Question
In the program below, you wrote a multithreaded program that estimated . However, there was a race condition on the shared variable that counted the
In the program below, you wrote a multithreaded program that estimated . However, there was a race condition on the shared variable that counted the number of random (x,y)(x,y) points that occurred within the circle. This assignment involves fixing this race condition using Pthreads mutex locks.
To-Do:
-
Make any necessary changes to the code below so that it correctly estimates using four threads.
-
Fix use of mutex lock to fix any race conditions
I would appreciate help to add these to-do items to this pre-existing code. Thank you and I look forward to your response. (:
/*
* This is a simple program that creates multiple threads
* to approximate Pi. It does so using Pthreads and by
* generating points randomly. It then checks if those points
* exist within the circle with a radius of 1.0.
*
* Points are generated and distributed among two threads.
* The threads create points and check and count the number.
* Lastly, pi is approximated by each thread. */
#include
#include
#include
#include
#include
#define POINTS 10 //points that will be distributed
#define THREADS 2 //create 2 threads
void *runner(void *param);
int circle_points = 0; //0 points in circle
double random_double() { //generate random double
return random() / ((double)RAND_MAX +1);
}
int main (int argc, const char * argv[]) {
int i;
double Pi;
int threadpoints = POINTS / THREADS; // points equally distributed between threads
pthread_t thread [THREADS]; //pthread_t to identify thread
srandom((unsigned)time(NULL)); //seed the random number generator
for (i = 0; i < THREADS; i++)
pthread_create(&thread[i], 0, runner, &threadpoints); //requires thread identifier
for (i = 0; i < THREADS; i++)
pthread_join(thread[i], NULL);
// calculate Pi
Pi = 4.0 * circle_points / POINTS;
;
//print results
printf(" # of Points: %d ", POINTS);
printf("Pi Approximation: %f ", Pi);
return 0;
}
void *runner(void *param) {
int POINTZ;
POINTZ = *((int *)param);
int i;
int count = 0;
double x,y;
for (int i = 0; i < POINTZ; i++) {
//generate random #'s between -1.0 and +1.0
//get random (x,y) point
x = random_double() * 2.0 - 1.0;
y = random_double() * 2.0 - 1.0;
//confirm (x,y) point is in the circle
if(sqrt(x*x + y*y) < 1.0)
++count;
}
circle_points += count;
pthread_exit(0);
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
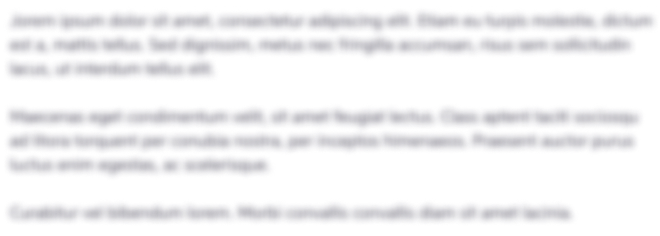
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started