Question
In this assignment, you will have to create a subroutine to display a full checker board. The checker board is a grid of 8x8 squares.
In this assignment, you will have to create a subroutine to display a full checker board. The checker board is a grid of 8x8 squares. The flow of the program should go as follows: 1. allocate space for board state 2. initialize board state to all zeros 3. Begin loop a. print board state b. ask for row c. ask for column d. ask for value e. set board (row,column) to value f. go to(3a) The input parameter to this function is the board state. The function prototype would be defined as follows: void display_board(BoardObject board) { } The BoardObject is a data type that stores the locations of all the checkers on the board and the information associated with it. This is referred to as the state of the board. When you declare your function in MIPS assembly, please pass the variable board by reference. Do not try to use the stack to pass by value In MIPS assembly, there are a limited number of color choices. We will use black for the illegal squares and white (spacebar) for the legal squares where game pieces are placed. The letter r is used for red game pieces while the letter w is used for white game pieces. The following list of characters can be used with the $print_char system call to denote pieces in individual squares ASCII 32 (spacebar): empty square. ASCII 114 (r): regular red game piece. ASCII 82 (R): red king. ASCII 119 (w): regular white game piece. ASCII 87 (W): white king. ASCII 219: an empty square of the opposite color. We will need to define the board data structure. The board requires 64 squares in an 8x8 grid. At each grid square, we have several possibilities: a) a checker can be on the square or not, b) the checker could be a king, or not, c) the checker could be either red or white. Since multidimensional arrays are not possible in MIPS32, we define the board as a single array of integers: unsigned int board[64]; Each integer represents a square on the board. Given a row and column, the location on the board can be referenced to assign a value as follows: board[row*8+column]=; You can multiply the row number row by 8 and add the column number column to get the information about the square located at (row,column). If a board location has no game piece, its value is 0. If the board value is non-zero, there is a game piece. The bits of each board location are defined as follows: E: if 1, there is a checker at this board location, 0 if no checker. C: If 1, the color is white, 0 if the color is black (red). K: If 1, the checker is a king, 0 if the checker is normal.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
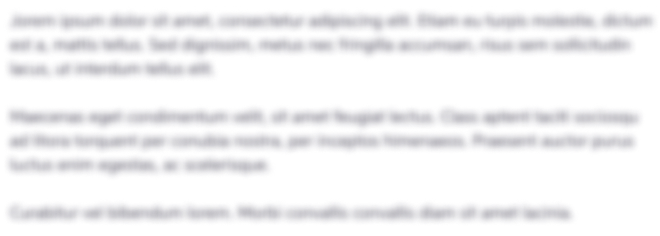
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started