Question
In this assignment, you will use AJAX to read an external JSON data file from the server. You will convert this external JSON data file
In this assignment, you will use AJAX to read an external JSON data file from the server. You will convert this external JSON data file into a JavaScript object and then add the data from each student object into new rows in an existing HTML table.
This assignment is very similar to Adding Rows to a Table assignment. The main difference is that you will:
- use jQuery instead of plain JavaScript
Set Up This Assignment
In this assignment, I'm giving you HTML, CSS, and some of the JavaScript to use. You will also use the same students.json file that you used in the week 9 assignment. Put the JSON file in a "data" subdirectory: "data/students.json"
Your JavaScript file for this assignment will be very similar to the week 9 - Adding Rows to a Table assignment.
Add this HTML to your "week10/index.html" file. Notice that we are also loading in an external JavaScript file to be able to use jQuery.
Put your name in the meta author tag (highlighted below).
jQuery: AJAX, JSON, and Table Rows jQuery: Add Rows to a Table
Introduction
In this assignment you will use AJAX to read in an external JSON data file. You will convert this external JSON data file into a JavaScript object and then display the contents in a web page as new rows in an existing table.
Student Information from JSON file
Loading student data...
Name Favorite Hobby Favorite Color
Add this CSS to your "css/styles.css" file:
body { background: transparent url('https://chelan.highline.edu/~tpollard/assets/images/tri.svg') top center no-repeat; background-size: cover; color: #555; font-family: Arial,Helvetica,sans-serif; } h1, h2, h3 { font-family: Arial, Helvetica, sans-serif; } h2 { border-bottom: 0.02em solid #3f87a6; color: #3f87a6; } .loading { background: transparent url('https://chelan.highline.edu/~tpollard/assets/images/ajax-loader_trans.gif') center left no-repeat; color: #94aace; font-size: smaller; font-style: italic; padding-left: 20px; } .loadingHidden { display: none; } main { width: 100%; min-height: 60vh; max-width: 40em; background: white; box-shadow: 0 0 2em #aaa; padding: 2em 5% 3em 5%; margin: 0 ; display: flex-container; } section { margin-bottom: 2em; } .student-info p { background: transparent url('https://chelan.highline.edu/~tpollard/assets/images/user_grayscale.png') center left no-repeat; padding-left: 20px; } table { border-collapse: collapse; min-width: 90%; } tbody tr:nth-child(odd) { background-color: #eff7fb; } td, th { text-align: left; padding-left: .3em; padding-right: .3em; } tr { border-bottom: 1px solid #ccc; } @media (min-width: 45em) { main { width: 80%; padding: 2em 5% 6em 5%; margin: 4em ; } }
jQuery uses "$( document).ready" to wait until the HTML page is loaded before any of your other JavaScript is run. Do not use "window.onload".
At the beginning of your "js/scripts.js" file you should do something like this:
// Wait until the page loads. $(document).ready(function(){ // Read JSON data and display to page. processData(); });
The processData function calls the ajaxLoadFile function. A copy of the ajaxLoadFile function to use is listed at the bottom of the assignment. You can use this function as it is. You don't need to edit it at all. Note that this time the ajaxLoadFile function is written using jQuery syntax.
We pass in 2 arguments to the ajaxLoadFile function:
- the path to the file "data/students.json", use the same json data file that you used in the week 9 assignment.
- and the callback function to run after the file was successfully read from the server.
The callback function then converts the JSON string into a JavaScript object, and then passes that object to the displayData function:
// Read the student JSON string, convert it to a JavaScript object. function processData() { ajaxLoadFile('data/students.json', function(response) { // Display the results displayData(response) ; }); }
This displayData function needs to do the same things as the week 9 assignment. Except for this time, we'll use jQuery:
- We'll walk through how to solve this assignment during class on Wednesday! Feel free to forge ahead on your own today, or you can wait until we discuss it on Wednesday.
// Display the student data. function displayData(students) { // Add the student data to the DOM. // Hide the loading message. }
Add the following JavaScript function to the end of your "js/scripts.js" file. You will use this function to read the JSON file from the server. Take some time to read through this code to understand how it works. You should be able to just use this function. You don't need to edit it at all!
Notice that the .ajax jQuery method receives all of its parameters in JavaScript object format.
/** * Description: Loads a file from the web server. Runs a callback function if sucessfull. * Parameters: filename The filename to load. * callback The callback function to run after the file has been loaded. */ function ajaxLoadFile(filename, callback) { $.ajax({url: "data/students.json", cache: false, success: function(result){ callback(result); } }); }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
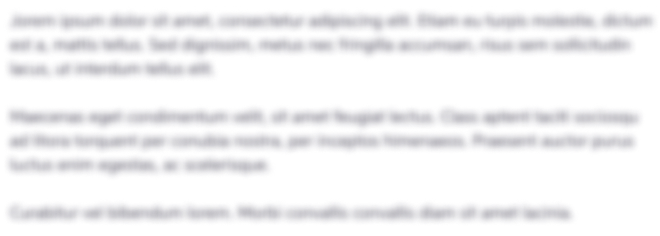
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started