Question
In the script element, add the JavaScript code for getting the users entry while the entry amount isnt 999. This should provide for multiple entries
In the script element, add the JavaScript code for getting the users entry while the entry amount isnt 999. This should provide for multiple entries and conversions.
Add the JavaScript code for deriving the letter grade from the table above and displaying it in an alert dialog box.
If you havent already done so, add data validation to make sure the entry is a valid number from 0 through 100. If the entry is invalid, the application should just display the starting prompt dialog box. It doesnt need to display a special error message.
To derive the letter grade, you should use this table:
A 88-100 B 80-87 C 68-79 D 60-67 F < 60
This what I have so far and I'm stuck on the if else part
var entry;
var letterGrade;
var total = 0;
var entryCount = 0;
var errorString = "Entry must by a valid number from 0 through 100 Or enter 999 to end entries";
do {
// get something from user
entry = prompt("Enter number grade from 0 through 100 Or enter 999 to end entries", 999);
// the user may also enter something that is not a number
if(isNaN(entry)){ // check if it is not a number
alert(errorString); //show error if not a number
}else{
// you now know you have a number so you can parse it
entry = parseInt(entry); // convert to number
// now check for a valid number
if (entry >= 0 && entry <= 100) {
// number is in valid range check each grade
if (entry < 60) {
letterGrade = "F";
}else
if (entry <= 67) {
letterGrade = "D";
}else
if (entry >= 68) {
letterGrade = "C";
}else
if (entry >= 80) {
letterGrade = "B";
}else
if (entry >= 88) {
letterGrade = "A";
}
total += entry; // add the total
entryCount += 1; // add ont to count
// display entry and grade
alert("Number grade = " + entry + " Letter grade = " + letterGrade);
}else // if not in valid range check if its 999
if (entry != 999) {
alert(errorString); // no show error
}
}
}while (entry != 999); // do this till 999 is entered
This page is displayed after the JavaScript is executed
Step by Step Solution
There are 3 Steps involved in it
Step: 1
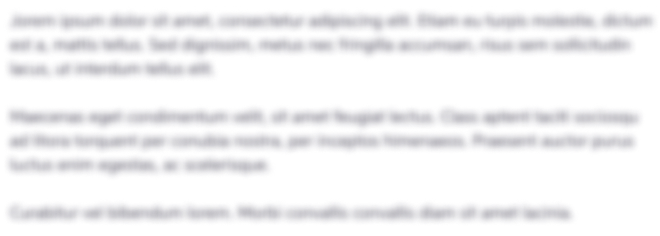
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started