Answered step by step
Verified Expert Solution
Question
1 Approved Answer
In this assignment, you will provide one possible implementation of the string class. You will use a dynamic array of characters to represent the string.
In this assignment, you will provide one possible implementation of the string class. You
will use a dynamic array of characters to represent the string. In order to avoid conflicts, we
will call the class myString and use the file names myString.h and myString.ccThere
are better ways to avoid conflicts, and we will discuss this later in the course.
Functionalities
Your class should provide the following methods. Here, current string refers to the string
that the method is called with. I.e in slength s is the current string.
default constructor: initializes to the empty string.
a constructor with a char parameter ie conversion The supplied pointer should
be treated as a character array. The string should be initialized to the content of
the character array until the character is seen. This is called a nullterminated
string or a Cstring. Note that a string literal in Ceg "hello" is treated as
a character array.
a constructor with a char parameter ie conversion A string with a single character
is constructed.
a copy constructor and an assignment operator.
a destructor.
int length: returns the length of the string.
char& atint i: returns a reference to the ith character. Also provide a version
which returns a reference to a constant.
myString substrint k int n: returns a substring of the current string starting
at index k and of length n Eg If s "abcdefg", then ssubstr "cdef".
myString& eraseint k int n: erase n characters starting at index k from the
current string. Returns a reference to the modified string. Eg If s "abcdefg",
then after serase s "abg".
myString& insertint k const myString& s: inserts the string s into the current
string at position k Returns a reference to the modified string. Eg If s
"abcdefg", then after sinsertxyz s "abxyzcdefg". Here, k can be the
length of the string, meaning that the string s is appended to the current string.
int findconst myString& s: returns the index to the first occurrence of the string
s inside the current string. If s does not occur in the current string, the function returns
myString::npos see notes below
Also, provide the following friend functions:
istream& getlineistream& is myString& s: reads a line of text into a string.
Notes
This is a large class. You may want to implement a few functionalities at a time and
test them. START EARLY!
Your assignment will be marked on program correctness and readability including
comments For all classes, use const member functions when appropriate. When
appropriate, use assert to ensure that the supplied indices are correct. You may wish
to write private helper functions for certain tasks, such as resizing the array, copying
the array, etc.
Also write a Makefile which can be used to compile all programs in this assignment.
The constant myString::npos should be a static constant member set to It is
guaranteed to be an invalid index into the string.
A driver program stringTestcc will be provided to you to test your string class.
Be sure that there are no memory errors: all arrays are large enough to hold the results,
and there are no memory leaks.
The standard way of using dynamic arrays allocating an array just large enough to
hold the result and remembering its size can be inefficient because of the frequent
need to allocate and deallocate arrays. In this assignment, we are not concerned about
efficiency.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
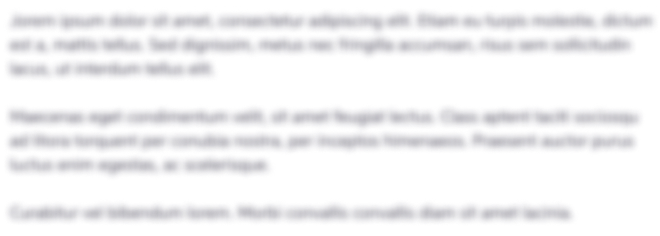
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started