In this assignment you are required to implement a CD database for a store. The store wants to keep for each artist a list of
In this assignment you are required to implement a CD database for a store. The store wants to keep for each artist a list of CDs. For each CD a CD number (int), title (char [20]), and a count (int) of the CD in stock will be stored in the database. Each CD should have a unique number. For each artist, artist name (char [20]) and a list of CDs will be stored.
Write a program that implements this database. Use Structs. To make it easy, approach the program development in various phases:
You should present the user with a menu that allows him/her to do the following operations:
1) Stock a new CD
2) Print the details of an artist
3) Search a CD
4) Sell a CD
5) Quit
When the user selects the first menu item, your program must ask the following information of the new CD:
Artist name
CD number
CD title
The amount to be stocked.
If the artists record already exists in the database, your program should add the new CD to the list of CDs of the artist in CD number order (i.e. the CDs are kept in ascending order of CD numbers and remember that CD numbers are unique).
If the artist doesnt exist in the database, you need to add the artist information and create the list with one CD.
When the user selects the second menu item, your program should ask the name of the artist and print the details of all of his/her CDs in the stock. (i.e. the number, title, count)
When the user selects the third menu item, your program should ask the CD number and print its details (i.e. artist name, title, count in stock) if it is in store. If the CD is not in store your program should print an appropriate message.
When the user selects the fourth menu item, your program should ask the CD number, search the database and if it is found, it should decrement the amount in stock by one. If this amount is zero, then that CD should be deleted from the list. An appropriate message should be printed to the screen after the purchase, or if the purchase could not be made.
When the user selects the fifth menu item, your programs ends.
After executing a menu choice, the menu should keep on popping up again until the user chooses to quit.
Design a menu interface that presents the user with the above options, and gets a response from the user. In the early stages of program development, just create a stub (i.e. a ``dummy" function) for each option. Ensure that any invalid option is catered for. Also, make sure to prompt the user for additional information required for each option (e.g. ``search a CD" will need the CDs number).
Implementation Requirements
This program is somewhat open ended so that you can interpret some things for yourself and decide how to implement them. But there are some restrictions on your design:
1) Create a CD_type_node structure having the following fields:
CD number
CD title
CD count
A pointer to CD_type_node to point to next CD.
2) Create a Artist_type_node structure having the following fields:
Artists name
A pointer to a CD_type_node, to enable you to create a linked list for CDs.
3) In your main, create an array of type Artist_type_nodes that can store up to 100 elements.
Thus, your database will be an array of 100 Artists, where each Artist has a list of CDs. Basically, each Artists name and a pointer to the list of CDs will be stored in the Artist_Array.
4) Write a function to insert a CD record into a linked list of CD_type_nodes in ascending order of CD number.
5) Write a function that will delete a CD record from a linked list of CD_type_nodes, given its CD number.
You may assume that the user correctly enters data. Thus, the user will always enter the correct type of data, all integers entered will be positive, and the user will never enter a duplicate number for a CD number. (Also, all CD titles will contain no spaces.). I am making a reference to a link list, but you can do the project also using arrays if you wish.
Write in C Write in C
Step by Step Solution
There are 3 Steps involved in it
Step: 1
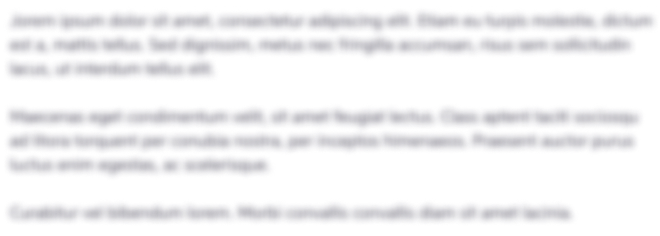
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started