Question
In this Data structures assignment, you are going to build a program that can help rental car companies to manage their rental fleet. Requirement: -this
In this Data structures assignment, you are going to build a program that can help rental car companies to manage their rental fleet. Requirement: -this should be done in c++. - you should have,arralist.cpp,arralist.h,car.cpp,car.h,cars.data files. - build ArrayList class as container. Size: 30 - build Car class to represent a car. - An ArrayList object is to hold objects from Car. ArrayList class should support following functions: - constructor - add (to append the object) - add (add at a specific index) - delete (by index) - search (an object) - traverse (i.e. print the whole list) - size (return the size of the list, not the capacity) Car class should have following fields: - id (int) - make (string) - model (string) - category (string. must be from compact, mid-size, full-size, c-suv, m-suv, f-suv, mini-van, truck) - constructor - accessors and getters. Instructions: - make up 15 cars and store them into the file: cars.data - load these cars' info from the file, initialize 15 Car objects, place them into the Arraylist object - if new cars are added by user, they should be appended to the cars.data file when exiting the program - design a menu based user interface that allows following operations: -- search ---- search by id ---- search by make ---- search by category -- add a new car (new car id cannot be same as existing ones) -- delete an existing car by id -- list all cars -- exit the program - main() should serve mainly as a dispatcher, i.e. it calls other funcitons according user's input.
Note, most parts of your arraylist should be implemented using the pseudocode below as a guide:
#include
using namespace std;
class ArrayList{
public:
typedef int data_type;
ArrayList(){ int count =0;}
void add (data_type obj){
if (count < 30)
arr[count++] = obj;
else
cout << "add failed ,no space in arraylist"<< endl;
}
void add (data_type obj,int index){
if ( count < 30 &&index <= count){
// shift remaining elements to the right
for (int i = count -1;i >= index;i--){
arr[i + 1] = arr[i];
}
arr[index]= obj;
count++;
}
else{
cout << "invalid index or arraylist is full."< } } // return -1 for not found otherwise return index int search (data_type obj){ for(int i=0;i< count;i++){ if (obj == arr[i]){ return i; } } return -1; }; data_type get (int index){ if(index >=0 && index < count){ return arr[index]; } else{ cout << "invalid index"< return -99999; } }; void del(int index){ if (0<= index && index < count){ for( int i = index; i < count-1;i++){ arr[i] = arr[i +1]; } // the boundary condition is takencare of by the count not the for loop count --; } else{ cout << "invalid index" < } }; void set(data_type obj,int index){ if (0<=index && index < count) arr[index] = obj; }; int size(){ return count; } int search (){ }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
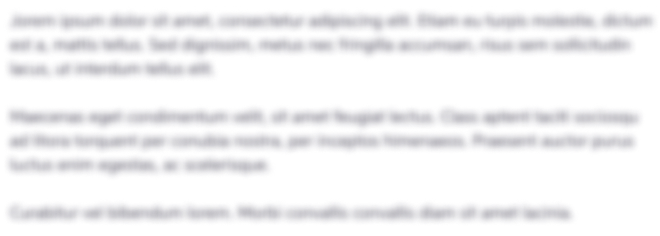
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started