Question
In this assignment, you are required to develop a simple Soccer Player Management and Visualisation System in python . The system is based on the
In this assignment, you are required to develop a simple Soccer Player Management and Visualisation System in python . The system is based on the European Soccer Database on Kaggle, which is a platform for predictive modeling and analytics competitions based on the datasets uploaded by companies and users. The soccer player database has been preprocessed and saved in the Players.txt file, which can be downloaded from here. The first line of the file contains the attribute name: "Player ID", "Play Name", "Age", "Height", and "Weight", and each following line contains a record for a player. The system allows a user to add, search and visualise the details of players.The main program should first display a menu as follows. A user needs to select an operation from the main menu.
=======================================================
Welcome to the Soccer Player Management and Visualisation System
Add details of a player.
Search student details for a player.
visualize student details.
Quit.
=======================================================
Please select an option from the above:
Once the new record has been added, the system will then ask the user 'Do you want to enter details for another player (Y/N)?' If they enter 'Y' the system will allow them to enter details for another student as before, if they enter 'N' the system will display the main menu again, otherwise it will ask the same question again.
A typical example of the display of the program (once a user chooses the option ) can be as follows. Your program MUST follow the same display style.
Please enter the player ID: 000011000 Please enter the player name: Lionel Messi Please enter the age: 30 Please enter the height: 169 Please enter the weight: 72
Thank You!
The details of the player you entered are as follows: Player ID: 000011000 Player name: Lionel Messi Age: 30 Height: 169 Weight: 72.0 The record has been successfully added to the Player.txt file.
Do you want to enter details for another plaer (Y/N)? N ================================================================= Welcome to the Soccer Player Management and Visualisation System dd details of a player. earch player details for a player. uit. ================================================================= Please select an option from the above:
After the operation, the Players.txt file will have the following content after the details of Lionel Messi are entered.
If a user chooses the option from the main menu then the program asks the user to enter the player ID for whom they want to see details. To facilitate the search option you need to use an appropriate data structure such as List. The program then collects the player details from the Players.txt file and displays it as follows (assuming the following player was searched for).
Please enter the player ID you want to search: 000011000
Thank You!
One player has been found: Player ID: 000011000 Player name: Lionel Messi Age: 30 Height: 169 Weight: 72.0
Do you want to search for another player (Y/N)? N
After displaying the player information the program prompts the user with the following message, 'Do you want to search for another player (Y/N)?' If a user enters 'Y' then the program asks them to enter the player ID for whom the information needs to be searched and displayed, else if the user enters 'N' then the program displays the main menu, otherwise the program prompts the same message again.
If a user chooses the option
1. Age 2. Height 3. Width
Please select the attribute you want to visualise: 1
For example, if the user selects "Age", the program will display the histogram of the ages of all the players. To show the histogram, you will need to use some 2D plotting libraries such as Matplotlib. Note that you need to appropriately choose the bin number to avoid producing too-sparse or too-dense histograms.
After displaying the histogram, the program prompts the user with the following message, 'Do you want to visualize for another attribute (Y/N)?' If a user enters 'Y' then the program asks them to select the attribute that needs to be visualised, else if the user enters 'N' then the program displays the main menu, otherwise the program prompts the same message again.
Finally, the program quits if the user chooses the option .
Your program should be able to handle invalid inputs such as not-a-number or negative ages, heights and weights.
Implement your algorithm in Python. Comment on your code as necessary to explain it clearly.
In addition for this exercise, use multiple functions, instead of using a single function to do everything. Create a good design of the functions to make the best use of the code and avoid duplicate calculations. For example, you can have a function for calculating the weighted mark of an assignment and the function can be used for calculating all weighted marks. Avoid duplicate code.
You also need to design your program so that it has components that can be reused in another program, if needed. Handle exceptions appropriately. Use appropriate data structure.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
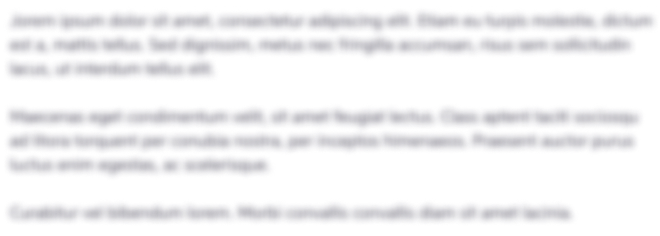
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started