Question
In this excercise, you'll create the DepartmentConstants interface presented in this chapter. In addition, you'll implement an interface named Displayable that's similar to the Printable
In this excercise, you'll create the DepartmentConstants interface presented in this chapter. In addition, you'll implement an interface named Displayable that's similar to the Printable interface.
Create the interfaces
1. Open the DisplayableTestApp class. Then, note that it includes a method named display that accepts a Displayable object as an argument.
2. Add an interface named DepartmentConstants that contains the three constants: ADMIN, EDITORIAL, and MARKETING.
Implement the interfaces
3. Open the Product class. Then, edit it so it implements the Displayable interface. To do that, add a getDisplayText method that returns a description of the product.
4. Open the Employee class. Then, edit it so it implements the DepartmentConstants and Displayable interfaces. To do that add a getDisplayText method that uses the constants in the DepartmentConstants interface to include the department name and the employee's name in the string that it returns.
Use the classes that implement the interfaces
5. Open the DisplayableTestApp class. Then, modify the variable that stores the Employee object so it is of the Displayable type.
6. Add code that passes the Displayable object to the static display method that's coded at the end of this class.
7. Run the application to make sure that it displays the employee information.
8. Repeat the previous three steps for a Product object. When you're done, the console should look like this:
Welcome to the Displayable Test application
John Smith (Editorial)
Murach's Java Programming
-------------------------------------------------------------
Use a default method
9. In the Employee and Product classes, rename the getDisplayText methods to toString methods so that they override the toString method of the object class. This should prevent the class from compiling and display an error message that indicates that the classes dont implement the getDisplayText method.
10. In the Displayable interface, modify the getDisplayText method so its a default method. The code for this method should return the String object that's returned by the toString method. This should allow the Employee and Product classes to compile since they can now use the default method.
11. Run the application to make sure it works as before.
This is the CODE:
Displayable.java
package murach.test;
public interface Displayable { String getDisplayText(); }
DisplayableTestApp.java
package murach.test;
public class DisplayableTestApp {
public static void main(String args[]) { System.out.println("Welcome to the Displayable Test application ");
Employee e = new Employee(2, "Smith", "John"); // TODO: add code that passes this object to the display method below
Product p = new Product("java", "Murach's Java Programming", 57.50); // TODO: add code that passes this object to the display method below System.out.println(); }
private static void display(Displayable d) { System.out.println(d.getDisplayText()); } }
Employee.java
package murach.test;
public class Employee {
private int department; private String firstName; private String lastName;
public Employee(int department, String lastName, String firstName) { this.department = department; this.lastName = lastName; this.firstName = firstName; } }
Product.java
package murach.test;
import java.text.NumberFormat;
public class Product {
private String code; private String description; private double price;
public Product() { this.code = ""; this.description = ""; this.price = 0; }
public Product(String code, String description, double price) { this.code = code; this.description = description; this.price = price; }
public void setCode(String code) { this.code = code; }
public String getCode() { return code; }
public void setDescription(String description) { this.description = description; }
public String getDescription() { return description; }
public void setPrice(double price) { this.price = price; }
public double getPrice() { return price; }
public String getPriceFormatted() { NumberFormat currency = NumberFormat.getCurrencyInstance(); return currency.format(price); } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
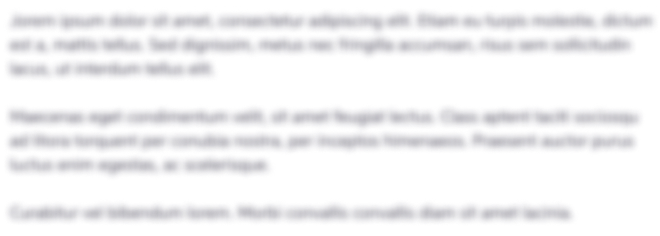
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started