Question
In this assignment, youll build on assignment 1, coding three new functions along with an expanded main. The three functions are: dynAlloc - dynamically allocates
In this assignment, youll build on assignment 1, coding three new functions along with an expanded main.
The three functions are: dynAlloc - dynamically allocates an array of integers linearSearch - searches an array sequentially looking for a target value. binarySearch - searches an array for a target value using a binary search algorithm.
In assignment 2, youll have main use dynAlloc to get space for the two arrays, one array at a time, and two new functions which both search the array for a given value. Youll also reuse one of your sort functions from assignment 1.
Youll have a main which will now look like: invoke dynAlloc to get space for the unsorted array populate the unsorted array with random integers display the unsorted array invoke dynAlloc to get space for the sorted array invoke a sort function display the sorted array get an integer from the user at the console loop while the user integer is non-negative invoke linearSearch display the result(s) invoke binarySearch display the result(s) get another integer from the user exit Function dynAlloc The dynamic allocation function has two arguments the number of elements to allocate, and a pointer to the allocated array. Use 100 as the number of elements. The pointer is defined in main and passed by reference so that when the function allocates the array, the pointer can be passed back to main.
The function uses the new operand to allocate the array. Functions linearSearch and binarySearch Each of the two search functions have four arguments: (1) a pointer to the array to search
(2) the size of the array
(3) the value to search for
(4) an integer which will contain the number of iterations it took to perform the search.
The search functions also return an integer value to the caller either the element number of the target element, or a -1 indicating that the target wasnt found. Function linearSearch is a simple linear search looping through the array sequentially, stopping when the value is found or when an entry with a greater value is found. (Remember that the search assumes that the array to search is sorted.) Function binarySearch is more complicated to code, but is faster in terms of execution. Both search algos are discussed in Gaddis chapter 8. Count iterations as follows. In the linear search, each comparison of the search value with an element of the array counts as an iteration. In the binary search, each time you chop the array into two pieces youre doing a comparison, and each of these counts as an iteration. Sorting function To sort the array, choose one of the sort functions you coded in assignment 1. Modify the code to accept pointers to the two arrays as arguments. Source code file structure Code your function declarations in a header (.hpp) file, your function definitions in a .cpp file, and your main in another .cpp file. Make sure you use a #include preprocessor directive to include the .h file in your main. Build and run all three source files together in one project.
Sample console session:
unsorted array
32 56 98 90 5 11 62 84 6 9
sorted array
1 2 3 5 6 7 8 9 11 14
Enter an integer to search for 49
invoking linear search
target found element # 55
number of iterations = 49
invoking binary search
target found element # 55
number of iterations = 5
Enter an integer to search for 82
invoking linear search
element not found
number of iterations = 57
invoking binary search
element not found
number of iterations = 6
Enter an integer to search for -1
thanks and have a nice day
Step by Step Solution
There are 3 Steps involved in it
Step: 1
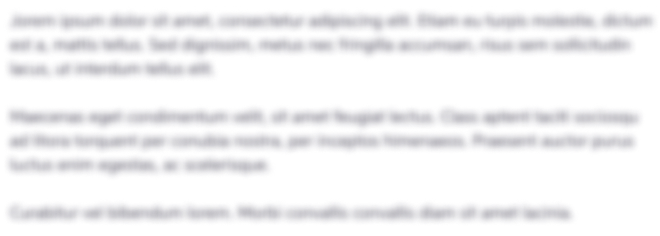
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started