Question
In this assignment we will explore the B-Tree data structure for use in data storage, specifically the use of its order property in a sorting
In this assignment we will explore the B-Tree data structure for use in data storage, specifically the use of its order property in a sorting application. As a use case, you will write a program to input a block of text and render it in both ascending and descending sort order.Similar to Assignment 1, text is read a line at a time using readLine (acm) or nextLine (scanner) until a blank line is encountered. The key difference is that each String object needs to be saved for later processing and output. A class such as ArrayList, which we will see later in Chapter 11, would normally be used for this purpose, but instead you will implement a bTree class to serve this purpose. Sorting becomes straightforward by performing an inorder traversal once the BTree is complete. Design Approach The program can be broken down into two classes, SortBuffer, which is responsible for all user interaction (Figure 1), and bTree, which implements a slightly more refined version of the bTree class shown in the notes. SortBuffer Has a structure much like Assignment 1, so you should be able to figure it out without any additional hints. You are not required to use the acm classes, but you can if you wish. bTree This class has the basic structure of the bTree class in the notes, but will require some modifications for this assignment. The most obvious is that the tree stores references to Sting objects, so a change to the bNode nested class will be required. bTree constructor The default constructor can be used as in the notes: bTree myTree = new bTree(); However, if you have done Java programming before, you could take an extra step and define a constructor for generic types by extending the Comparable class. Note that this is *optional*. The bTree class methods listed below are mandatory: public void addNode (String data) Adds a new String object to the bTree. public void displayInOrder() Performs an inorder traversal of the bTree, printing the string associated with each node. public void displayInReverseOrder() A slight modification of the displayInOrder method to traverse the bTree in reverse sort order. public void setDisplay(ConsoleProgram link) In order to use methods such as println() from the acm Program class, you will need to maintain an instance variable in bTree that provides a link to the ConsoleProgram instance, SortBuffer. If we assume that this variable is named link, then writing link.println() will enable println to be used within the bTree class. 3/4 Mandatory bTree methods continued: setDisplay is the setter called in the run() method once an instance of the bTree class has been created. Note: this is obviously not mandatory if not using the acm package.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
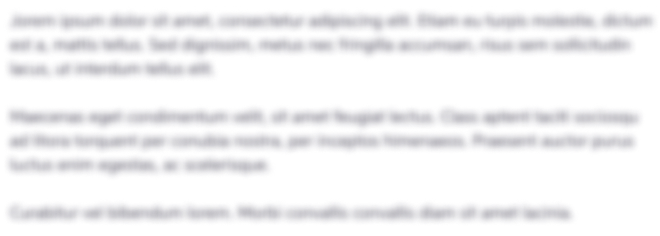
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started