Question
In the first three homeworks, you will develop a simulation for a building's elevator system. The major objects you will deal with in homeworks #1,
In the first three homeworks, you will develop a simulation for a building's elevator system. The major objects you will deal with in homeworks #1, #2 and #3 are:
- Building: Contains multiple Floors and one Elevator. (While multiple Elevators are possible, the homeworks will only deal with one.)
- Elevator: Carries Passengers between Floors. Has a limited capacity.
- Floor: Passengers spend some time on a Floor, and then use the Elevator to go to another Floor.
- Passenger: A person who enters and leaves the Building. While inside the Building, a Passenger is either on a Floor, or in the Elevator, moving between Floors.
In the first homework, you will only be concerned with the Elevator object. The other objects will be added in later homeworks. This initial version of the Elevator class will keep track of the number of passengers for each floor destined for each floor, and whether a stop on that floor is required. Any number of passengers may board the elevator. Elevator capacity will be limited in later homeworks.
The Elevator starts on the ground floor and goes up. When it reaches the top floor it starts going down. It keeps moving, stopping only at destination floors. A stop on a floor is necessary if there are passengers destined for that floor. In later homeworks, a passenger on a floor may summon the Elevator, requiring it to stop there, even if the Elevator is not carrying any passengers who want to get off there.
When the Elevator stops on a destination floor, it should discharge the passengers who wanted to go there, and note that the floor is no longer a destination.
Tasks
Elevator class
Write an Elevator class as follows:
- Create an Elevator class. The class should have a no-argument constructor that sets up the elevator's state.
- Define a constant, for the number of floors in the building, and set it to 7. Use it where appropriate.
- Define fields for tracking the Elevator's current floor, the direction of travel.
- Define an array-valued field for tracking, for each floor, the number of passengers destined for that floor.
- Define a move() method which, when called, modifies the Elevator's state, (i.e., updates the fields appropriately):
- Increments/decrements the current floor, i.e. the Elevator moves one floor at a time.
- Modifies the direction of travel, if the ground floor or top floor has been reached.
- Clears the array entry tracking the number of passengers destined for the floor that the elevator has just arrived at.
- Prints out the status of the Elevator [see toString() method below]
- Define a boardPassenger(int floor) method which adds to the Elevator a passenger destined for the indicated floor.
- Define a toString() method to aid in debugging and testing. The String returned by toString() should indicate the number of passengers on board, and the current floor.
This list defines requirements of the Elevator class. Your submission should therefore define all the fields and methods specified above, with appropriate access modes (private, public, etc.) You are free to provide additional fields and methods that you find to be useful.
In this homework, the only reason to stop at a floor is that a passenger wants to get off there. However, in future homeworks, passengers on a floor who want to leave the building (for example) will summon the Elevator, and then the Elevator must stop there, even if nobody already on the Elevator wants to stop there.
Testing
To demonstrate that your Elevator class is working properly, create an ElevatorTest.CPP file. The main() in this class should do the following:
- Create an Elevator object.
- Board two passengers for the 3nd floor, and one for the 5th floor.
- Move the Elevator from the ground floor to the top floor, and then back to the ground floor.
- Print the state of the elevator before the first move, and after each move. Your output should look like this:Floor 1: 3 passengersFloor 2: 3 passengersFloor 4: 1 passengerFloor 3: 1 passenger Floor 5: 0 passengersFloor 7: 0 passengersFloor 6: 0 passengers Floor 6: 0 passengers Floor 5: 0 passengersFloor 2: 0 passengersFloor 4: 0 passengers Floor 3: 0 passengersFloor 1: 0 passengersIn c++ pls
Step by Step Solution
There are 3 Steps involved in it
Step: 1
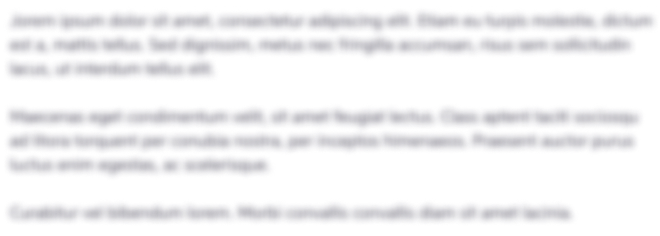
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started