Question
In this assignment, you are asked to write a program that stores and manipulates a list of (at-most 1000) polygons in C. There is a
In this assignment, you are asked to write a program that stores and manipulates a list of (at-most 1000) polygons in C. There is a starter code bellow. Each polygon must be stored in a structure like this:
typedef struct {
int numberOfVertices;
Direction shiftDirection;
Vertex* vertexList; } Polygon;
where Direction and Vertex are defined like this:
typedef enum {
NONE = 0,
UP = 1,
DOWN = 2,
LEFT = 4,
RIGHT = 8 }
Direction;
typedef struct {
int x, y; } Vertex;
Program Input Commands. There are five valid input commands:
add x1y1x2y2 . . . xnyn: adds an n-gon with vertices located at coordinates (x1, y1), (x2, y2), . . . , (xn, yn) to the list of all polygons. The newly added polygon must have its shiftDirection set to NONE.
summary: prints the programs list of polygons. For each polygon, you need to print the number of vertices and the x-y coordinates of the polygons centroid. To avoid the complications of calculating the centroid, you can assume that the x-coordinate (y-coordinate) of a polygons centroid is equal to the average of x-coordinates (ycoordinates) of the polgyons vertices 1.
turn k x: updates the shiftDirection of polygon stored at index k of the programs list of polygons. The shiftDirection has to be updated based on the value of x that can be equal to one of the following strings: left right up down right-up or up-right left-up or up-left right-down or down-right left-down or down-left
shift k s: updates the x-y coordinates of vertices of polygon stored at index k of the programs list of polygons. To update the vertices coordinates of a polygon, you need to check its shiftDirection. If the direction is NONE, then the shift command has no side effect. If the direction is RIGHT(LEFT), then s has to be added to(subtracted from) the x-coordinate of every vertex of the polygon. If the direction is UP(DOWN), then s has to be added to(subtracted from) the y-coordinate of every vertex of the polygon. If the direction is RIGHT and UP, then s has to be added to both the x-coordinate and y-coordinate of every vertex of the polygon, etc.
quit: ends the program.
_______________________________________________________________________________________________________________________________________________
#include#include #include #include "commands.h" typedef enum{ NONE =0, UP=1, DOWN=2, LEFT=4, RIGHT=8 }Direction; typedef struct{ int x,y; }Vertex; typedef struct{ int numberOfVertices; Direction shiftDirection; Vertex* vertexList; }Polygon; Polygon shift(int k,Direction s){ Polygon p; // write logic for shifting return p; } Polygon turn(int k,Direction x){ Polygon p; //Write logic for turning return p; } Polygon add(Vertex*vs){ Polygon p; p.shiftDirection = NONE; //Write logic for adding vertexes return p; } void commands(){ while(1){ char* input; scanf("%s",&input); if(strcmp(input,"quit") == 0) break; else if(strcmp(input,"summary") == 0) printf(""); else{ char* temp; for(int i=0;i<4;i++){ if(input[i]) *(temp+i) = *(input+i); else continue; } if(strcmp(temp, "turn")==0){ char *ktmp; int i=5; while(input[i]!= ' '){ *(ktmp+i) = *(input+i); } int k=0; sscanf(ktmp,"%d",&k); Direction x; i++; char*direction; while(input[i]){ *(direction+i) = *(input+i); } if(strcmp(direction,"RIGHT") == 0){ x = RIGHT; }else if(strcmp(direction,"LEFT") == 0){ x = LEFT; }else if(strcmp(direction,"UP") == 0){ x = UP; }else if(strcmp(direction,"DOWN") == 0){ x = DOWN; }else if(strcmp(direction,"up-right")){ x= UP+RIGHT; }else if(strcmp(direction,"up-left")){ x = UP+LEFT; }else if(strcmp(direction,"down-right")){ x = DOWN+RIGHT; }else if(strcmp(direction,"down-left")){ x = DOWN+LEFT; } else{ printf("INVALID INPUT"); } Polygon p = turn(k,x); printf(" "); } else if(strcmp(temp, "shift")==0){ char *ktmp; int i=5; while(input[i]!= ' '){ *(ktmp+i) = *(input+i); } int k=0; sscanf(ktmp,"%d",&k); char* direction; Direction s; i++; while(input[i]){ *(direction+i) = *(input+i); } if(strcmp(direction,"RIGHT") == 0){ s = RIGHT; }else if(strcmp(direction,"LEFT") == 0){ s = LEFT; }else if(strcmp(direction,"UP") == 0){ s = UP; }else if(strcmp(direction,"DOWN") == 0){ s = DOWN; }else{ printf("INVALID INPUT"); } Polygon p = shift(k,s); printf(" "); }else{ char*Tadd; for(int i=0;i<3;i++){ *(Tadd+i) = *(input+i); } Vertex* vs; int i=4; // the coordinates should be space separated while(input){ char*x,*y; for(int k=0;k<2;k++){ *(x+k) = *(input+i); *(y+k) = *(input+i+2); i++; } int a,b; sscanf(x,"%d",&a); sscanf(y,"%d",&b); vs->x = a; vs->y = b; } Polygon p = add(vs); printf(" "); } } } } int main(void) { printf(" "); commands(); return 0; }
I can't figure out the code for the commands. Please, help me with the add function and the turn, so I can continue with the shift. Thank you!!!
Step by Step Solution
There are 3 Steps involved in it
Step: 1
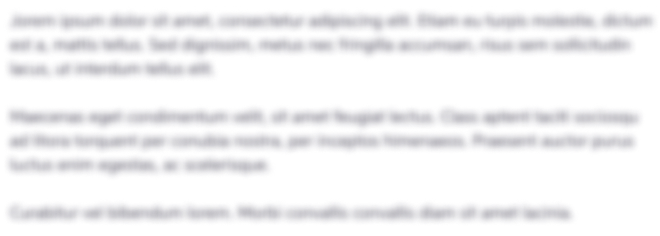
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started