Question
In this assignment you will implement a circular singly-linked list. Note that a circular linked list is a linked list where last.next = head. The
In this assignment you will implement a circular singly-linked list. Note that a circular linked list is a linked list where last.next = head. The name of your class should be CircularLinkedList. Begin your assignment by using LinkedList.java file posted online. This file defines the instance variables for the LinkedList class and the code for inner Node class. Use the file HWFive.java to test your code.
In addition to the methods defined in LinkedList.java include the following methods. public void rotate(int numPositions) If numPositions < 0 then returns an IllegalArgumentException. If numPositions > 0 then move the head numPositions to the right. (Moving to the next Node is considered moving to the right.) If numPositions is zero then the head of the list remains unchanged. Note that numPosition can be greater than size 1 The methods currently in LinkedList.java need to be modified: addFirst both add methods remove hasNext in the Iterator class
---------------------------------------Class for testing the code:---------------------------------------------------------------------
public class HWFive {
public static void main(String[] args) {
CircularLinkedList list = new
CircularLinkedList<>();
for(int i = 0; i < 5; i++) {
list.add("" + i);
}
System.out.println("First Output");
for(String s:list) {
System.out.println(s);
}
System.out.println(" Second Output");
list.add(0, "one");
for(String s:list) {
System.out.println(s);
}
System.out.println(" First element in the list: " +
list.get(0));
list.remove(0);
System.out.println(" First element in the list: " +
list.get(0));
System.out.println(" Third Output");
for(String s:list) {
System.out.println(s);
}
System.out.println();
list.addFirst("Two");
System.out.println("Two is in the list: " +
list.contains("Two"));
System.out.println("Three is in the list: " +
list.contains("Three"));
list.add(list.size(), "last");
System.out.println(" Fourth Output");
for(String s:list) {
System.out.println(s);
}
list.remove(list.size() - 1);
System.out.println(" Fifth Output");
for(String s:list) {
System.out.println(s);
}
try {
list.remove(list.size());
}
catch(IndexOutOfBoundsException e) {
System.out.println(" remove Index Out Of
Bounds");
}
try {
list.get(list.size());
}
catch(IndexOutOfBoundsException e) {
System.out.println(" get Index Out Of
Bounds");
}
list = new CircularLinkedList<>();
for(int i = 1; i <= 5; i++) {
list.add("" + i);
}
try {
list.rotate(-1);
}
catch(IllegalArgumentException e) {
System.out.println("Illegal Argument
Exception");
}
list.rotate(1);
System.out.println(" Sixth Output");
for(String s:list) {
System.out.println(s);
}
list.rotate(2);
System.out.println(" Seventh Output");
for(String s:list) {
System.out.println(s);
}
list.remove(list.size() -1);
System.out.println(" Eighth Output");
for(String s:list) {
System.out.println(s);
}
list.remove(0);
list.remove(list.size() -1);
System.out.println(" Ninth Output");
for(String s:list) {
System.out.println(s);
}
list.add(0, "4");
System.out.println(" Tenth Output");
for(String s:list) {
System.out.println(s);
}
list.add(list.size(), "2");
System.out.println(" Eleventh Output");
for(String s:list) {
System.out.println(s);
}
list.rotate(2003); // equivalent to rotate(2003%size)
System.out.println(" Twelth Output");
for(String s:list) {
System.out.println(s);
}
int size = list.size();
}
catch(IndexOutOfBoundsException e) {
System.out.println(" get Index Out Of
Bounds");
}
list = new CircularLinkedList<>();
for(int i = 1; i <= 5; i++) {
list.add("" + i);
}
try {
list.rotate(-1);
}
catch(IllegalArgumentException e) {
System.out.println("Illegal Argument
Exception");
}
list.rotate(1);
