Question
In the class Theater , implement the constructor and the following methods (headers are provided) cancelScreening closeTheater getPerformerMovieCount printMoviesICanWatch totalDurationOfAllMovies genreAfter directorAndPerformer Theatre class: public
In the class Theater, implement the constructor and the following methods (headers are provided)
- cancelScreening
- closeTheater
- getPerformerMovieCount
- printMoviesICanWatch
- totalDurationOfAllMovies
- genreAfter
- directorAndPerformer
Theatre class:
public class Theater { private ArrayList
/** * Constructor for objects of class Theater */ public Theater() { // initialise instance variables }
/** * Add the movies recorded in the given filename to the schedule (replacing * previous movies). * * @param filename A CSV file of Movie records. */ public void addMoviesFromFile(String filename) { MovieReader reader = new MovieReader(); screeningSchedule = new ArrayList<>(); screeningSchedule.addAll(reader.getMovies(filename)); }
public void printAllMovies() { // prints for all movies the corresponding info // movies are separated by an empty line screeningSchedule.stream().forEach(movie ->movie.printInfoSimple()); }
public void printMoviesBefore(String time) { // prints all movies which play before the given time // one movie per line String[] timeHoursMinute = time.split(":"); int hours = Integer.parseInt(timeHoursMinute[0]); int minutes = Integer.parseInt(timeHoursMinute[1]); int screenTimeInMinutes = (hours * 60) + minutes; screeningSchedule.stream().filter(movies -> movies.getScreeningTimeInMinutes()< screenTimeInMinutes).forEach(movies -> movies.printInfoSimple()); }
public void printMoviesInBefore(int theater, String time) { // prints all movies playing before the given time and in the given theater // one movie per line String[] timeHoursMinute = time.split(":"); int hours = Integer.parseInt(timeHoursMinute[0]); int minutes = Integer.parseInt(timeHoursMinute[1]); int screenTimeInMinutes = (hours * 60) + minutes; screeningSchedule.stream().filter(movies -> movies.getScreeningTimeInMinutes()< screenTimeInMinutes) .filter(movies -> (movies.getTheaterNumber() == theater)).forEach(movies -> movies.printInfoSimple()); }
public void moviesInTheater(int theater) { // prints all movies that play in the given theater // one movie per line screeningSchedule.stream().filter(movies -> (movies.getTheaterNumber() == theater)).forEach(movies -> movies.printInfoSimple()); }
public int numberOfMoviesInTimePeriod(String start, String end) { // returns number of movies that start screening between start and end time String[] startTimeeHoursMinute = start.split(":"); int hours = Integer.parseInt(startTimeeHoursMinute[0]); int minutes = Integer.parseInt(startTimeeHoursMinute[1]); int startTimeInMin = (hours * 60) + minutes;
String[] endTimeHoursMinute = end.split(":"); hours = Integer.parseInt(endTimeHoursMinute[0]); minutes = Integer.parseInt(endTimeHoursMinute[1]); int endTimeInMin = (hours * 60) + minutes; return this.screeningSchedule.stream() .filter(movie -> (movie.getScreeningTimeInMinutes() > startTimeInMin && movie.getScreeningTimeInMinutes() < endTimeInMin)) .map(movie -> 1).reduce(0, (total, count) -> total + count); }
public void cancelScreening(String movie, int theater) { // removes a given movie in a given theater from the screening schedule this.screeningSchedule.removeIf(mov -> (mov.getTheaterNumber() == theater && mov.getMovieTitle().equals(movie))); }
public void closeTheater(int theater) { // removes all movies in a given theater from the the screening schedule }
public int getPerformerMovieCount(String performer) { // calculates the number of screened movies in which a given performer plays // (according to the screening schedule) return 0; }
public void printMoviesICanWatch(String start, String end) { // prints all movies that screen after the start time and finish screening // before the end time }
public int totalDurationOfAllMovies() { // returns the combined duration of all movies played in all theaters return 0; }
