify(name, number); } else if (choice == 4) { System.out.println("Enter name:"); input = in.nextLine(); String name = input; manager.delete(name); } else if (choice == 5) { // save } else if (choice == 6) { // load } else if (choice == 7) { System.out.println("Quitting..."); } } while (choice != 7); }
}
Your contact manager must:
- Have a menu bar that provides access to the following functionality (it is up to you to organize):
- Quitthe application
- Savethe Contacts
- Loadthe Contacts
- Adda Contact
- Deletea Contact
- Edita Contact
- Viewall entries in a table
- Sortthe Contacts by a selected field
- Store the contacts in a 1D Contact array with at least the following fields:
- First Name
- Last Name
- Address
- Phone Number
- Use methods to incorporate a modular design.
- Use proper internal documentation as per the Java Coding Conventions.
- Include the executable JAR of your program.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
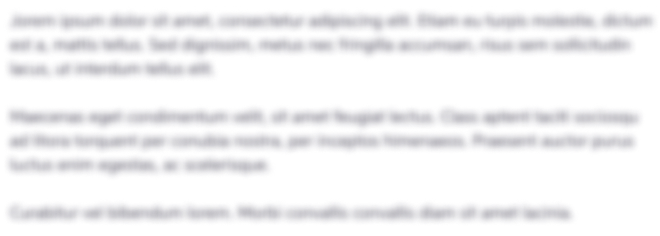
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started