System.out.println(" Sixth Output");
for(String s:list) {
System.out.println(s);
}
list.rotate(2);
System.out.println(" Seventh Output");
for(String s:list) {
System.out.println(s);
}
list.remove(list.size() -1);
System.out.println(" Eighth Output");
for(String s:list) {
System.out.println(s);
}
list.remove(0);
list.remove(list.size() -1);
System.out.println(" Ninth Output");
for(String s:list) {
System.out.println(s);
}
list.add(0, "4");
System.out.println(" Tenth Output");
for(String s:list) {
System.out.println(s); }
list.add(list.size(), "2");
System.out.println(" Eleventh Output");
for(String s:list) {
System.out.println(s); }
list.rotate(2003); // equivalent to rotate(2003%size)
System.out.println(" Twelth Output");
for(String s:list) {
System.out.println(s);
}
int size = list.size();
for(int i = 1; i < size; i++) {
list.remove(list.size() - 1); }
list.rotate(1001);
System.out.println(" Thirteenth Output");
for(String s:list) {
System.out.println(s); }
list.remove(0);
System.out.println(" size = " + list.size()); } }
-------------------------------------------------Below is LinkedList class:----------------------------------------------------------------------------
import java.util.Iterator;
import java.util.function.Consumer;
public class LinkedList implements Iterable {
private Node head;
private int size;
private class Node {
Node next;
Item item;
}
public void forEach(Consumer c){
Node ptr = head;
for(int i = 0; i < size; i++){
c.accept(ptr.item);
ptr = ptr.next;
}
}
public Item remove(int i){
if (i < 0 || i >= size) {
throw new IndexOutOfBoundsException();
}
Item removed;
if (i == 0) {
removed = head.item;
head = head.next;
}
else {
Node ptr = head;
for (int index = 0; index < i - 1; index++) {
ptr = ptr.next;
}
removed = ptr.next.item;
ptr.next = ptr.next.next;
}
size--;
return removed;
}
public void add(int i, Item item) {
if (i < 0 || i > size) {
throw new IndexOutOfBoundsException();
}
Node tmp = new Node();
tmp.item = item;
if (i == 0) {
tmp.next = head;
head = tmp;
}
else {
Node ptr = head;
for (int index = 0; index < i - 1; index++) {
ptr = ptr.next;
}
tmp.next = ptr.next;
ptr.next = tmp;
}
size++;
}
public boolean contains(Object item) {
boolean flag = false;
Node ptr = head;
while (ptr != null && !flag) {
if (ptr.item.equals(item)) {
flag = true;
}
ptr = ptr.next;
}
return flag;
}
public Item set(int i, Item item) {
if (i < 0 || i >= size) {
throw new IndexOutOfBoundsException();
}
Node ptr = head;
for (int index = 0; index < i; index++) {
ptr = ptr.next;
}
Item old = ptr.item;
ptr.item = item;
return old;
}
public Item get(int i) {
if (i < 0 || i >= size) {
throw new IndexOutOfBoundsException();
}
Node ptr = head;
for (int index = 0; index < i; index++) {
ptr = ptr.next;
}
return ptr.item;
}
public void addFirst(Item item) {
Node temp = new Node();
temp.next = head;
temp.item = item;
head = temp;
size++;
}
public void add(Item item) { // insert item at the end of the
LinkedList
Node tmp = new Node();
tmp.item = item;
tmp.next = null;
if (head == null) // the list is empty
{
head = tmp; // place the new Node at the
front of the linked list
} else if (head != null) { // list is not empty
Node ptr = head;
while (ptr.next != null) // find the last
Node in the linked list
{
ptr = ptr.next;
}
ptr.next = tmp; // add the new Node to the
end of the linked list
} // end else
size++;
; // increase the size
}
public int size() {
return size;
}
public Iterator iterator(){
return new LinkedListIterator();
}
private class LinkedListIterator implements Iterator{
private Node ptr = head;
public boolean hasNext(){
return ptr != null;
}
public Item next(){
Item item = ptr.item;
ptr = ptr.next;
return item;
} } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
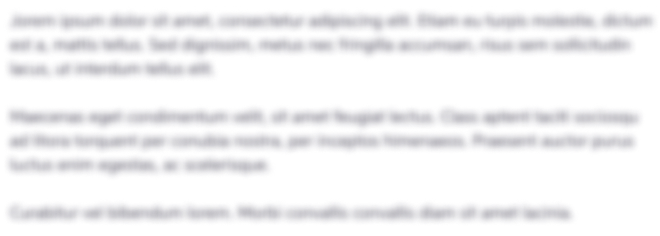
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started