Answered step by step
Verified Expert Solution
Question
1 Approved Answer
In the given skeleton code, implement the following: 1 . Implement insertion sort in the insertion sort function. This functions takes in an unsorted sequence
In the given skeleton code, implement the following:
Implement insertion sort in the insertion sort function. This functions takes in an unsorted sequence
given in first last and sorts them using insertion sort approach.
Implement the traditional merge operation in the merge function. This functions takes in two sorted
sequences first mid and mid last and merges them into a single sequence contained in first last
Implement the merge operation of nk subsequences in the merge k function. This functions takes
in nk sorted sequences defined in first last and merges them into a single sequence contained in
first last Here the input k is the size of each subsequence excluding the last which might be smaller
than k
Implement merge sort algorithm as stated above in the merge sort function.
Skeleton Code be#ifndef SORTH
#define SORTH
#include
#include
namespace myalgorithm
@brief Swap values of two elements
@tparam T Value type
@param a First value
@param b Second value
template
void swapT& a T& b
T tmp a;
a b;
b tmp;
@brief Sort the range first last into nondecreasing order
@tparam RandomAccessIterator Random Access Iterator
@tparam Compare Comparator function
@param first Initial position of sequence to be sorted
@param last Final position of sequence to be sorted
@param comp Binary function that accepts two elements in the range as
arguments and returns a value convertable to bool. The value
returned indicates whether the element passed as first argument
is considered to go before the second in the ordering it
defines.
Bubble Sort Example
template
void bubblesortRandomAccessIterator first, RandomAccessIterator last,
Compare comp
Traverse from left to right for each ith largest element positioning
forRandomAccessIterator i first; i last; i
Compare each element with its right neighbor to bubble up ith largest element
forRandomAccessIterator j first; j last; j
ifcompjj Compare j with its right neighbor
swapjj; Swap if neighbor is smaller
@brief Your implementation of insertion selection sort
@tparam RandomAccessIterator Random Access Iterator
@tparam Compare Comparator function
@param first Initial position of sequence to be sorted
@param last Final position of sequence to be sorted
@param comp Binary function that accepts two elements in the range as
arguments and returns a value convertable to bool. The value
returned indicates whether the element passed as first argument
is considered to go before the second in the ordering it
defines.
template
void insertionsortRandomAccessIterator first, RandomAccessIterator last, Comparator comp
@TODO
int i key, j;
for i ; i n; i
key RandomAccessIteratori;
j i;
while j && RandomAccessIteratorj key
RandomAccessIteratorj RandomAccessIterator j;
j j;
arrj key;
@brief Your implementation of merging two sorted sequences
@tparam RandomAccessIterator Random Access Iterator
@tparam Compare Comparator function
@param first Initial position of the first sequence to be merged
@param mid Initial position of the second sequence to be merged
@param last Final position of the second sequence to be merged
@param comp Binary function that accepts two elements in the range as
arguments and returns a value convertable to bool. The value
returned indicates whether the element passed as first argument
is considered to go before the second in the ordering it
defines.
template
void mergeRandomAccessIterator first, RandomAccessIterator mid, RandomAccessIterator last, Comparator comp
Hint: To allocate external memory to store merging of result use
std::vector
Remember to copy back the merged result
@TODO
@brief Your implementation of merging nk sorted sequences of size k
@tparam Randomlow:
Step by Step Solution
There are 3 Steps involved in it
Step: 1
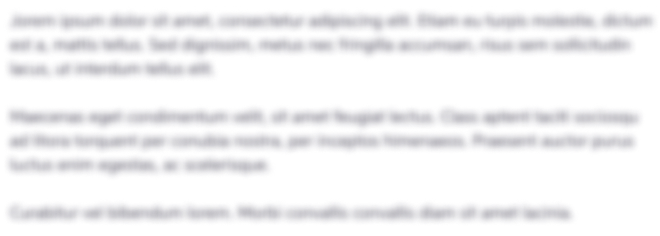
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started