Question
In this assignment, we will use Python to evaluate prefix expressions. Your algorithm will be recursive. Example Expressions: (+ (* 2 3) (* 4 5))
In this assignment, we will use Python to evaluate prefix expressions. Your algorithm will be recursive.
Example Expressions:
(+ (* 2 3) (* 4 5))
10
We must be able to recognize tokens in the expression. Notice that we can't simply split the expression on spaces since "(" and "+" are separate symbols, but are not separated by spaces.
Place all your code in p6.py
You must create the prefixReader function which does the following:
Reads each text line in the input file (which was passed as a command argument). For each text line:
It should print the prefix text string, preceded by "> "
It must tokenize the expression using Python's regex (use the re module). The Python Part 4 notes will be useful.
Reset the global current parsing pos to 0
It should invoke prefixEval passing the token array. If that function returned successfully (i.e., it didn't raise an exception), it should print the value returned from prefixEval.
It should provide a try except block which prints errors. After printing an error do not exit the program.
You must create the prefixEval function which is passed a token array and modifies the global current parsing pos:
Based on the token at the current position, if if is a:
( It is evaluating a function. prefixEval should treat the next token as a function name. It now needs to evaluate the arguments for that function:
-Assume the function has only two arguments. See extra credit for handling a variable number of arguments.
-It should invoke prefixEval to get the value of each argument (appropriately advancing the global current parsing position).
-It should advance the global current parsing position past its corresponding ")".
-It should invoke evalOperator passing the function and arguments and return that value as prefixEval's functional value.
number It should return that number's integer value as prefixEval's functional value.
prefixEval should use another function to actually apply the function to the operands. The following functions must be supported:
+ addition
minus - subtracts the second operand from the first
* multiplication
/ division - divides the second operand into the first, truncating the result
> greater than (numeric)
< less than (numeric)
and Boolean and returns True or False
or Boolean or returns True or False
Before returning, prefixEval advances the global current parsing position to the position immediately after its expression
Step by Step Solution
There are 3 Steps involved in it
Step: 1
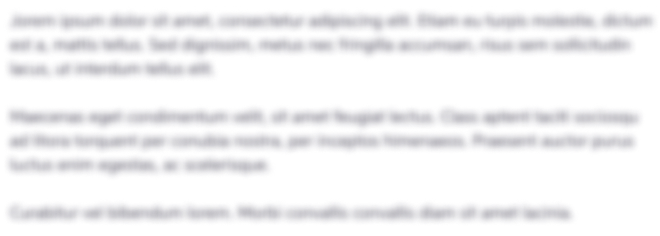
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started