Question
In the file strFuncs . cpp , write a function called isAnagram that takes two strings as arguments and returns a boolean true if the
In the file strFuncs.cpp, write a function called isAnagram that takes two strings as arguments and returns a boolean true if the two strings are anagrams, otherwise it returns false. The function should not be case sensitive and should disregard any punctuation or spaces. Two strings are anagrams if the letters can be rearranged to form each other. For example, Eleven plus two is an anagram of Twelve plus one. Each string contains one v, three es, two ls, etc. Similarly Rats and Mice and in cats dream are anagrams of each other. You may use any of the C string library or string class functions to complete this code. You may not use built-in C++ functions that we have NOT discussed in lecture. You must follow a TDD style of coding. Write your own test code in a separate file and write a Makefile to compile the code.
Code to follow and EDIT:
#include
#include
#include
#include
#include "strFuncs.h"
using namespace std;
/* Precondition: Two valid strings s1 and s2, each containing a mix of alphabet\
s, spaces and punctuations
* Post condition: Return true if one string is an anagram of the other string.\
White spaces, punctuations and
* the case for the letters (upper or lower) should not
* affect your result
*/
bool isAnagram(string s1, string s2){
return true;
}
/* Precondition: s1 is a valid string that may contain upper or lower case alph\
abets, no spaces or special characters
* Postcondition: Returns true if s1 is a palindrome, false otherwise
*You may provide a recursive OR non-recursive solution*/
bool isPalindrome(const string s1){
return true;
}
bool isPalindromeHelper(const char *s1, int len);
/* Precondition: s1 is a valid C-string that may contain upper or lower case al\
phabets, no spaces or special characters
* Postcondition: Returns true if s1 is a palindrome, false otherwise
*You MUST provide a recursive implementation and are recommended to write a he\
lper function where the recursion actually takes place*/
bool isPalindrome(const char *s1){
return true;
}
/* Precondition: s1 is a valid C-string that may contain upper or lower case al\
phabets, no spaces or special characters
* Postcondition: Returns true if s1 is a palindrome, false otherwise
*You MUST provide an iterative implementation */
bool isPalindromeIterative(const char *s1){
return true;
}
"strFuncs.h":
#ifndef STRFUNCS_H
#define STRFUNCS_H
using namespace std;
#include
bool isAnagram(string s1, string s2);
bool is Palindrome(const string s1);
bool isPalindrome (const char *s1);
bool isPalindromeIterative(const char *s1);
#endif //STRFUNCS_H
Step by Step Solution
There are 3 Steps involved in it
Step: 1
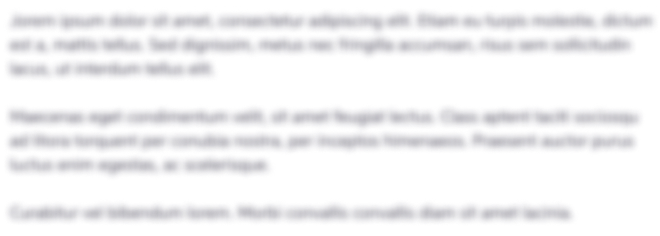
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started