public String genreAfter(String time) { // returns a string which includes all genres playing after given time return ""; }
public String directorAndPerformer(String director, String performer) { // returns a string which includes the titles of movies directed by director and // in which performer is acting return ""; }
}
Movie class:
public class Movie { private String movieTitle; private String screeningTime; private int durationInMinutes; private int theaterNumber; private String genre; private String directorName; private String leadPerformer;
final String[] VALID_GENRES = { "drama", "comedy", "thriller", "horror", "family", "action", "adventure" };
/** * Constructor for objects of class Movie */ public Movie(String title, String time, int duration, int theater, String genre, String director, String performer) { /*if (!this.checkDuration(duration)) { throw new IllegalArgumentException("Duration value is not valid."); } else if (!this.checkGenre(genre)) { throw new IllegalArgumentException("Genre is not valid."); } else if (this.checkTheaterNumber(theater)) { throw new IllegalArgumentException("Theater value is not valid."); } else if (this.checkScreeningTime(time)) { throw new IllegalArgumentException("Screen time is not valid."); } */ this.movieTitle = title; this.screeningTime = time; this.durationInMinutes = duration; this.theaterNumber = theater; this.genre = genre; this.directorName = director; this.leadPerformer = performer; } public String getMovieTitle() { return this.movieTitle; } public int getScreeningTimeInMinutes() { String[] timeHoursMinute = this.screeningTime.split(":"); int hours = Integer.parseInt(timeHoursMinute[0]); int minutes = Integer.parseInt(timeHoursMinute[1]); int screenTimeInMinutes = (hours * 60) + minutes;
return screenTimeInMinutes; } private boolean checkGenre(String genre) { boolean contains = Arrays.stream(VALID_GENRES).anyMatch(genre::equals); return contains; }
private boolean checkTheaterNumber(int theater) { return theater <= 16 && theater >= 1; } public int getTheaterNumber() { return this.theaterNumber; } private boolean checkScreeningTime(String time) { // converting time into units. IE: 23:45 = [(23 * 60) + 45] int screenTimeUpperBound = 1425; // 23:45 converted into time minutes int screenTimeLowerBound = 600; // 10:00 converted into time minutes
// Convert 24hr time format to minutes String[] timeHoursMinute = time.split(":"); int hours = Integer.parseInt(timeHoursMinute[0]); int minutes = Integer.parseInt(timeHoursMinute[1]); int screenTimeInMinutes = (hours * 60) + minutes;
// Checks if screen Time is with bounds. return screenTimeInMinutes >= screenTimeLowerBound && screenTimeInMinutes <= screenTimeUpperBound; }
private boolean checkDuration(int duration) { return duration >= 45 && duration <= 210; } public void printInfo() { StringBuilder movieInfoStringBuilder = new StringBuilder(); movieInfoStringBuilder.append("Title: " + this.movieTitle);
movieInfoStringBuilder.append("Title: " + this.movieTitle); movieInfoStringBuilder .append("\t ScreenTime: " + this.screeningTime + "(" + this.durationInMinutes + "in minutes)"); movieInfoStringBuilder.append("\tYou can locate it in Theater #" + this.theaterNumber); movieInfoStringBuilder.append("\t Genre: " + this.genre); movieInfoStringBuilder.append("Director: " + this.directorName); movieInfoStringBuilder.append("\t Movie Lead: " + this.leadPerformer);
System.out.println(movieInfoStringBuilder.toString()); } public void printInfoSimple() { StringBuilder movieInfoStringBuilder = new StringBuilder(); movieInfoStringBuilder.append(this.movieTitle); movieInfoStringBuilder.append("-" + this.screeningTime + "(" + this.durationInMinutes + "in minutes)"); movieInfoStringBuilder.append("-" + this.theaterNumber); movieInfoStringBuilder.append("-" + this.genre); movieInfoStringBuilder.append("-" + this.directorName); movieInfoStringBuilder.append("-" + this.leadPerformer + " "); System.out.println(movieInfoStringBuilder.toString()); } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
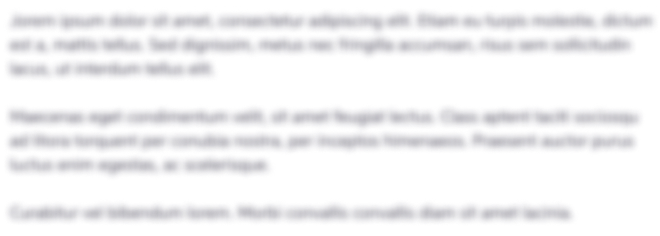
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started