Question
In this assignment, you will be creating a Java console application as a Winter Food Planner to store information about a meal made of Entrees
In this assignment, you will be creating a Java console application as a "Winter Food Planner" to store information about a meal made of Entrees and Desserts. Please post the output when finished.
Please use the below code to help you get started:
public class Main { public static void main(String[] args) { System.out.println(" " + " ^ ^ ^ ^ ^ ^ ^ ^ ^ ^ ^ ^ ^ ^ ^ ^ ^ " + " /W\\ /i\\ \\ /t\\ /e\\ /r\\ /M\\ /e\\ /a\\ /l\\ /P\\ /l\\ /a\\ \\ \\ /e\\ /r\\ " + "<___x___x___x___x___x___> <___x___x___x___> <___x___x___x___x___x___x___> " + ""); System.out.println("Enter (1) to enter an Entree"); System.out.println("Enter (2) to enter a Dessert"); System.out.println("Enter (3) to quit"); System.out.print(">> "); // Choice 1: Entree System.out.print("What's the name of the entree ? "); System.out.print("What is the prep time (minutes) ? "); System.out.print("What is the cost of ingredients $ "); System.out.print("What side dishes accompany it ? "); // Choice 2: Dessert System.out.print("What's the name of the dessert ? "); System.out.print("What is the prep time (minutes) ? "); System.out.print("What is the cost of ingredients $ "); System.out.print("Is it sugar-free (Y/N) ? "); // Choice 3: Quit System.out.println("~~~All Winter Foods Entered~~~ "); System.out.println(" Total cost of all food = "); System.out.println("Average cost of all food = "); System.out.println("Food with min prep time = "); // Any other choice is an error: System.out.println("You have entered an invalid choice. Please try again."); } }


Below is an example output:

WinterFood: This is the abstract parent (base) class of the other two. Here are the specifications: 1. [1 point] Make WinterFood an abstract class (cannot be instantiated). 2. [1 point] Create instance variables for name (String), prepTime (int) and cost (double). Be sure that all instance variables can be inherited by the two child classes** 3. [3 points] Create a constructor with 3 parameters (name, prepTime, cost) that can only be used by the children. Initialize all instance variables. 4. [1 point] Create accessors/mutators for all instance variables. Entree: This one of the concrete child (derived) classes. Here are the specifications: 1. [1 point] Create instance variable for sideDishes (String) 2. [1 point] Create accessor/mutator for sideDishes 3. [4 points] Create a constructor with 4 parameters (name, prepTime, cost, sideDishes). Initialize all instance variables. 4. [5 points] Override the toString() method that displays all fields in the following format (ensure price is formatted as currency): Entree [Name=Pozole Rojo, Sides=tostadas, rice, PrepTime =210min, Cost =$15.77 ] Dessert: This is the other concrete child (derived) class. Here are the specifications: 1. [1 point] Create instance variable for sugarFree(String). 2. [1 point] Create accessor/mutator for sugarFree 3. [4 points] Create a constructor with 4 parameters (name, prepTime, cost, sugarFree). Initialize all instance variables. 4. [5 points] Override the toString() method that displays all fields in the following format (ensure price is formatted as currency): Dessert [ Name = Sugar Free Christmas Cookies, Sugar - Free =Y, PrepTime =30min, Cost =$9.00 ] in.java: in this demo, write a public static void main(String[ ] args) method that performs the following: 1. [2 points] Maintains an array named meal of WinterFood[ ] with size 10. 2. [2 points] Prompt the user with 3 options to record a new Entree (option 1) or Dessert (option 2). Option 3 is to exit - [5 points] If the user enters option 1 (Entree), prompt for all necessary information. Create a new Entree object and add it to the array. - [5 points] Else if the user enters option 2 (Dessert), prompt for all necessary information. Create a new Dessert object and add it to the array. - [1 points] Else if the user enters option 3 (quit), do the following: [8 points] Display the total cost of all the WinterFoods in the array. Be sure to make a value-returning method to accomplish this task, with the signature: public static double totalCost(WinterFood[] meal, int count) [8 points] Display the WinterFood with the minimum prep time. Be sure to make a value-returning method to accomplish this task, with the signature: public static WinterFood foodWithMinPrepTime(WinterFood[] meal, int count) Finally, display the message "Hope we "cook" again in a future CS course soon!" and exit. - [1 point] Else, if the user enters an invalid option (not 1-3), display the error message: "You have entered an invalid choice. Please try again." Enter (1) to enter an Entree Enter (2) to enter a Dessert Enter (3) to quit 2 What's the name of the dessert ? Sugar Free Cookies What is the prep time (minutes) ? 30 What is the cost of ingredients $9.00 Is it sugar-free (Y/N) ? Y Enter (1) to enter an Entree Enter (2) to enter a Dessert Enter (3) to quit >>3 All Winter Foods Entered Entree [Name=Glazed Ham, Sides=sweet potatoes, green beans, PrepTime=135 min, Price= $ Entree [Name=Pozole Rojo, Sides=tostadas, rice, PrepTime=210 min, Price=\$15.77] Dessert [Name=Bunuelos, Sugarfree (Y/N) ? N, PrepTime=45 min, Price= $12.50 ] Dessert [Name=Sugar Free Cookies, Sugarfree (Y/N) ? Y, PrepTime=30 min, Price=\$9.00] Total cost of all food =$70.17 Average cost of all food =$17.54 Food with min prep time = Dessert [Name=Sugar Free Cookies, Sugarfree(Y/N)? Y, PrepTime=30 min, Price Hope we "cook" again in another CS course soon
Step by Step Solution
There are 3 Steps involved in it
Step: 1
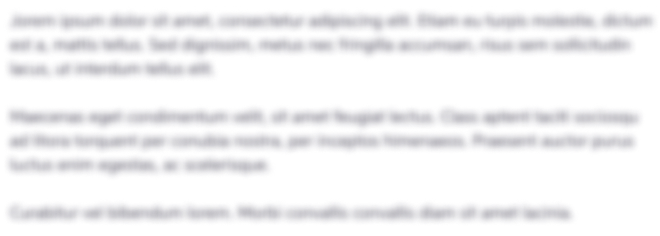
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started